Troubleshooting Groovy HTTP Server Issues
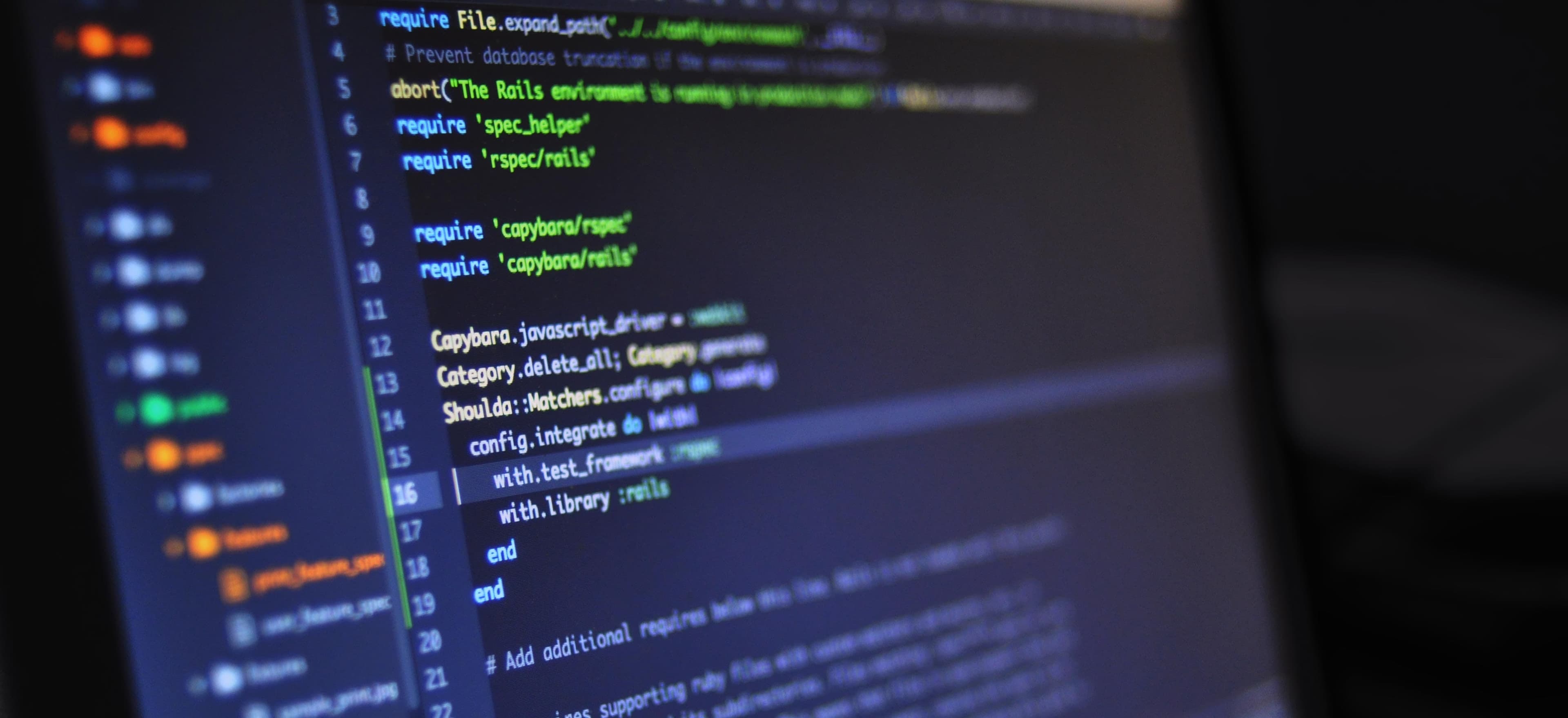
- Published on
Troubleshooting Groovy HTTP Server Issues
When working with Groovy, you may encounter issues with your HTTP server, causing frustration and delaying your development process. In this post, we'll explore common problems related to Groovy HTTP servers and how to troubleshoot and resolve them effectively.
Understanding Groovy HTTP Server
Before we delve into troubleshooting, let's have a quick overview of the Groovy HTTP server. Groovy provides a simple way to create an HTTP server using the groovyx.net.http.*
library. This library allows you to create server instances, handle requests, and send responses.
Common Issues and Solutions
1. Port Binding Errors
Issue: When trying to start the HTTP server, you may encounter a "Port already in use" error.
Solution: This error occurs when the specified port is already in use by another process. To resolve this, you can either change the port number in your server configuration or stop the process that's using the specified port. Additionally, you can use tools like netstat
to identify which process is using the port.
2. Request Handling Errors
Issue: Your server is running, but it fails to handle incoming requests correctly.
Solution: Check your request handling code for any potential issues. Make sure that your request mapping and handling logic are implemented correctly. Use logging and debugging tools to trace the flow of incoming requests and identify any errors in the request handling process.
3. SSL/TLS Configuration Issues
Issue: When configuring SSL/TLS for your HTTP server, you might encounter handshake or certificate errors.
Solution: Double-check your SSL/TLS configuration, including the certificate and key files. Ensure that the paths to these files are correctly specified in your server configuration. If you're using a self-signed certificate, make sure it's properly generated and trusted by the client making the request. You can also use online SSL/TLS testing tools to diagnose and resolve configuration issues.
4. Performance Bottlenecks
Issue: Your HTTP server experiences slow response times and high latency under load.
Solution: Evaluate your server's performance under load using profiling tools to pinpoint potential bottlenecks. Consider optimizing your request handling logic, using caching mechanisms, or scaling your server horizontally to distribute the load across multiple instances.
Troubleshooting Example
Let's consider an example where our Groovy HTTP server fails to handle a specific POST request correctly. The following code snippet represents a simplified version of our server implementation:
import groovyx.net.http.*
def server = HttpServer.create {
request.uri.path == '/api' && request.method == 'POST'
}
server.handler = { req, res ->
// Handle the POST request
// ...
}
server.start()
In this example, the server is intended to handle POST requests to the /api
endpoint. However, we're experiencing issues with the request handling logic. Let's troubleshoot this problem step by step.
First, let's ensure that the server is correctly configured to handle POST requests to the /api
endpoint. We'll add some debug logging to verify incoming requests:
server.handler = { req, res ->
if (req.method == 'POST' && req.uri.path == '/api') {
println("Received POST request to /api")
// Continue with request handling
} else {
res.status = 404
res.send("Not Found")
}
}
By adding logging, we can verify if the server is indeed receiving and identifying the POST requests to the /api
endpoint. This allows us to confirm if the issue lies in the request handling logic itself.
Next, we can inspect the request object to ensure that it contains the expected data. We can log the request body to identify any potential issues:
server.handler = { req, res ->
if (req.method == 'POST' && req.uri.path == '/api') {
println("Received POST request to /api")
println("Request body: ${req.body.toString()}") // Log the request body
// Continue with request handling
} else {
res.status = 404
res.send("Not Found")
}
}
By logging the request body, we can confirm if the server is receiving the expected data in the POST request.
If the issue persists, we can further debug and trace the flow of the request handling logic to identify any potential errors.
Closing the Chapter
In conclusion, troubleshooting Groovy HTTP server issues requires a systematic approach that involves verifying server configuration, request handling logic, and performance under load. By using logging, debugging, and profiling tools, you can effectively diagnose and resolve issues with your Groovy HTTP server.
Remember to refer to official Groovy documentation and community forums for additional support and insights into addressing specific issues with Groovy HTTP servers.
Keep learning and exploring, and happy coding!
Official Groovy HTTP Server Documentation Troubleshooting SSL/TLS Configuration