Common Mistakes in Linear Regression Analysis
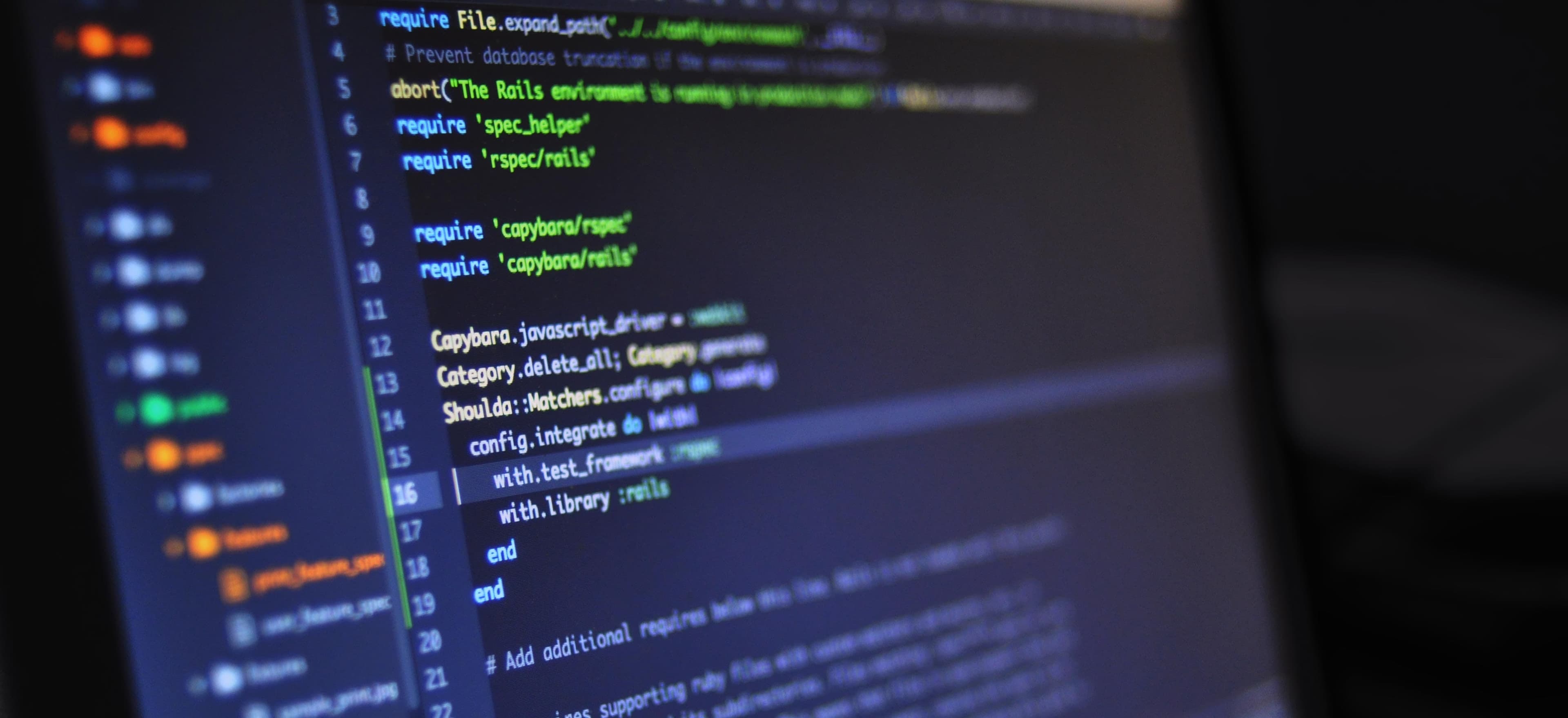
- Published on
Common Mistakes in Linear Regression Analysis
Linear regression is one of the most fundamental and widely used statistical techniques in data analysis and machine learning. However, even experienced statisticians and data scientists can make mistakes in their linear regression analysis, leading to erroneous results and conclusions. In this blog post, we'll discuss some common mistakes that are made in linear regression analysis and how to avoid them.
1. Ignoring the Assumptions of Linear Regression
Linear regression makes several key assumptions about the data, including linearity, independence, homoscedasticity, and normality of residuals. Ignoring these assumptions can lead to biased and inefficient estimates. Before running a linear regression model, it's crucial to check whether these assumptions hold for the data at hand.
// Checking for linearity using scatter plots
import org.jfree.data.xy.DefaultXYDataset;
DefaultXYDataset dataset = new DefaultXYDataset();
// Add data points to the dataset
// Create scatter plot
2. Multicollinearity
Multicollinearity occurs when independent variables in a regression model are highly correlated with each other. This can lead to inflated standard errors and unreliable estimates of the regression coefficients. Before fitting a linear regression model, it's important to check for multicollinearity among the independent variables and consider addressing it through techniques such as variable selection or principal component analysis.
// Calculate the correlation matrix
import org.apache.commons.math3.stat.correlation.PearsonsCorrelation;
double[][] data = {{/* Your data points */}};
PearsonsCorrelation correlation = new PearsonsCorrelation(data);
RealMatrix correlationMatrix = correlation.getCorrelationMatrix();
// Check for high correlations
3. Overfitting the Model
Adding too many independent variables to a linear regression model can lead to overfitting, where the model fits the noise in the data rather than the underlying relationship. This can result in poor predictive performance on new data. It's important to strike a balance between model complexity and predictive accuracy by using techniques such as cross-validation and regularization.
// Implementing cross-validation
import org.apache.commons.math3.stat.regression.OLSMultipleLinearRegression;
OLSMultipleLinearRegression regression = new OLSMultipleLinearRegression();
// Split data into training and testing sets
// Fit the model on the training data
// Evaluate the model on the testing data
4. Not Standardizing or Normalizing Variables
Failure to standardize or normalize the independent variables in a linear regression model can lead to biased estimates, especially when the variables are measured on different scales. Standardizing or normalizing the variables ensures that each variable contributes equally to the regression model.
// Standardizing variables
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
double[] data = {/* Your data points */};
DescriptiveStatistics stats = new DescriptiveStatistics(data);
// Calculate the mean and standard deviation
// Standardize the variable
5. Assuming Causation from Correlation
It's important to remember that correlation does not imply causation. Just because two variables are correlated in a linear regression model, it doesn't mean that one causes the other. Causal relationships require additional evidence from experimental or quasi-experimental studies.
6. Not Checking for Heteroscedasticity
Heteroscedasticity occurs when the variance of the residuals in a linear regression model is not constant across all levels of the independent variables. This violates the assumption of homoscedasticity and can lead to inefficient and biased estimates. It's crucial to check for heteroscedasticity and consider using robust standard errors or transforming the dependent variable if it is present.
7. Failing to Consider Interaction Effects
Ignoring interaction effects between independent variables can lead to misspecified models and biased estimates. It's important to consider potential interactions between the independent variables and include them in the model if they are theorized or observed in the data.
// Adding interaction terms to the model
regression.addInteractionTerm(/* Your interaction term */);
Final Considerations
While linear regression is a powerful and versatile tool, it's essential to be mindful of the potential pitfalls and mistakes that can arise in its application. By understanding these common mistakes and how to avoid them, you can ensure that your linear regression analysis yields accurate and reliable results. Always remember to thoroughly assess the assumptions, data quality, and model specifications before drawing conclusions from a linear regression analysis.
By being aware of these pitfalls, you can aim for more accurate and meaningful linear regression results. Don't forget to double-check assumptions, explore variable relationships, and always keep an eye out for potential mistakes in your analysis. Mastering linear regression takes time and practice, so be sure to give yourself the space to learn from any mistakes you make along the way.