Optimizing Code Reusability with Prototype Pattern
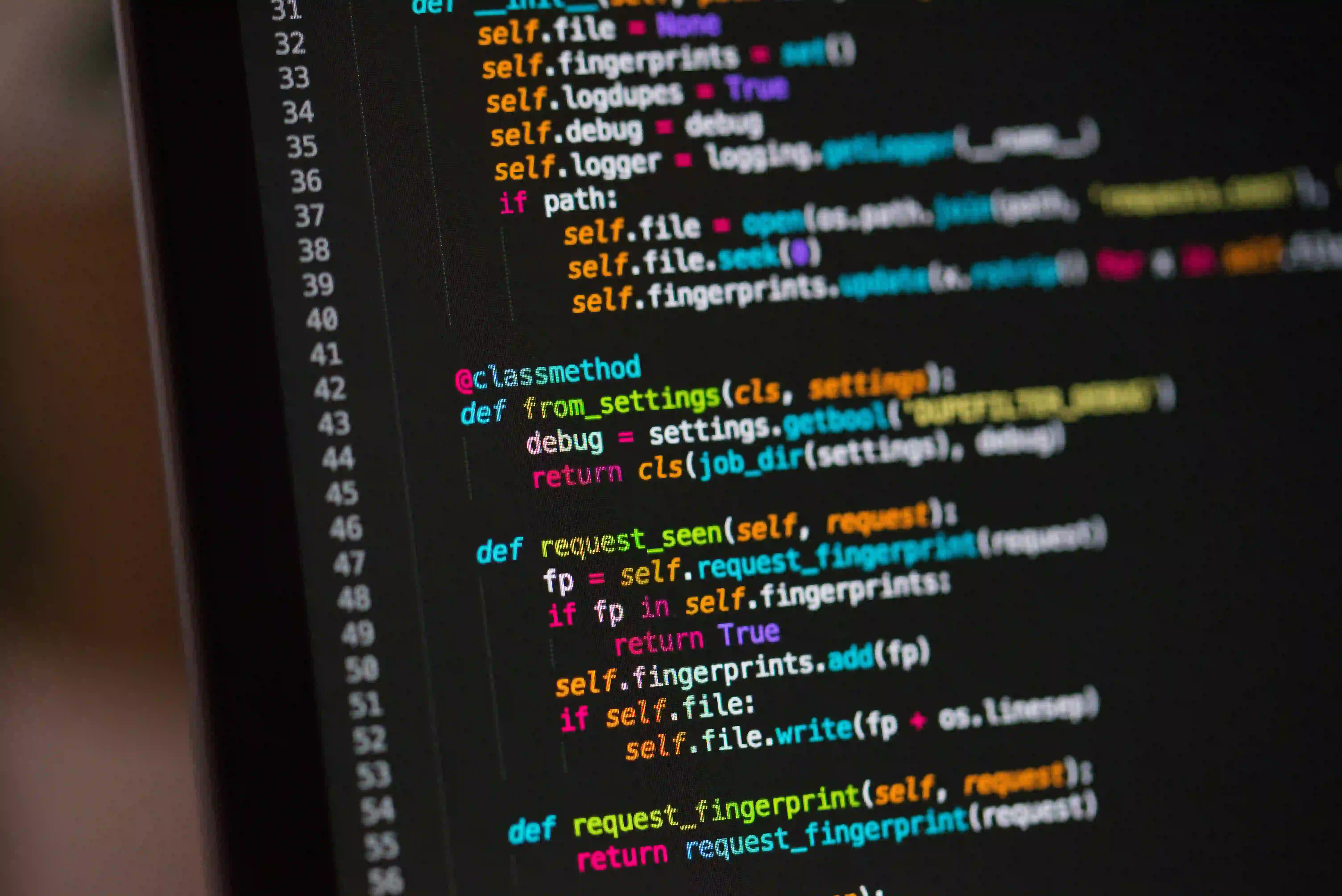
Optimizing Code Reusability with Prototype Pattern
In software development, reusability is a critical aspect of writing efficient, maintainable, and scalable code. Among the various design patterns available, the Prototype Pattern stands out as a powerful tool for creating new objects by copying an existing object, known as the prototype. This pattern not only enhances code reusability but also provides a flexible solution for object creation in complex systems. In this article, we will explore the Prototype Pattern, its implementation in Java, and its benefits in optimizing code reusability.
Understanding the Prototype Pattern
The Prototype Pattern is categorized under the creational design patterns and is used for creating objects. Instead of instantiating new objects directly, the pattern utilizes cloning to produce new instances. This approach is beneficial when the cost of creating a new object is high or when the creation process involves complex steps.
The key idea behind the Prototype Pattern is to create new objects by copying an existing object, which serves as a prototype. This prototype is used as a blueprint for creating new objects with predefined initial state and behavior. By using cloning, the pattern avoids the necessity of subclassing and the associated overhead.
Implementation in Java
Using Object.clone()
In Java, the Prototype Pattern can be implemented using the clone()
method, which is defined in the java.lang.Object
class. To utilize the clone()
method for cloning objects, the Cloneable
interface must be implemented to indicate that the objects of the class can be cloned. Here's a simple example demonstrating the implementation of the Prototype Pattern using clone()
:
public abstract class Shape implements Cloneable {
private String type;
abstract void draw();
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public Shape clone() {
Shape clone = null;
try {
clone = (Shape) super.clone();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
return clone;
}
}
public class Circle extends Shape {
public Circle() {
setType("Circle");
}
@Override
void draw() {
System.out.println("Inside Circle::draw() method.");
}
}
public class Rectangle extends Shape {
public Rectangle() {
setType("Rectangle");
}
@Override
void draw() {
System.out.println("Inside Rectangle::draw() method.");
}
}
public class PrototypeDemo {
public static void main(String[] args) {
ShapeCache.loadCache();
Shape clonedCircle = ShapeCache.getShape("1");
System.out.println("Shape : " + clonedCircle.getType());
Shape clonedRectangle = ShapeCache.getShape("2");
System.out.println("Shape : " + clonedRectangle.getType());
}
}
public class ShapeCache {
private static Map<String, Shape> shapeMap = new HashMap<>();
public static Shape getShape(String shapeId) {
return shapeMap.get(shapeId).clone();
}
public static void loadCache() {
Circle circle = new Circle();
circle.setId("1");
shapeMap.put(circle.getId(), circle);
Rectangle rectangle = new Rectangle();
rectangle.setId("2");
shapeMap.put(rectangle.getId(), rectangle);
}
}
In the above example, the Shape
class serves as the prototype, and the Circle
and Rectangle
classes extend the Shape
class while implementing the draw()
method. The ShapeCache
class maintains a map of shape prototypes and provides a method to retrieve cloned instances of shapes.
Using Java's Clonable Interface
Another method to implement the Prototype Pattern in Java is by using the java.lang.Cloneable
interface. This approach follows a similar concept but with the primary focus on implementing the clone()
method.
Benefits of Prototype Pattern
The Prototype Pattern offers several benefits, which make it a valuable tool for optimizing code reusability:
-
Minimizes Subclassing: With the Prototype Pattern, new objects are created by copying the existing ones, eliminating the need for creating subclasses to initialize new objects.
-
Reduces Cost of Object Creation: In scenarios where creating new objects is a costly process, the Prototype Pattern saves resources by cloning existing objects.
-
Enhances Flexibility: The pattern provides a flexible way of creating new objects with different configurations based on the existing prototypes, promoting easy customization.
-
Simplifies Object Creation: By encapsulating the cloning process within the prototype, the pattern simplifies the object creation process, especially for complex objects.
Key Takeaways
The Prototype Pattern is a powerful design pattern that empowers developers to optimize code reusability by leveraging object cloning. In Java, the pattern can be effectively implemented using the clone()
method or by utilizing the Cloneable
interface. By reducing the overhead of subclassing and providing a flexible approach to object creation, the Prototype Pattern significantly enhances the maintainability and scalability of codebases.
By incorporating the Prototype Pattern in your design and development workflows, you can streamline the creation of new objects, minimize the cost of object creation, and pave the way for a more efficient and reusable codebase.
In conclusion, the Prototype Pattern stands as a valuable asset in the arsenal of design patterns, offering an elegant solution to the challenge of optimizing code reusability in Java and other object-oriented programming languages.
To dive deeper into design patterns in Java, take a look at this comprehensive guide.