Implementing Multiple Inheritance with Java 8 Interfaces
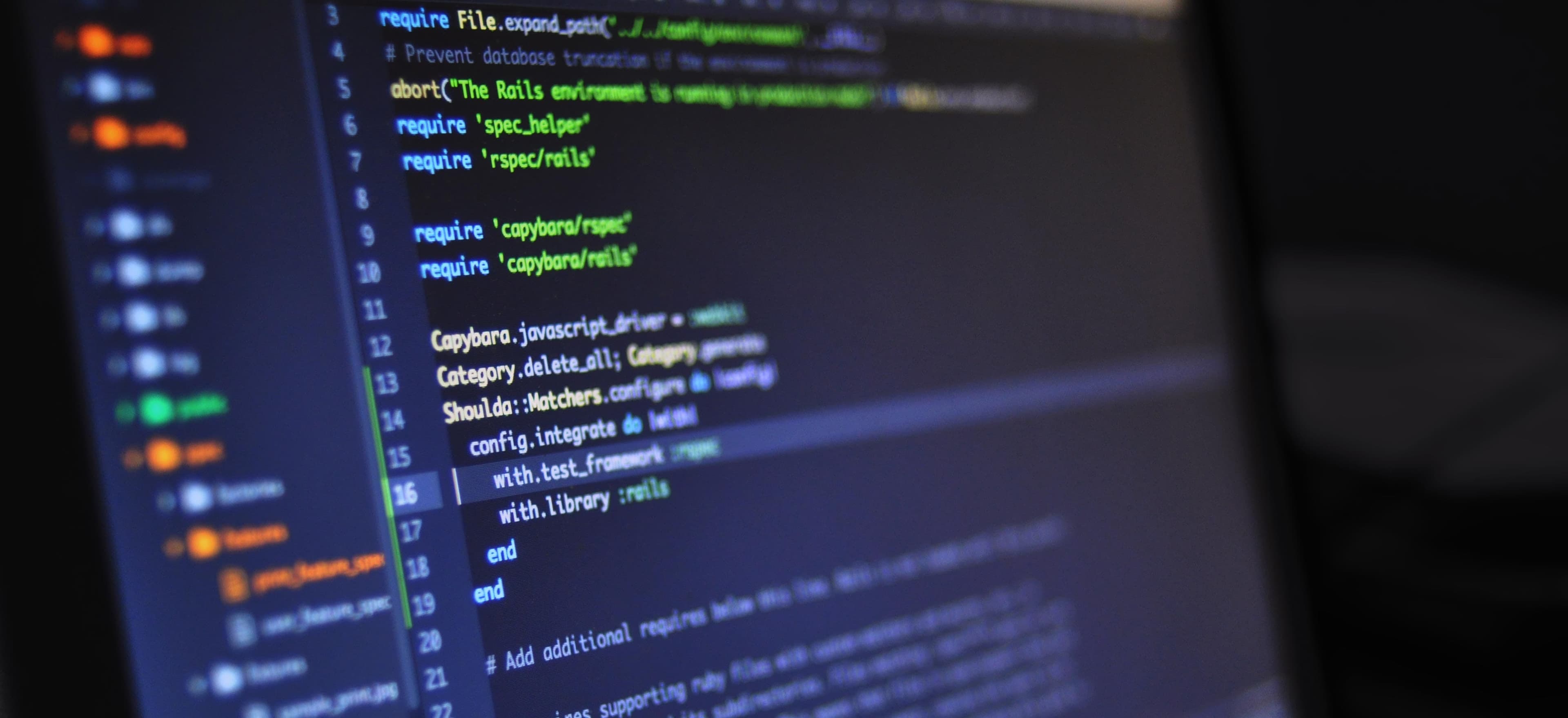
- Published on
Understanding Multiple Inheritance
Multiple inheritance is a concept in object-oriented programming where a class can inherit properties and behavior from more than one parent class. In Java, the language does not support multiple inheritance for classes, but it does allow a form of multiple inheritance through interfaces.
Java 8 introduced a major enhancement to interfaces by allowing them to have method implementations using default and static methods. This feature effectively enables interfaces to support multiple inheritance of behavior, making them more powerful than before.
In this article, we will delve into implementing multiple inheritance using Java 8 interfaces. We will explore how to define and implement interfaces with default methods, and we will discuss the best practices and potential pitfalls of using multiple inheritance in Java.
Defining Interfaces
In Java, an interface is similar to a class, but with only method signatures and constants. Prior to Java 8, interfaces could not contain method implementations, making it impossible to share code among multiple classes without resorting to abstract classes or code duplication.
With Java 8, interfaces can have method implementations using the default
and static
keywords. A default
method provides a default implementation that can be overridden by implementing classes, while a static
method is a utility method associated with the interface itself.
Let's define an example interface with default and static methods to showcase multiple inheritance:
public interface Shape {
double calculateArea();
default void printDescription() {
System.out.println("This is a shape");
}
static void printStaticInfo() {
System.out.println("Static method in Shape interface");
}
}
In this example, the Shape
interface declares calculateArea()
as an abstract method, and defines printDescription()
as a default method with a default implementation. Additionally, it contains a static
method printStaticInfo()
.
Implementing Interfaces
A class can implement multiple interfaces, thereby inheriting the default method implementations from each interface. If there are conflicts (e.g., two interfaces provide default methods with the same signature), the implementing class must override the method and provide an implementation.
Here's an example of a class implementing the Shape
interface:
public class Circle implements Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
// printDescription() is inherited from Shape interface
}
In this example, the Circle
class implements the Shape
interface and provides an implementation for the calculateArea()
method. It inherits the default implementation of printDescription()
from the Shape
interface.
Resolving Conflicts
As mentioned earlier, conflicts may arise when a class implements multiple interfaces with conflicting default method implementations. In such cases, the implementing class must explicitly override the conflicting method and provide its own implementation.
Consider the following example where a class implements two interfaces with conflicting default methods:
public interface A {
default void method() {
System.out.println("A");
}
}
public interface B {
default void method() {
System.out.println("B");
}
}
public class MyClass implements A, B {
@Override
public void method() {
A.super.method(); // Call A's default method
B.super.method(); // Call B's default method
}
}
In this example, the MyClass
class implements both interfaces A
and B
, each of which provides a default method called method()
. To resolve the conflict, the MyClass
explicitly overrides the method()
and calls the desired implementation using InterfaceName.super.method()
.
Best Practices
While multiple inheritance through interfaces can be powerful, it can also lead to complex class hierarchies and potential conflicts. When using multiple inheritance, consider the following best practices:
-
Prefer Composition over Inheritance: Instead of relying heavily on multiple inheritance, favor composition to create flexible and maintainable code.
-
Keep Interfaces Cohesive: Define interfaces with a clear and single responsibility to avoid interface bloat and potential conflicts.
-
Document Default Methods: When providing default methods in interfaces, document them clearly to guide implementing classes on how to handle conflicts or when to override.
The Bottom Line
In Java 8, interfaces gained the ability to provide method implementations using default and static methods, effectively enabling a form of multiple inheritance. By implementing multiple interfaces, a class can inherit default method implementations from each interface, with rules for resolving conflicts when they arise.
It's important to use this feature judiciously and follow best practices to avoid potential conflicts and maintain code clarity. By understanding how to define interfaces with default methods and how to implement them in classes, you can leverage multiple inheritance in Java effectively.
In conclusion, multiple inheritance through interfaces enhances the flexibility and reusability of code in Java, but it should be used thoughtfully to prevent complexity and ambiguity in the class hierarchy.
Remember, with great power comes great responsibility!
To further explore the concept of default methods in interfaces, you can visit Oracle's official documentation on default methods.
If you're interested in diving deeper into the best practices for using interfaces, you can check out this insightful article on Interface Design Best Practices.