Streamline Your Code with Java 9 Optional Enhancements
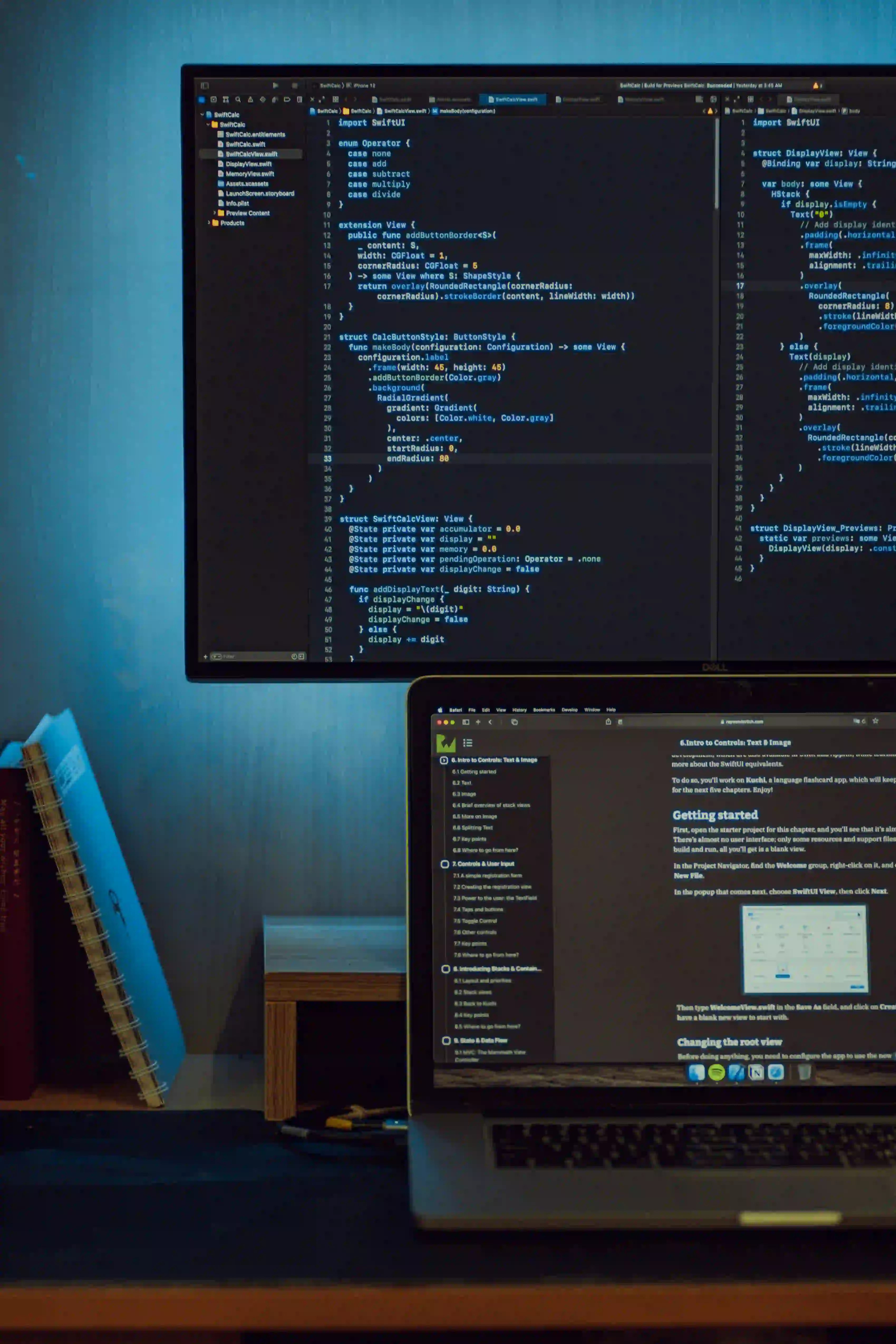
Streamline Your Code with Java 9 Optional Enhancements
Optional is a well-known class introduced in Java 8 to handle NullPointerExceptions more gracefully. In Java 9, the Optional class received several enhancements to further streamline and improve its usability.
What is Optional?
Optional is a container object that may or may not contain a non-null value. It was introduced to address the issue of null references, which often lead to NullPointerExceptions. By using Optional, developers can explicitly state whether a value is present or not, thereby reducing the likelihood of null pointer exceptions.
Java 9 Optional Enhancements
ifPresentOrElse
Java 9 introduced the ifPresentOrElse
method to the Optional class. This method allows developers to perform a certain action if a value is present and another action if the value is not present, eliminating the need for cumbersome isPresent()
checks.
Optional<String> optionalName = Optional.of("John");
optionalName.ifPresentOrElse(
name -> System.out.println("Hello, " + name),
() -> System.out.println("Hello, Guest")
);
In the above example, if optionalName
contains a value, it will be printed as "Hello, John". If optionalName
is empty, it will print "Hello, Guest".
or
Java 9 also added the or
method to the Optional class. This method returns the value contained in the Optional if present, otherwise it returns the specified fallback value.
Optional<String> optionalName = Optional.empty();
String name = optionalName.or(() -> Optional.of("Guest")).get();
System.out.println("Hello, " + name);
In this snippet, since optionalName
is empty, it will default to "Guest" and print "Hello, Guest".
stream
Another enhancement in Java 9 is the stream
method, which returns a stream containing the value if it is present, otherwise an empty stream.
Optional<String> optionalName = Optional.of("John");
optionalName.stream().forEach(name -> System.out.println("Hello, " + name));
The stream
method allows for easy integration with the stream API and functional-style operations.
ifPresentOrElse Use Case
Let's consider a practical use case for the ifPresentOrElse
method. Suppose we have a method that retrieves a user's name from a database. If the name is present, we want to perform some action, and if it's absent, we want to log the absence.
Optional<String> userName = getUserFromDatabase();
userName.ifPresentOrElse(
name -> performAction(name),
() -> logger.log("User name not found")
);
With the ifPresentOrElse
method, we can clearly define the actions to be taken based on the presence or absence of the user's name, improving the clarity and readability of our code.
In Conclusion, Here is What Matters
The enhancements made to the Optional class in Java 9 have provided developers with more streamlined and expressive ways to handle optional values. The ifPresentOrElse
, or
, and stream
methods have simplified common use cases and improved the overall usability of the Optional class.
By leveraging these enhancements, developers can write cleaner, more concise code while reducing the risk of null pointer exceptions.
In conclusion, Java 9's Optional enhancements offer a powerful tool for managing optional values, contributing to the creation of more robust and readable code. Embracing these enhancements can lead to more reliable and maintainable Java applications.
For further reading on Optional enhancements and other Java 9 features, check out the Java 9 official documentation and explore how these updates can benefit your projects.