Optimizing Incremental Migration in MongoDB
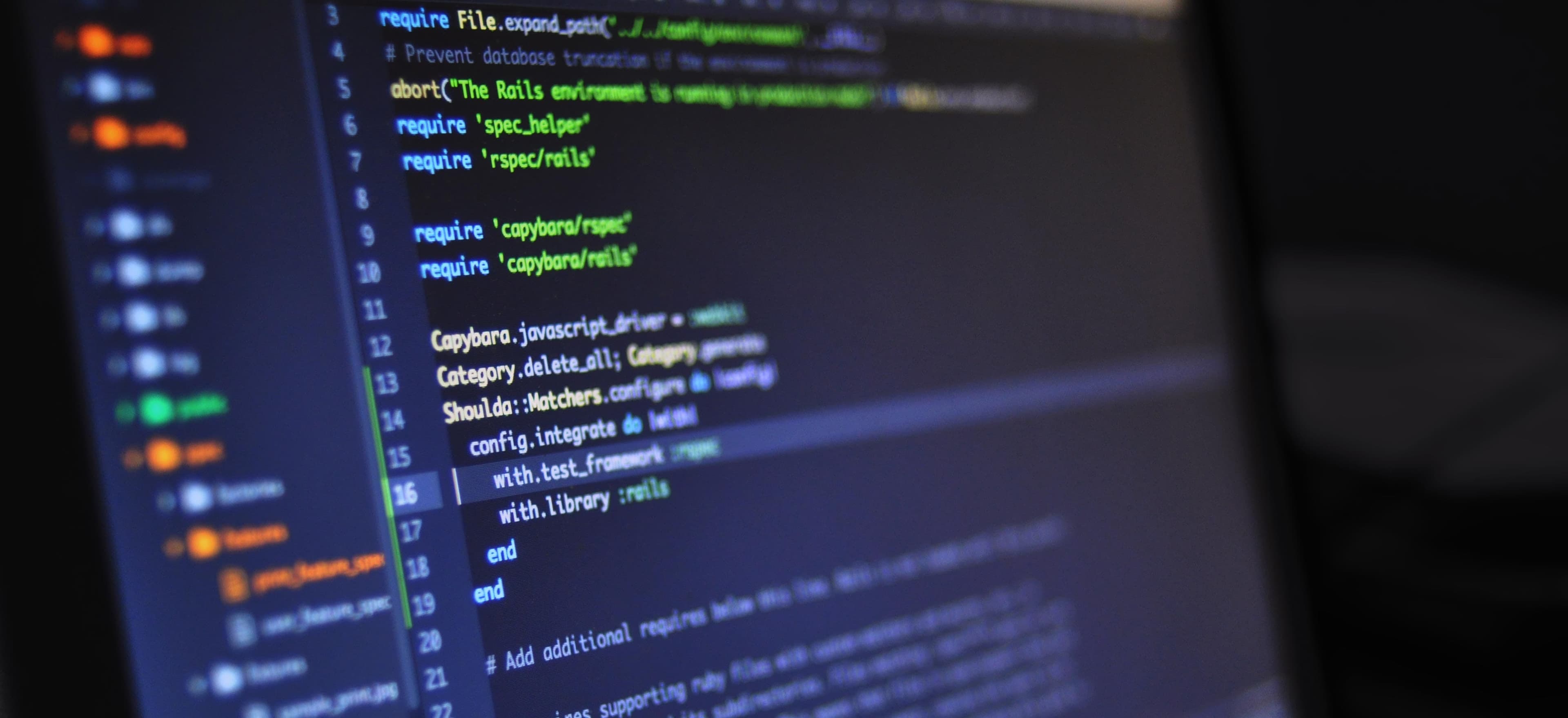
- Published on
Optimizing Incremental Migration in MongoDB
When dealing with large-scale applications, effective data migration is essential to ensure the smooth evolution of your databases. In the realm of NoSQL databases, MongoDB stands out as a popular choice, offering powerful features and flexibility. However, as your application grows, the need for efficient incremental migration becomes increasingly critical. In this post, we'll explore how to optimize incremental migration in MongoDB, leveraging Java and the MongoDB Java driver.
Understanding Incremental Migration
Before delving into optimization techniques, let's first grasp the concept of incremental migration. In the context of databases, incremental migration involves applying changes to the database schema and data in a controlled, step-by-step manner. This approach is particularly advantageous in scenarios where continuous deployment and frequent updates are the norm, as it allows for seamless adaptation to evolving requirements without disrupting the application.
In MongoDB, incremental migration typically involves updating the database schema (e.g., adding or removing fields) and migrating existing data to align with the new schema. This process needs to be carried out efficiently, especially when dealing with large datasets, to minimize downtime and maintain data consistency.
Leveraging the MongoDB Java Driver for Migration
The MongoDB Java driver provides a robust toolkit for interacting with MongoDB databases programmatically. To optimize incremental migration, we can leverage this driver to execute migration scripts within our Java application. Let's explore some key strategies for optimizing this process.
Efficient Data Retrieval and Manipulation
When migrating data in MongoDB, efficient data retrieval and manipulation are paramount. The MongoDB Java driver offers various methods for querying and updating documents in a performant manner. For instance, by utilizing the find
and updateOne
methods, we can selectively retrieve and update documents based on specific criteria, minimizing the impact on database performance.
// Example of using the MongoDB Java driver for data manipulation
MongoCollection<Document> collection = database.getCollection("myCollection");
Bson filter = Filters.eq("oldField", null);
Bson update = Updates.set("newField", "defaultValue");
collection.updateOne(filter, update);
In this snippet, we are updating documents by adding a new field (newField
) with a default value, based on a specific criterion. This targeted approach helps streamline the migration process while avoiding unnecessary overhead.
Bulk Operations for Performance
In scenarios where a large number of documents need to be migrated, employing bulk operations can significantly enhance performance. The MongoDB Java driver supports bulk write operations, allowing us to efficiently execute a high volume of update operations in a single request to the database.
// Example of utilizing bulk write operations for migration
List<WriteModel<Document>> bulkWrites = new ArrayList<>();
// Construct individual update operations and add them to bulkWrites
bulkWrites.add(new UpdateOneModel<>(Filters.eq("condition"), Updates.set("newField", "value")));
BulkWriteResult result = collection.bulkWrite(bulkWrites);
By aggregating multiple update operations into a single bulk request, we can minimize network overhead and expedite the migration process, ensuring optimal performance during incremental data migration.
Index Optimization for Query Performance
In the context of incremental migration, it's essential to optimize database indexes to support efficient querying during and after the migration process. The MongoDB Java driver allows us to create and manage indexes programmatically, enabling us to fine-tune index configurations to align with the evolving data model.
// Example of index creation using the MongoDB Java driver
collection.createIndex(Indexes.ascending("fieldToIndex"));
By strategically creating and updating indexes as part of the migration process, we can ensure that query performance remains optimal, even as the database schema undergoes changes.
Final Thoughts
In conclusion, optimizing incremental migration in MongoDB entails leveraging the capabilities of the MongoDB Java driver to execute efficient data retrieval, manipulation, and indexing operations. By harnessing targeted data manipulation, bulk write operations, and index optimization, we can streamline the migration process, minimize downtime, and uphold data consistency within our MongoDB databases. As you embark on optimizing incremental migration in your MongoDB-powered applications, keep in mind the performance implications of each migration approach and tailor your strategies to suit the specific characteristics of your data and workload.
By adhering to these best practices and harnessing the power of the MongoDB Java driver, you can elevate the efficiency and effectiveness of incremental migration, ensuring the seamless evolution of your MongoDB databases in tandem with your application's growth.