Efficient String Data Compression in Java
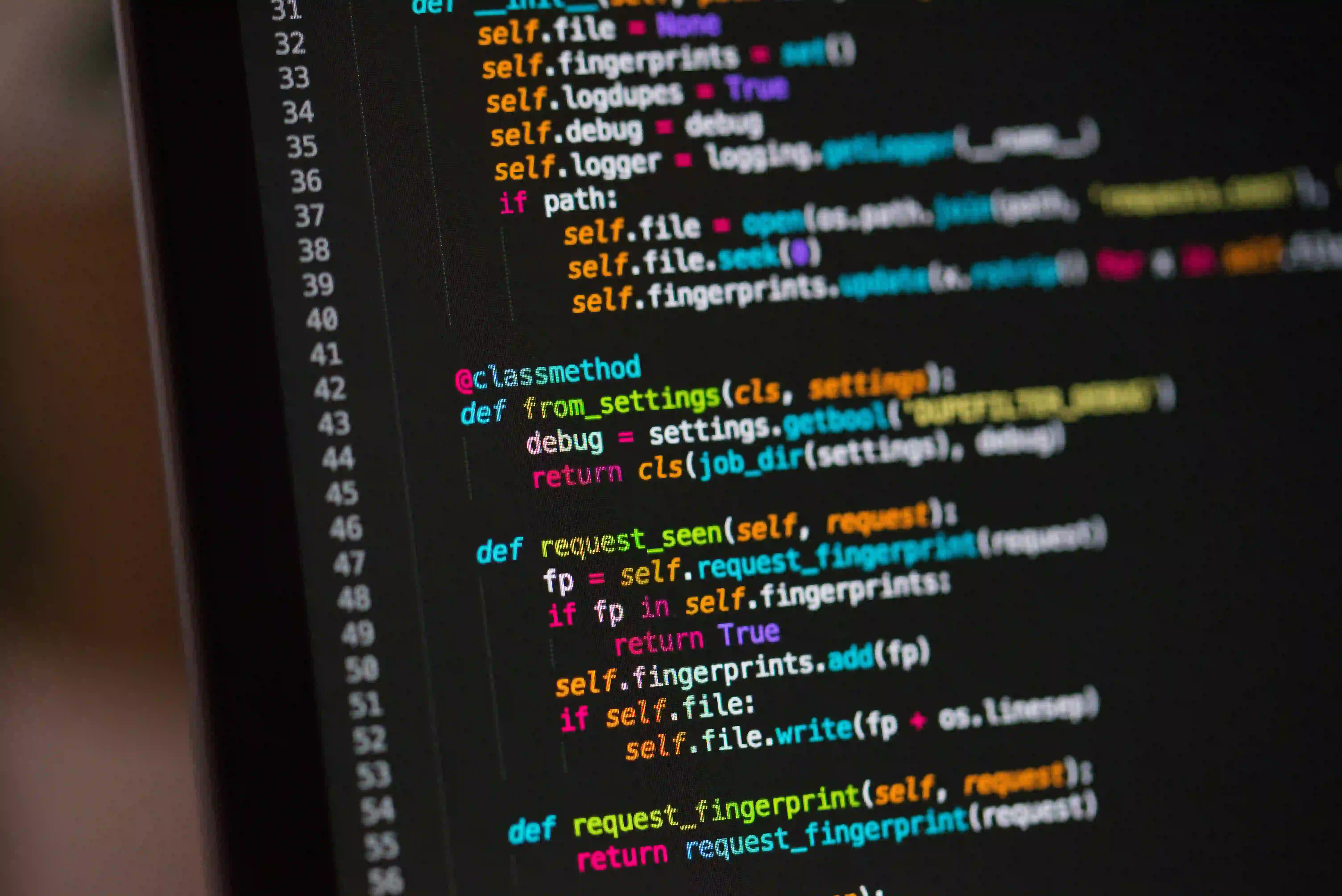
Efficient String Data Compression in Java
In the world of software development, the need to efficiently store and transmit data is ever-present. One common task is compressing strings for storage or transmission, and Java provides several approaches to accomplish this. In this blog post, we will explore various methods to efficiently compress string data in Java, and discuss their advantages and use cases.
Using Java's built-in compression libraries
Java provides support for data compression through its java.util.zip
package. This package contains classes such as Deflater
and Inflater
, which can be used to compress and decompress data using the DEFLATE algorithm, among others.
Example: Using Deflater
and Inflater
for String Compression
import java.util.zip.Deflater;
import java.util.zip.Inflater;
public class StringCompressionExample {
public static byte[] compressString(String input) {
byte[] inputData = input.getBytes();
Deflater deflater = new Deflater();
deflater.setInput(inputData);
deflater.finish();
byte[] compressedData = new byte[inputData.length];
int compressedSize = deflater.deflate(compressedData);
byte[] result = new byte[compressedSize];
System.arraycopy(compressedData, 0, result, 0, compressedSize);
deflater.end();
return result;
}
public static String decompressString(byte[] input) {
Inflater inflater = new Inflater();
inflater.setInput(input);
byte[] decompressedData = new byte[input.length * 3]; // Assuming three times the compressed size
int decompressedSize;
try {
decompressedSize = inflater.inflate(decompressedData);
} catch (DataFormatException e) {
// Handle exception
}
inflater.end();
return new String(decompressedData, 0, decompressedSize);
}
public static void main(String[] args) {
String originalString = "Lorem ipsum dolor sit amet, consectetur adipiscing elit.";
byte[] compressedString = compressString(originalString);
String decompressedString = decompressString(compressedString);
System.out.println("Original string: " + originalString);
System.out.println("Decompressed string: " + decompressedString);
}
}
In the above example, we use the Deflater
and Inflater
classes to compress and decompress a string, respectively. The compressed data is stored as a byte array, which can be easily stored or transmitted.
Using third-party libraries for more advanced compression
While Java's built-in compression utilities are useful for basic compression needs, there are third-party libraries that offer more advanced compression algorithms and techniques. One popular library for this purpose is Apache Commons Compress.
Example: Using Apache Commons Compress for LZMA Compression
import org.apache.commons.compress.compressors.CompressorStreamFactory;
import org.apache.commons.compress.compressors.CompressorOutputStream;
import org.apache.commons.compress.compressors.lzma.LZMACompressorOutputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class AdvancedStringCompression {
public static byte[] compressStringWithLZMA(String input) throws IOException {
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
try (CompressorOutputStream cos = new CompressorStreamFactory()
.createCompressorOutputStream(CompressorStreamFactory.LZMA, outputStream)) {
cos.write(input.getBytes());
}
return outputStream.toByteArray();
}
public static String decompressStringWithLZMA(byte[] input) throws IOException {
// Similar process for decompression
}
public static void main(String[] args) throws IOException {
String originalString = "Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.";
byte[] compressedString = compressStringWithLZMA(originalString);
// Decompress and use the data
}
}
In this example, we utilize Apache Commons Compress to compress a string using the LZMA algorithm. The library provides a wide range of compression formats and options, making it a versatile choice for advanced compression needs.
Considerations for choosing a compression method
When deciding on a compression method for string data in Java, several factors should be considered:
Compression ratio
Different compression algorithms offer varying levels of compression. Some algorithms may provide a higher compression ratio but at the cost of increased compression and decompression time. It's essential to assess the trade-offs between compression ratio and performance based on the specific use case.
Performance
The performance of compression and decompression operations can significantly impact the overall efficiency of an application. Depending on the requirements, such as real-time data transmission or batch processing, the performance characteristics of the chosen compression method should align with the application's needs.
Compatibility and interoperability
When selecting a compression method, it's crucial to consider the compatibility with other systems or components that will handle the compressed data. Additionally, interoperability with different programming languages or platforms may influence the choice of compression library or algorithm.
Use case-specific requirements
Different use cases may have specific requirements, such as streaming data, random access to compressed data, or long-term archival storage. Understanding these use case-specific requirements can help in selecting the most suitable compression approach.
Closing Remarks
Efficiently compressing string data in Java is a crucial aspect of many applications. By leveraging Java's built-in compression libraries or utilizing third-party libraries like Apache Commons Compress, developers can strike a balance between compression ratio, performance, and compatibility to meet their specific requirements.
Whether it's compressing data for storage, reducing network overhead, or optimizing memory usage, the choice of an appropriate compression method plays a significant role in the overall design and effectiveness of a Java application.
By carefully evaluating the factors such as compression ratio, performance, compatibility, and use case-specific requirements, developers can make informed decisions when it comes to selecting the right string compression approach for their Java projects.
In summary, effective string data compression in Java is not only about reducing the size of data but also about maintaining compatibility, optimizing performance, and meeting the specific needs of the application. With the right approach to string compression, Java developers can ensure efficient data handling and transmission in their software solutions.