Handling Multiple Resource Transactions in Spring JTA with Atomikos
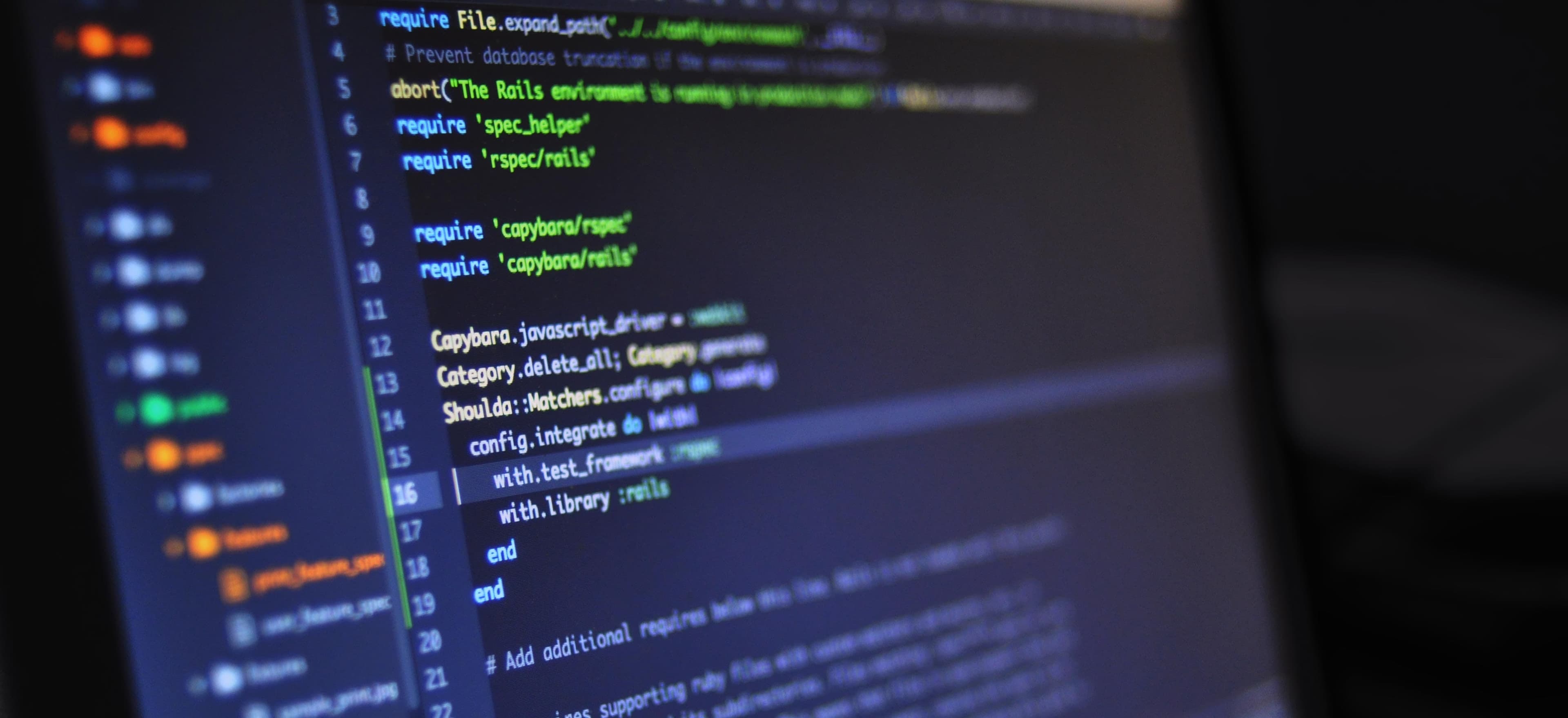
- Published on
Handling Multiple Resource Transactions in Spring JTA with Atomikos
In the world of enterprise applications, managing transactions across multiple resources is a common requirement. Especially in a microservices architecture, it's important to ensure that transactions are coordinated across several services and databases. In Java applications, the Java Transaction API (JTA) provides a standard way to manage distributed transactions. When using the Spring Framework, integrating with JTA for managing transactions becomes crucial for building resilient and consistent applications.
In this blog post, we'll explore how to handle multiple resource transactions in a Spring application using JTA with Atomikos, a popular open-source implementation of JTA. We'll go through a step-by-step guide and provide code examples to demonstrate how to configure and use Atomikos with Spring for managing distributed transactions.
Setting up the Project
First, let's create a new Spring Boot project using Spring Initializr. Make sure to include the dependencies for "Spring Web" and "Spring Data JPA" if you plan to work with databases. Additionally, we'll need the "Atomikos Transactions Essentials" dependency for integrating Atomikos with Spring.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.atomikos</groupId>
<artifactId>transactions-essentials</artifactId>
<version>4.0.6</version>
</dependency>
Configuring Atomikos Transaction Manager
To configure Atomikos as the transaction manager, we'll define a bean of type UserTransactionManager
and JtaTransactionManager
in our Spring configuration.
@Configuration
public class TransactionConfig {
@Bean
public UserTransactionManager userTransactionManager() {
UserTransactionManager manager = new UserTransactionManager();
manager.setForceShutdown(false);
return manager;
}
@Bean
public UserTransaction userTransaction() throws SystemException {
UserTransactionImp userTransactionImp = new UserTransactionImp();
userTransactionImp.setTransactionTimeout(300);
return userTransactionImp;
}
@Bean
public JtaTransactionManager jtaTransactionManager() throws SystemException {
JtaTransactionManager jtaTransactionManager = new JtaTransactionManager();
jtaTransactionManager.setTransactionManager(userTransactionManager());
jtaTransactionManager.setUserTransaction(userTransaction());
jtaTransactionManager.setAllowCustomIsolationLevels(true);
return jtaTransactionManager;
}
}
In this configuration, we create instances of UserTransactionManager
and UserTransaction
provided by Atomikos. We then use these instances to configure the JtaTransactionManager
, which will be used by Spring to manage transactions across multiple resources.
Using JTA Transactions in Spring Services
With Atomikos and JTA configured, we can start using distributed transactions within our Spring services. Let's consider an example of a service that needs to perform operations across multiple data sources in a transactional manner.
@Service
@Transactional(transactionManager = "jtaTransactionManager")
public class OrderService {
@Autowired
private OrderRepository orderRepository;
@Autowired
private PaymentService paymentService;
public void placeOrder(Order order, Payment payment) {
orderRepository.save(order);
paymentService.processPayment(payment);
}
}
In this example, the OrderService
is annotated with @Transactional(transactionManager = "jtaTransactionManager")
to indicate that its methods should be executed within a JTA-managed transaction. The OrderService
interacts with an OrderRepository
for database operations and a PaymentService
for payment processing. Both the database and payment processing are handled within the same transaction, ensuring consistency across these resources.
Dealing with Distributed Transactions
When working with distributed transactions, it's important to consider scenarios where failures might occur. With JTA and Atomikos, we can handle distributed transaction failures gracefully. For example, let's consider a scenario where an exception occurs during payment processing in the PaymentService
.
@Service
public class PaymentService {
@Transactional(transactionManager = "jtaTransactionManager")
public void processPayment(Payment payment) {
// process payment logic
if (paymentFailed) {
throw new PaymentProcessingException("Payment processing failed");
}
}
}
In this case, when the payment processing fails and an exception is thrown, the entire distributed transaction (including the database operation in OrderService
) will be rolled back automatically. This ensures that all resources involved in the transaction are left in a consistent state, even in the event of failures.
Testing Distributed Transactions with JUnit
Writing tests for distributed transactions is crucial to ensure that the transactional behavior is working as expected. When using JTA and Atomikos with Spring, we can write integration tests to validate the behavior of distributed transactions.
@RunWith(SpringRunner.class)
@SpringBootTest
@Transactional
public class OrderServiceIntegrationTest {
@Autowired
private OrderService orderService;
@Autowired
private PaymentService paymentService;
@Test
public void testPlaceOrderWithSuccessfulPayment() {
// perform order placement and payment
orderService.placeOrder(order, payment);
// assert the state of data sources after the transaction
}
@Test
public void testPlaceOrderWithFailedPayment() {
// perform order placement with a payment that is designed to fail
try {
orderService.placeOrder(order, failedPayment);
} catch (PaymentProcessingException e) {
// assert that the entire transaction was rolled back
}
}
}
In these integration tests, we can verify that the distributed transactions are behaving as expected. The first test validates a successful transaction, while the second test ensures that a failed payment results in a rolled-back transaction.
Wrapping Up
In this blog post, we've explored how to handle multiple resource transactions in a Spring application using JTA with Atomikos. By configuring Atomikos as the transaction manager and leveraging JTA, we can achieve coordination and consistency across distributed transactions. We've also seen how to use JTA-managed transactions in Spring services, handle distributed transaction failures, and write integration tests for validating transactional behavior.
Integrating JTA and Atomikos with Spring provides a robust solution for managing distributed transactions in enterprise applications. With the ability to coordinate transactions across multiple resources, Spring applications can maintain data consistency and reliability, even in complex microservices architectures.
When building enterprise applications that require transactional consistency across distributed resources, incorporating JTA with Atomikos in Spring can be a powerful and essential component of the overall architecture. With the guidance provided in this blog post, you have a solid foundation for leveraging JTA and Atomikos to manage multiple resource transactions in your Spring applications.
Checkout our other articles