Easing the Migration from Commons CLI to Picocli
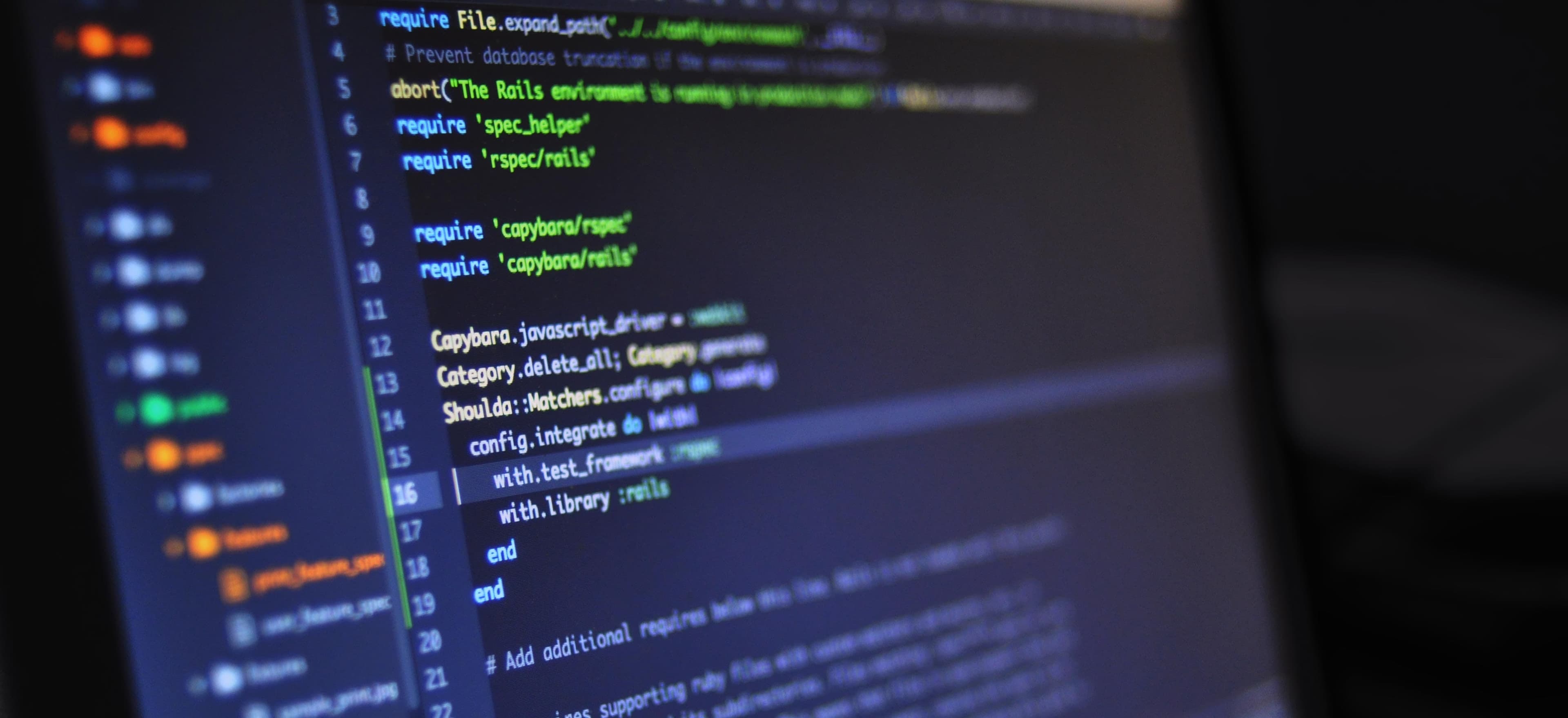
- Published on
Easing the Migration from Commons CLI to Picocli
Migrating from one library to another in a Java project can be a daunting task, especially when dealing with command-line parsing. In this post, we will delve into the process of migrating from Apache Commons CLI to the newer and more feature-rich Picocli library.
Why Migrate to Picocli?
Apache Commons CLI has been a popular choice for parsing command-line arguments in Java applications. However, the library lacks some advanced features that are increasingly necessary in modern applications. Picocli, on the other hand, provides a more extensive feature set, including support for subcommands, strong typing, and comprehensive error messages.
Understanding the Differences
Before diving into the migration process, it’s essential to understand the key differences between Apache Commons CLI and Picocli.
Support for Annotations
One of the most significant differences is Picocli's support for annotations. With Apache Commons CLI, you need to define options and arguments programmatically. Picocli, on the other hand, allows you to use annotations to define command-line options and arguments directly in your Java code.
Subcommands
Picocli also offers built-in support for subcommands, making it easier to create complex command-line interfaces. This is a feature that is not available in Apache Commons CLI.
Strongly Typed Arguments
With Picocli, you can define strongly-typed arguments, which simplifies the parsing and validation process. This feature is not as straightforward in Apache Commons CLI.
Comprehensive Error Messages
Picocli provides detailed error messages, which are especially helpful for users who are unfamiliar with the command-line interface. Apache Commons CLI may provide less informative error messages, making it harder for users to understand and correct their inputs.
Migration Steps
Now that we understand the differences between the two libraries, let’s discuss the steps involved in migrating from Apache Commons CLI to Picocli.
Step 1: Add Picocli Dependency
The first step is to add the Picocli dependency to your project. You can do this by including the following Maven dependency in your pom.xml
file:
<dependency>
<groupId>info.picocli</groupId>
<artifactId>picocli</artifactId>
<version>4.6.1</version>
</dependency>
Step 2: Annotate Command Definition
In Apache Commons CLI, you would typically define options and arguments using code. With Picocli, you can use annotations to define your command-line interface.
import picocli.CommandLine;
import picocli.CommandLine.Command;
import picocli.CommandLine.Option;
import picocli.CommandLine.Parameters;
@Command(name = "myapp", description = "This is my awesome app")
public class MyApp implements Runnable {
@Option(names = {"-h", "--help"}, description = "Prints usage help")
private boolean helpRequested;
@Parameters(description = "The input file")
private File inputFile;
// Other command logic
}
Step 3: Parse Command Line
With Apache Commons CLI, you would use a CommandLineParser
to parse the command-line arguments. In Picocli, you can simply call the CommandLine.execute(String...)
method to parse and execute your command.
public static void main(String[] args) {
CommandLine commandLine = new CommandLine(new MyApp());
commandLine.execute(args);
}
Step 4: Handle Subcommands
If your application uses subcommands, Picocli provides built-in support for defining and handling them. This is a feature not available in Apache Commons CLI.
@Command(name = "subcommand", description = "This is a subcommand")
public class SubCommand implements Runnable {
// Subcommand definition
}
By following these steps, you can effectively migrate your command-line parsing from Apache Commons CLI to Picocli. The advanced features and improved usability of Picocli make it a compelling choice for modern Java applications.
A Final Look
Migrating from Apache Commons CLI to Picocli may seem like a daunting task at first, but with the right understanding and steps, it can be a smooth transition. Picocli’s extensive feature set, annotated command definitions, and support for subcommands make it a worthy successor to Apache Commons CLI. By embracing Picocli, you can elevate the command-line interface of your Java applications to a whole new level of usability and maintainability.
In conclusion, if you are still using Apache Commons CLI for your command-line parsing needs, it's time to consider migrating to Picocli for a more robust and feature-rich solution.
For further insights on the migration process and the potential benefits of Picocli, check out the official Picocli documentation.
Now that you've completed the migration from Apache Commons CLI to Picocli, your Java applications are well-equipped with advanced features for parsing command-line arguments. Embracing Picocli opens the doors to creating more powerful and user-friendly command-line interfaces.