Avoiding Common Pitfalls in Your Groovy Soft Introduction
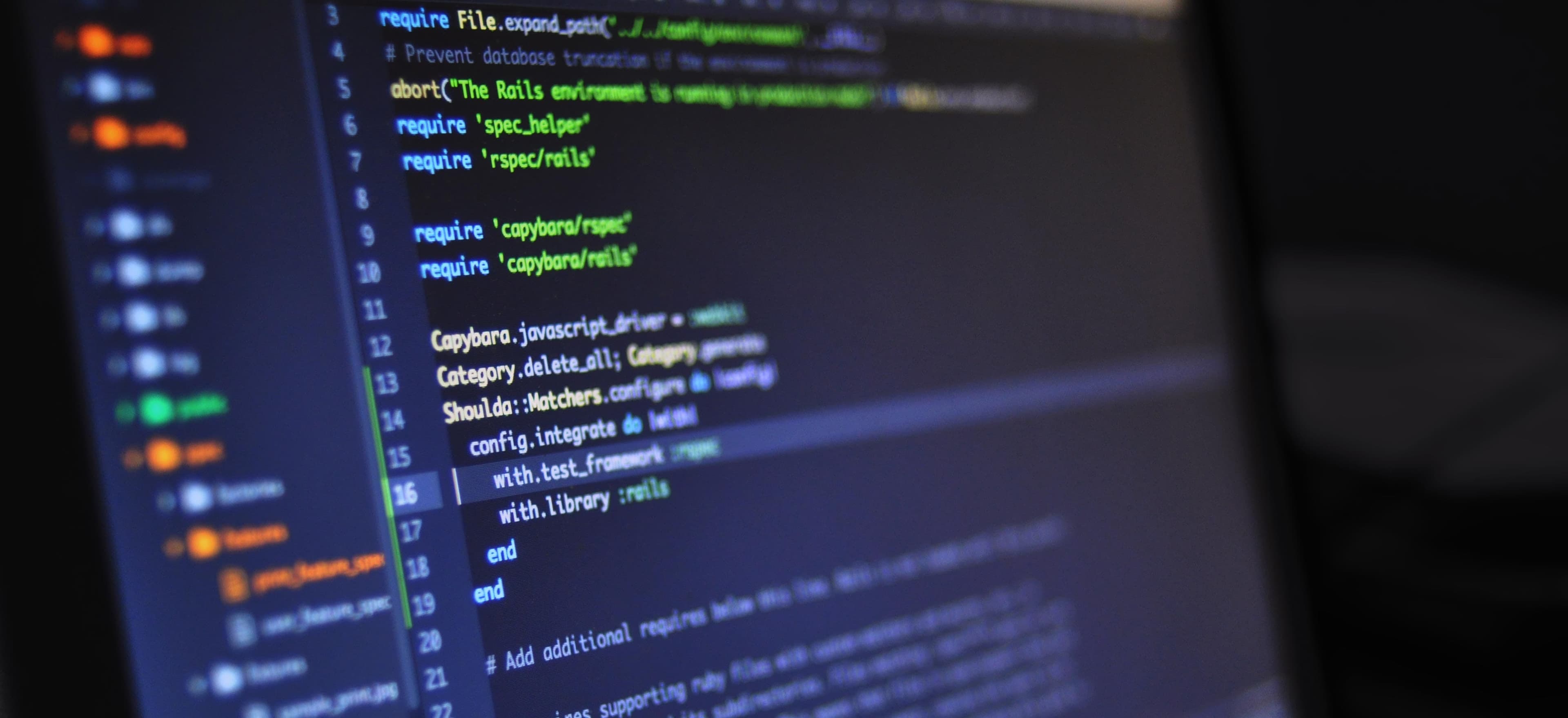
- Published on
Avoiding Common Pitfalls in Your Groovy Soft Introduction
When it comes to Java development, Groovy has marked its presence as a powerful language that complements and enhances Java projects. Its flexibility and ease of use make it an attractive choice for developers looking to improve productivity and adopt a more dynamic and expressive language. However, like any technology, Groovy has its pitfalls. In this post, we'll explore some common mistakes and pitfalls that developers might encounter when starting their journey with Groovy, and how to avoid them.
Pitfall 1: Treating Groovy as Java
Groovy isn't just Java with a different syntax. Groovy brings its own set of features, functionalities, and coding styles that are distinct from Java. It's essential to understand and embrace these differences to fully leverage the benefits of Groovy.
// Java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
// Groovy
class HelloWorld {
static void main(args) {
println "Hello, World!"
}
}
As seen in the example, Groovy allows for a more concise and expressive syntax compared to Java. Embracing this difference can lead to cleaner and more maintainable code.
Pitfall 2: Neglecting Dynamic Typing
Groovy's dynamic typing can be a double-edged sword. While it provides flexibility and agility, it can also lead to runtime errors that are not caught during compilation. It's crucial to strike a balance between leveraging dynamic typing and ensuring code reliability.
// Dynamic Typing Example
def greet(name) {
"Hello, $name"
}
println greet("Alice") // Output: Hello, Alice
println greet(123) // Output: Hello, 123
In this example, the greet
function can accept any parameter without explicit type declarations. While this flexibility can be powerful, it also requires careful consideration to prevent unintended runtime errors.
Pitfall 3: Overusing Metaprogramming
Groovy's metaprogramming capabilities can lead to code that is hard to understand and maintain. While metaprogramming can be a powerful tool, it should be used judiciously and sparingly, prioritizing clarity and maintainability.
// Metaprogramming Example
String.metaClass.repeat = { n ->
delegate * n
}
def message = "Hello"
println message.repeat(3) // Output: HelloHelloHello
In this example, we've added a repeat
method to the String
class using metaprogramming. While this can be handy, overusing such techniques can make the codebase harder to grasp for other developers.
Pitfall 4: Ignoring DSL Capabilities
Groovy's support for Domain-Specific Languages (DSLs) is a powerful feature that is often overlooked. DSLs allow you to create expressive and readable domain-specific syntax for specific tasks, improving the overall readability and maintainability of your code.
// DSL Example
def html = new MarkupBuilder()
html.body {
h1 "Groovy DSL"
p "DSLs make code readable"
}
In this example, we've created a simple DSL for generating HTML markup. Leveraging Groovy's DSL capabilities can lead to more expressive and readable code for specific domains.
The Last Word
In conclusion, Groovy is a powerful language that can greatly enhance Java development. However, to fully leverage its capabilities, developers need to be mindful of common pitfalls such as treating it as Java, neglecting dynamic typing, overusing metaprogramming, and ignoring DSL capabilities. By understanding these pitfalls and incorporating best practices, developers can harness the full potential of Groovy in their projects.
Remember, Groovy isn't just a replacement for Java; it's a companion that brings its own unique strengths to the table.
To delve deeper into Groovy and its intricacies, consider exploring Groovy's official documentation and [Groovy Style Guide](https://github.com/apache/groovy/wiki/Groovy-Style- Guide).
So, embrace Groovy, but do it on its own terms!