Configuring centralized properties in microservices architecture
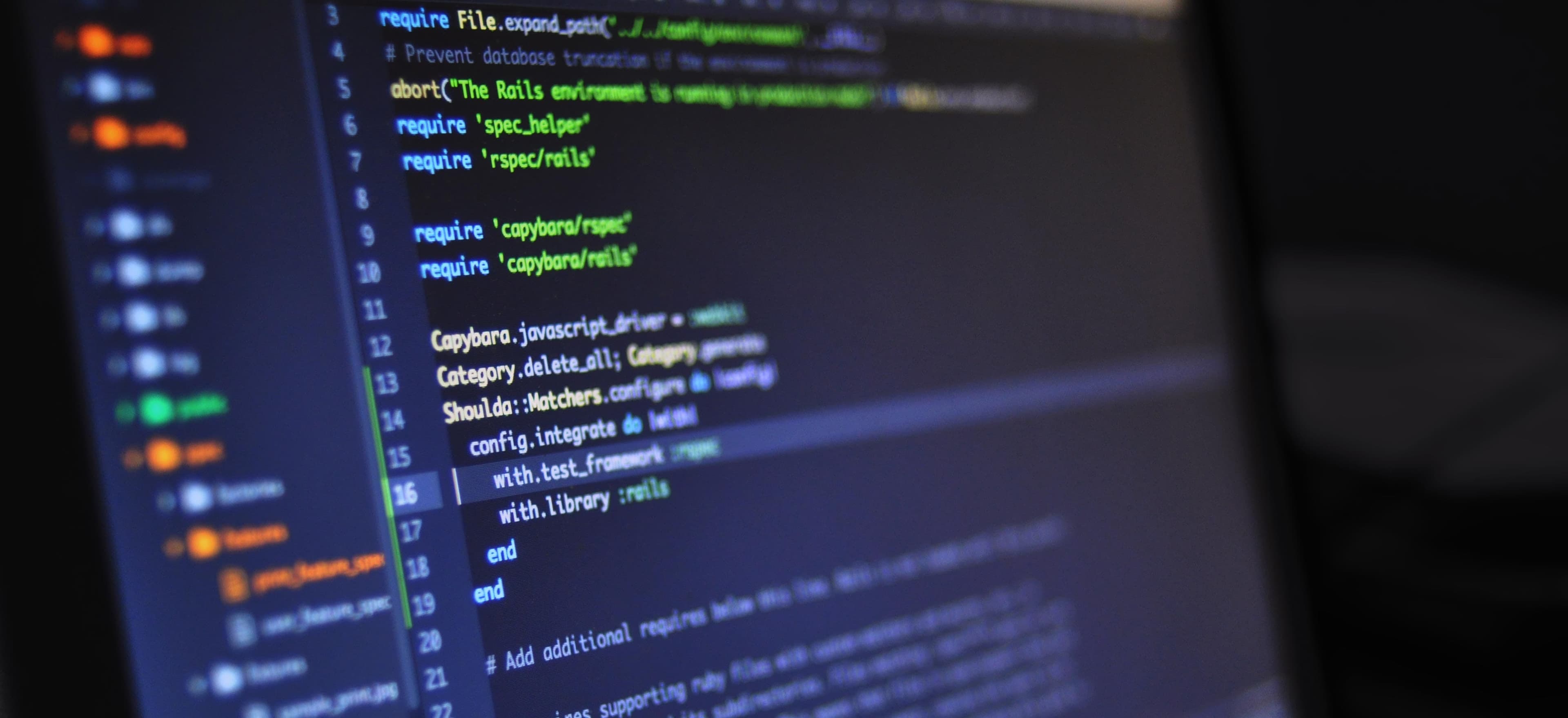
- Published on
Centralized Properties in Microservices Architecture Using Spring Cloud Config
In a microservices architecture, managing configuration settings for multiple services can become complex. Each service might have its configuration such as database connections, API keys, or feature toggles. Centralizing these configurations can simplify management, improve security, and enable easier changes across the architecture.
One popular solution for centralized configuration management in a microservices environment is Spring Cloud Config. Spring Cloud Config provides server and client-side support for externalized configuration in a distributed system. It also supports a variety of configuration sources such as Git repositories, Subversion repositories, and file system based settings.
In this blog post, we will explore how to set up centralized properties using Spring Cloud Config in a microservices architecture.
Setting up Spring Cloud Config Server
To get started, we need to set up a Spring Cloud Config Server to centralize the configurations. Below is a basic example of how to configure a Spring Cloud Config Server using Spring Boot.
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
This simple Spring Boot application with @EnableConfigServer
annotation enables the config server functionality. The server can be configured to fetch configuration from a designated source such as a Git repository.
Creating Configuration Files
Once the Spring Cloud Config Server is set up, we can create configuration files for our microservices. These configuration files can be in various formats such as properties, YAML, JSON, etc. Let's create a sample configuration file for a hypothetical microservice called user-service
.
# user-service.properties
server.port=8081
database.url=jdbc:mysql://localhost:3306/users
database.username=admin
database.password=secret
The above user-service.properties
file includes configurations for server port, database URL, username, and password. These configurations can be centralised, version-controlled, and managed through Spring Cloud Config.
Configuring Microservices to use Spring Cloud Config
Now, let's configure the user-service
to use Spring Cloud Config to fetch its configurations from the centralized server. Below is an example of how to configure a Spring Boot application to use Spring Cloud Config.
@SpringBootApplication
@EnableDiscoveryClient
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
# application.yml
spring:
application:
name: user-service
cloud:
config:
uri: http://localhost:8888
In the UserServiceApplication
class, we enable the Config Client functionality using @EnableDiscoveryClient
for service discovery and the application.yml
file includes the configuration to connect to the Spring Cloud Config Server.
Accessing Centralized Configuration
Now that the user-service
is configured to use Spring Cloud Config, it will fetch the configuration from the centralized server. We can access the configurations just like we would with local configuration files.
@RestController
public class UserController {
@Value("${server.port}")
private int serverPort;
@Value("${database.url}")
private String databaseUrl;
@GetMapping("/config")
public String getConfig() {
return "Server Port: " + serverPort + ", Database URL: " + databaseUrl;
}
}
In the UserController
class, we inject the configuration values using @Value
annotation. Now, when we access the /config
endpoint, the server port and database URL will be fetched from the centralized configuration.
Bringing It All Together
Centralized properties in a microservices architecture using Spring Cloud Config streamlines configuration management, promotes consistency, and allows for easier modifications across multiple services. With Spring Cloud Config Server and Client, operations teams can simplify the management of configurations, leading to more scalable and maintainable microservices architecture.
Setting up centralized properties with Spring Cloud Config in a microservices environment empowers teams to efficiently manage and update configurations, minimizing deployment risks and improving the agility of the system.
This approach helps in achieving a more organized and secure infrastructure. Centralized properties is a critical aspect of microservices deployment that shouldn't be overlooked, and Spring Cloud Config provides a robust solution for this requirement.
In conclusion, leveraging Spring Cloud Config for centralized properties in a microservices architecture is a best practice that enhances the overall manageability, scalability, and security of the system.
For further reading on Spring Cloud Config, you can refer to the official Spring Cloud Config documentation.
I hope you found this article helpful in understanding how to configure centralized properties in a microservices architecture using Spring Cloud Config. Thank you for reading!