Testing Grails 3.3 with Spock 1.1
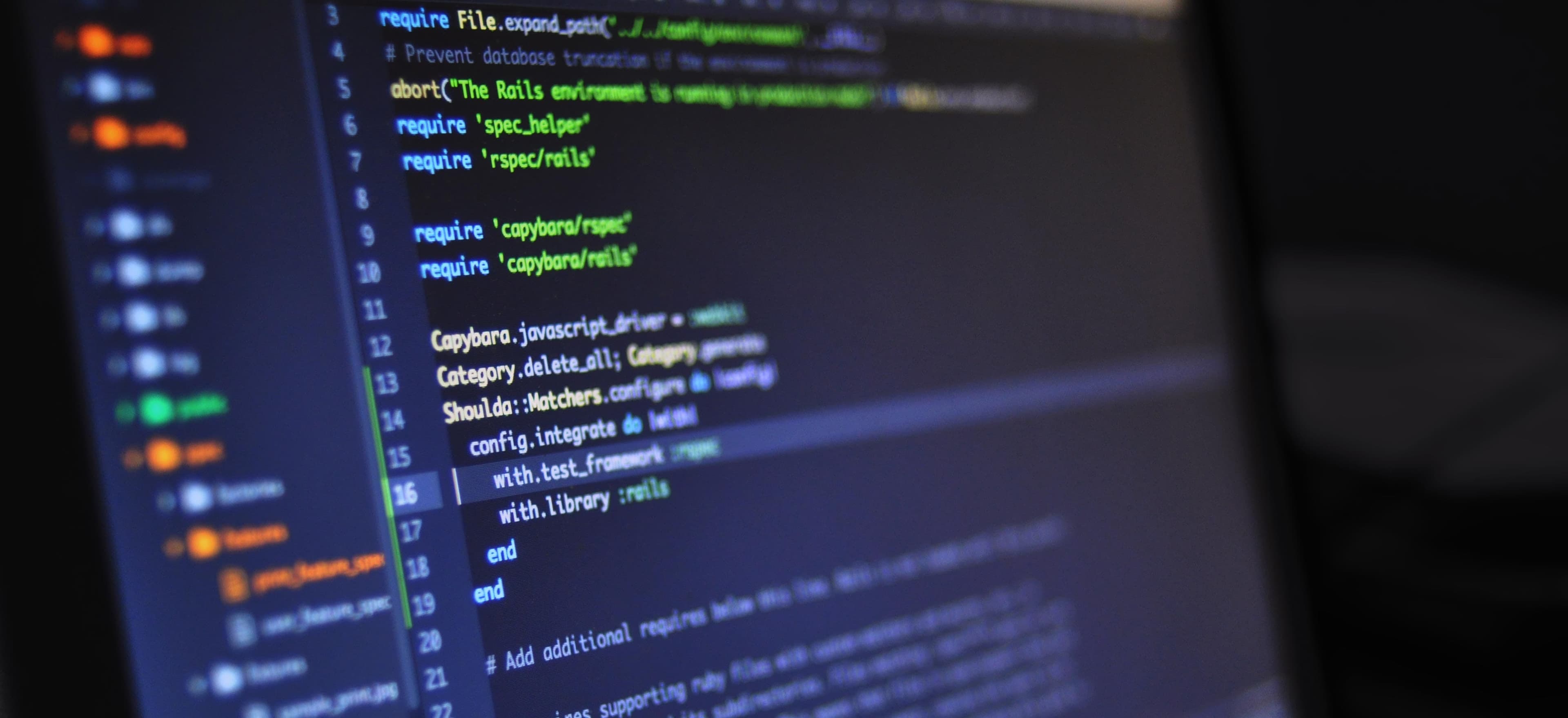
- Published on
Testing Grails 3.3 with Spock 1.1
When it comes to developing Java applications, testing is an integral part of the software development lifecycle. It ensures that the software works as expected, catching bugs and issues before they reach the end-users. In the Grails framework, testing plays a crucial role in maintaining the quality and reliability of applications. In this article, we will explore how to test a Grails 3.3 application using Spock 1.1, a powerful and expressive testing framework for Java and Groovy applications.
Setting Up the Environment
Before we dive into testing with Spock, let's ensure we have Grails 3.3 installed in our system. If not, you can follow the official guide for installing Grails.
Assuming you have Grails 3.3 set up, let's create a new Grails application by running the following command:
grails create-app myapp
cd myapp
Now that we have our Grails application set up, we can start adding tests using Spock.
Understanding Spock
Spock is a testing and specification framework for Java and Groovy applications. It provides a clear and expressive syntax for writing tests and is highly extensible. Spock tests are written in a behavior-driven development (BDD) style, making them easy to read and understand.
Writing a Simple Spock Specification
Let's start with a simple example to understand how Spock specifications work. In a Grails application, we often have domain classes that need to be tested. Assume we have a Book
domain class, and we want to test its functionality. We will create a Spock specification for this domain class.
Create a new file named BookSpec.groovy
inside the src/test/groovy
directory of your Grails application and add the following code:
import spock.lang.Specification
class BookSpec extends Specification {
def "test if the book title is capitalized"() {
given:
def book = new Book(title: "spock testing")
when:
def capitalizedTitle = book.title.capitalize()
then:
capitalizedTitle == "Spock Testing"
}
}
In this example, we are testing if the capitalize()
method of the Book
class capitalizes the title correctly. Let's break down the important parts of the Spock specification:
given:
block sets up the initial state for the test.when:
block performs the action to be tested.then:
block verifies the expected outcome of the action.
This is a basic example of a Spock specification. You can write more complex scenarios and use different Spock features to test various aspects of your Grails application.
Running the Spock Specifications
Now that we have our Spock specification in place, we can run the tests using the following Grails command:
grails test-app
This command will execute all the tests in your Grails application, including the Spock specifications.
Advanced Testing with Spock
Spock provides various features for advanced testing scenarios. Let's explore a few of them.
Mocking Collaborators
In many cases, our domain logic depends on collaborators such as services or repositories. Spock allows us to easily mock these collaborators to isolate the behavior being tested.
import spock.lang.Specification
import spock.lang.Mock
class BookServiceSpec extends Specification {
@Mock
BookRepository bookRepository
def "test if the book title is saved"() {
given:
def bookService = new BookService(bookRepository)
when:
bookService.saveBook("Spock Testing")
then:
1 * bookRepository.save(_)
}
}
In this example, we are testing the saveBook()
method of the BookService
class. We are using Spock's @Mock
annotation to mock the BookRepository
collaborator and verifying if its save()
method is called exactly once.
Data-Driven Testing
Spock supports data-driven testing, allowing us to run the same test with different inputs and expected outputs.
import spock.lang.Specification
import spock.lang.Unroll
class MathSpec extends Specification {
def "test if #a plus #b equals #c"() {
expect:
a + b == c
where:
a | b | c
2 | 2 | 4
3 | 5 | 8
}
}
In this example, we are testing the addition operation with different inputs using the where:
block. The @Unroll
annotation provides meaningful names for each data-driven test case.
Interaction-Based Testing
Spock provides a clean way to verify interactions between objects using the Interaction Based Testing
approach, which ensures that the objects under test collaborate correctly.
import spock.lang.Specification
import spock.lang.Mock
class PaymentServiceSpec extends Specification {
@Mock
PaymentGateway paymentGateway
def "test if payment is processed using the payment gateway"() {
given:
def paymentService = new PaymentService(paymentGateway)
when:
paymentService.processPayment(100)
then:
1 * paymentGateway.process(100)
}
}
In this example, we are testing if the processPayment()
method of the PaymentService
class correctly interacts with the PaymentGateway
collaborator.
The Closing Argument
In this article, we have explored how to test a Grails 3.3 application using Spock 1.1. We started by setting up the environment, understanding Spock, and writing a simple Spock specification. Then, we delved into advanced testing scenarios, including mocking collaborators, data-driven testing, and interaction-based testing.
Testing is a critical aspect of building robust and reliable applications. With Spock, you can write expressive and powerful tests to ensure the quality of your Grails applications. Embracing good testing practices will ultimately lead to higher confidence in your codebase and a better experience for your end-users. Start integrating Spock into your Grails projects and elevate your testing game to the next level!
Now that you have a grasp of testing with Spock in Grails, it's time to put your knowledge to work. Explore the Spock documentation and experiment with different testing scenarios to enhance the quality and reliability of your Grails applications.
Happy testing!