Common Pitfalls in Spring 3 and HornetQ 2.1 Integration
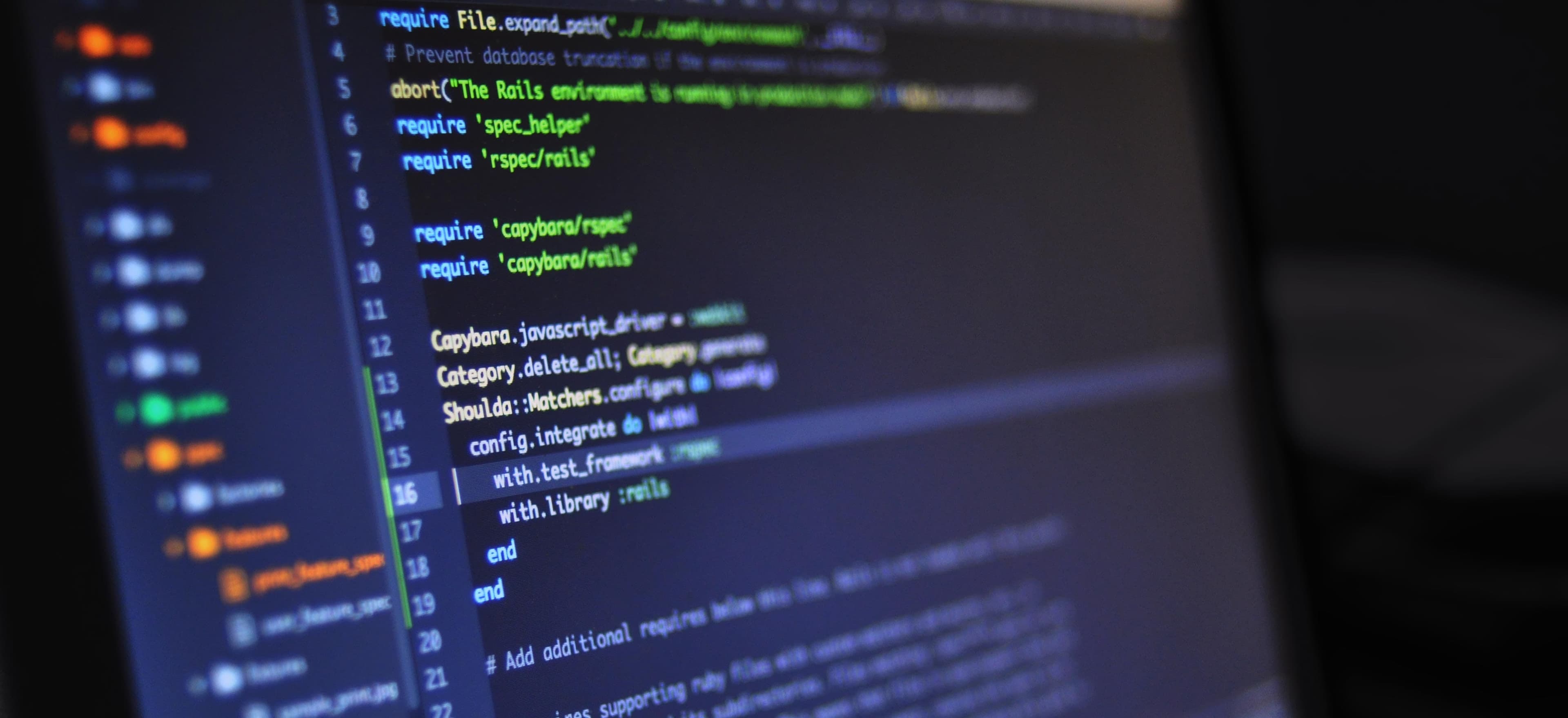
- Published on
Common Pitfalls in Spring 3 and HornetQ 2.1 Integration
Integrating Spring 3 with HornetQ 2.1 can significantly improve your Java applications' messaging capabilities. However, developers often encounter pitfalls that can derail their progress and productivity. This blog post explores the common challenges faced during this integration, providing insights and solutions to help you navigate these complexities effectively.
Why Use Spring 3 and HornetQ Together?
Spring Framework is known for its rich set of features that simplify Java application development through Dependency Injection and other design patterns. HornetQ, on the other hand, is a high-performance messaging system that supports various messaging protocols. When combined, they can create robust, scalable applications capable of reliable messaging.
However, before diving into the pitfalls, we're going to look at a basic integration setup.
Initial Setup
To get started, ensure you have the following dependencies in your pom.xml
when using Maven:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jms</artifactId>
<version>3.2.18.RELEASE</version>
</dependency>
<dependency>
<groupId>org.hornetq</groupId>
<artifactId>hornetq-jms-client</artifactId>
<version>2.1.1.Final</version>
</dependency>
</dependencies>
This setup provides the necessary libraries to facilitate the integration.
Common Pitfalls
1. Misconfiguration of JNDI
The Issue
One common issue arises from misconfigured JNDI (Java Naming and Directory Interface). JNDI is critical for looking up resources in a voting system like HornetQ.
The Solution
Ensure you have the correct resource configuration in your context.xml
file.
<Context>
<Resource name="jms/ConnectionFactory" auth="Container"
type="org.hornetq.jms.client.HornetQConnectionFactory"
maxActive="100" maxWait="10000" />
</Context>
Here, the type
should be aligned with your HornetQ connection factory class. If incorrectly specified, lookups will fail.
2. Thread Safety of Messaging Components
The Issue
Another pitfall involves the thread safety of messaging objects. HornetQ JMS components like Connection
, Session
, MessageProducer
, and MessageConsumer
are not thread-safe. Thus, sharing these objects among multiple threads can lead to runtime exceptions.
The Solution
Create dedicated instances of these objects per thread or, better yet, use a connection pool. Here’s an example of how to configure a simple ConnectionFactory
with a connection pool:
import org.hornetq.jms.client.HornetQConnectionFactory;
public class ConnectionFactoryConfig {
public HornetQConnectionFactory createConnectionFactory() {
HornetQConnectionFactory factory = new HornetQConnectionFactory();
factory.setUser("user");
factory.setPassword("password");
factory.setServerURL("tcp://localhost:5445");
return factory;
}
}
With this method, you ensure every thread has its dedicated connection, greatly reducing concurrency-related issues.
3. Ignoring Transaction Management
The Issue
Ignoring transaction management can lead to lost messages or inconsistencies, especially in distributed systems that utilize messaging. Spring provides robust transaction management that should be leveraged during integration.
The Solution
Enable transaction management in Spring with annotations. Use @Transactional
to wrap methods that send or receive messages:
import org.springframework.transaction.annotation.Transactional;
@Transactional
public void sendMessage(String message) {
jmsTemplate.convertAndSend("myQueue", message);
}
This ensures that if an error occurs during message sending, the transaction will roll back, maintaining your system's integrity.
4. Proper Exception Handling
The Issue
Many developers overlook exception handling. HornetQ produces specific exceptions related to messaging failures that need to be correctly managed to maintain application flow.
The Solution
Implement robust exception handling around messaging operations. Here's an example:
try {
sendMessage("Test Message");
} catch (JMSException e) {
// Log exception
logger.error("Failed to send message: " + e.getMessage());
// Further recovery logic can go here
}
This way, your application can recover gracefully and log pertinent information for debugging.
5. Framework Mismatch
The Issue
Spring 3 is no longer the latest version, and HornetQ has evolved with newer features in later versions like Artemis. Using older frameworks can limit functionality and introduce incompatibilities.
The Solution
Evaluate whether upgrading is feasible. Consider transitioning to Spring 5 or 6 along with HornetQ’s successor, ActiveMQ Artemis. For detailed guidance, you can refer to the official Spring Framework documentation and HornetQ documentation.
Testing Your Integration
After addressing these pitfalls, extensive testing is crucial. Spring provides tools like integration testing with @SpringBootTest
that can simulate real scenarios with HornetQ.
For example:
@SpringBootTest
public class MessagingIntegrationTest {
@Autowired
private JmsTemplate jmsTemplate;
@Test
public void testMessageSending() {
jmsTemplate.convertAndSend("testQueue", "Hello World");
// Add assertions to verify if the message is received correctly
}
}
By performing proper tests, you can ensure that your setup works efficiently and without issues.
The Bottom Line
Integrating Spring 3 with HornetQ 2.1 offers significant advantages but comes with its challenges. By being aware of these common pitfalls and implementing the suggested solutions, you can create resilient applications that fully leverage the capabilities of both frameworks.
Don’t forget to continuously monitor your application’s performance and incorporate feedback into future developments for improved stability and scalability. Happy coding!
Checkout our other articles