Improving Test Design for Enhanced Software Quality
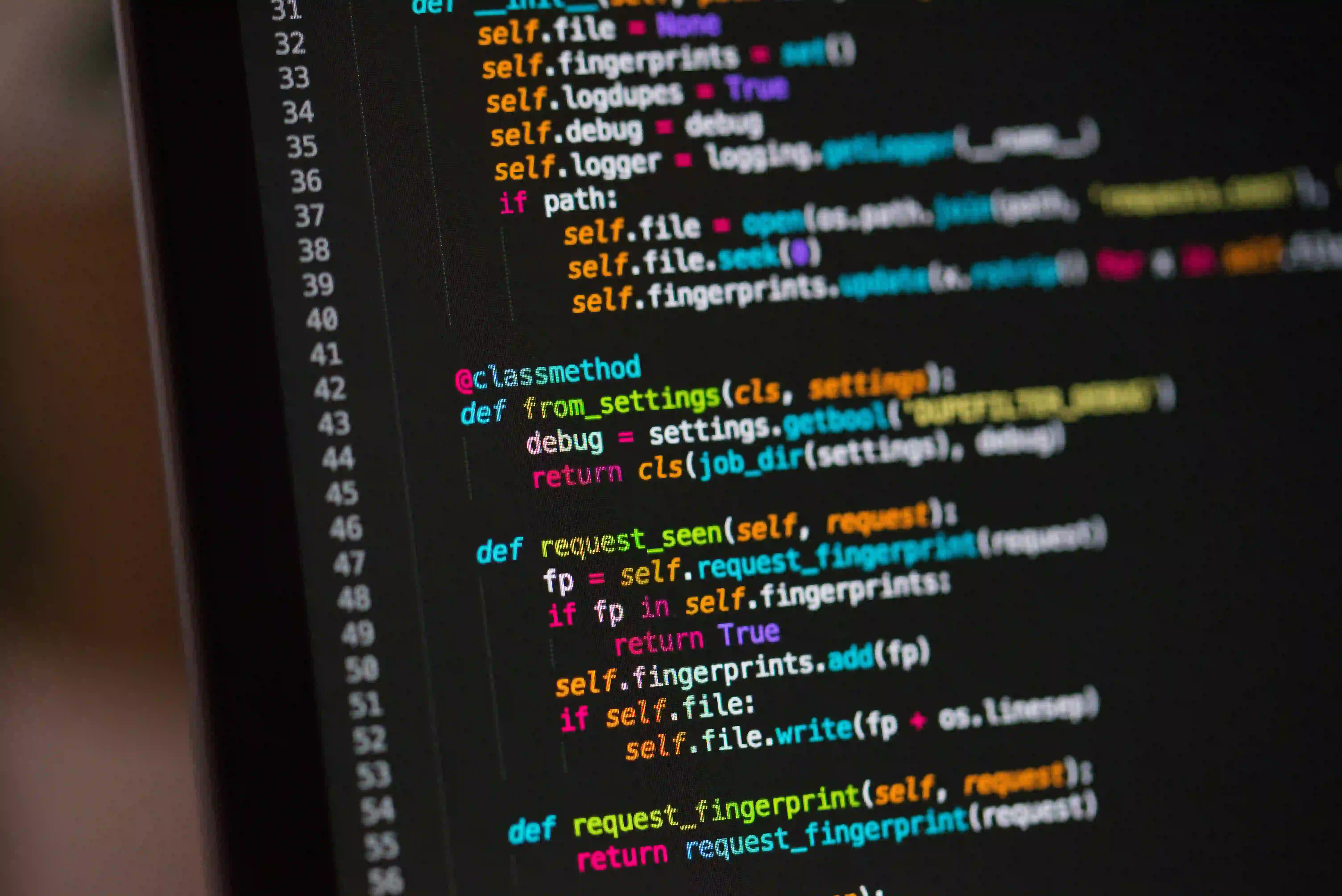
The Importance of Effective Test Design in Java
Software testing is a critical aspect of the software development lifecycle. It ensures that the software functions as expected, meets user requirements, and maintains its integrity. Java, being a widely-used programming language in the development of numerous applications, requires an efficient and robust test design to guarantee software quality.
Test Design Basics
Test design involves creating a set of test cases or scenarios that validate the functionality of the software. In Java, test design includes the creation of unit tests, integration tests, and end-to-end tests using frameworks like JUnit, TestNG, or Mockito.
Writing Effective Unit Tests in Java
Unit tests are crucial in Java development as they verify the behavior of individual components or units of code. Utilizing JUnit, a popular testing framework for Java, ensures that the code functions as intended on a granular level.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class ExampleUnitTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In the example above, a simple unit test using JUnit is demonstrated. The testAddition
method checks if the add
method of the Calculator
class correctly returns the sum of two numbers. This demonstrates the importance of writing unit tests to ensure the correctness of individual methods.
Conducting Effective Integration Tests
Integration tests are essential to validate the interactions between different modules or components of the software. When utilizing Spring Boot for building Java applications, tools such as @SpringBootTest
can enable effective integration testing.
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import static org.junit.jupiter.api.Assertions.*;
@SpringBootTest
public class ExampleIntegrationTest {
@Autowired
private UserService userService;
@Test
public void testUserCreation() {
User user = new User("John Doe");
userService.createUser(user);
assertNotNull(user.getId());
}
}
In the code snippet above, an integration test ensures that the createUser
method of the UserService
class effectively creates a user with a non-null ID. This showcases the significance of integration tests in Java applications to guarantee the coherence of component interactions.
Strategies for Improved Test Design
Embracing Test-Driven Development (TDD)
Test-Driven Development encourages writing tests before implementing the corresponding code. This approach leads to more focused code development and ensures that the code meets the specified requirements.
Incorporating Mocking Frameworks
Mocking frameworks such as Mockito or PowerMock provide the ability to create mock objects, allowing the isolation of the code under test. This separation is crucial for testing individual components without the reliance on external dependencies.
import static org.mockito.Mockito.*;
public class ExampleMockTest {
@Test
public void testPaymentProcessor() {
PaymentGateway mockedGateway = mock(PaymentGateway.class);
PaymentProcessor processor = new PaymentProcessor(mockedGateway);
when(mockedGateway.processPayment(anyDouble())).thenReturn(true);
assertTrue(processor.processPayment(100.0));
}
}
The code above demonstrates the usage of Mockito to mock a PaymentGateway
and verify the behavior of the processPayment
method in the PaymentProcessor
class. Employing mocking frameworks plays a crucial role in improving test design by enabling focused testing on specific components.
Applying Test Automation
Automating tests using tools like Selenium for end-to-end testing or Apache JMeter for performance testing significantly enhances the efficiency and effectiveness of the testing process.
Leveraging Continuous Integration and Continuous Deployment (CI/CD)
Integrating tests into the CI/CD pipeline ensures that any changes introduced to the codebase are immediately validated. Jenkins, GitLab CI, or Travis CI are common tools for automating the integration and deployment processes while incorporating the execution of test suites.
Wrapping Up
Incorporating effective test design practices in Java development is crucial for ensuring the reliability and quality of software. From unit testing with JUnit to implementing TDD and leveraging CI/CD pipelines, the significance of comprehensive testing cannot be overstated. By embracing these strategies, developers can enhance the overall software quality and deliver robust, reliable Java applications.
To delve deeper into the world of Java testing frameworks, check out JUnit's official site and the Mockito documentation. These resources provide invaluable insights into further advancing test design practices in Java.