Common Heroku Errors Java Beginners Face and How to Fix Them
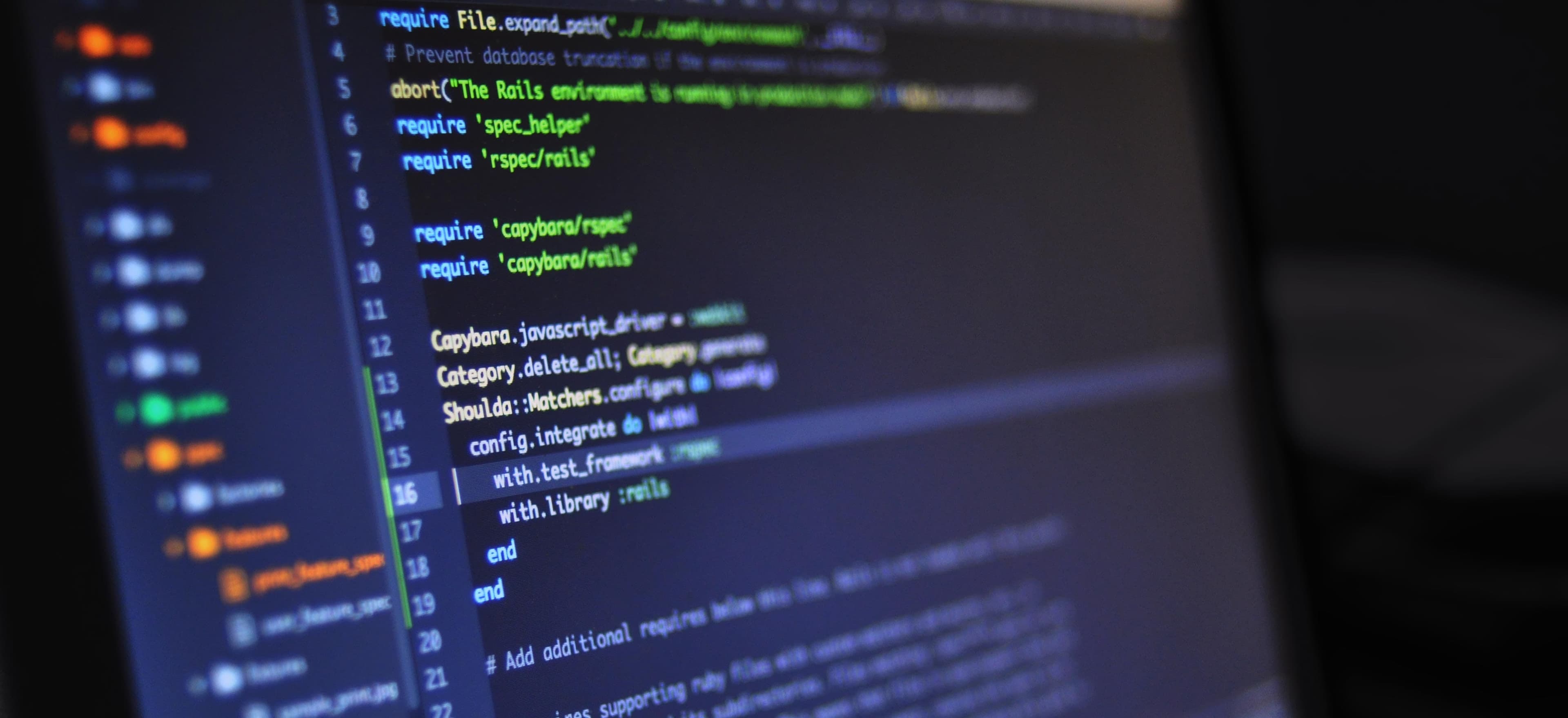
- Published on
Common Heroku Errors Java Beginners Face and How to Fix Them
Heroku is an amazing platform for deploying Java applications with ease. However, beginner developers often encounter various errors that can be frustrating. Understanding these common issues and their fixes is crucial for a smooth Heroku deployment experience. In this blog post, we'll discuss several common Heroku errors faced by Java beginners and provide actionable solutions to troubleshoot them.
Why Heroku?
Before we dive into common errors, let's briefly discuss why Heroku is popular among Java developers. Heroku is a cloud platform that allows developers to build, run, and operate applications entirely in the cloud.
- Ease of Use: Deploying applications can be done with simple Git commands.
- Scalability: Easily scale your application with just a few clicks.
- Integrated Add-Ons: Offers several add-ons for databases, caching, and other functionalities.
With this understanding, let’s explore some common errors and how to address them.
1. Buildpack Errors
Error Description
This is one of the most frequently encountered issues when deploying your Java application. A buildpack is responsible for determining how to set up your application. If Heroku can't find a suitable buildpack, it will fail during the deployment phase.
Solution
To set a Java buildpack explicitly, use the following command:
heroku buildpacks:set heroku/java
Why? This command ensures that Heroku uses the correct Java buildpack tailored for your application. Always ensure your system.properties
file specifies the correct Java version as well.
Example system.properties
Create or update your system.properties
file with the following content:
java.runtime.version=11
2. Application Crashes on Start
Error Description
After deployment, a common error is when the application crashes immediately upon starting. This can be due to various factors such as missing environment variables or misconfigurations in the application.
Solution
First, check the application logs to identify the reason for the crash:
heroku logs --tail
Why? This command provides you real-time logging from your Heroku application, which is invaluable for debugging.
Common Fixes
-
Environment variables: Ensure all required variables are set. You can set them using:
heroku config:set VAR_NAME=value
-
Database Configuration: If you are using a database, ensure your database configuration is correctly set up in your
application.properties
or equivalent configuration file.
3. Port Binding Errors
Error Description
Heroku dynamically assigns a port for your application to listen on. If your application is hardcoded to listen on a fixed port (like 8080
), it will fail.
Solution
Modify your application to use the port assigned by Heroku via the PORT
environment variable. Here's how to do this in a Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
// Get the port from the environment variable, or default to 8080
int port = Integer.parseInt(System.getenv("PORT", "8080"));
SpringApplication.run(MyApplication.class, args);
}
}
Why? This code snippet ensures that your application listens on the port provided by Heroku, avoiding crashes caused by failed port bindings.
4. Database Connection Issues
Error Description
Another common error among beginners is improper database connection setup, resulting in SQLExceptions or connection timeouts.
Solution
-
Ensure correct JDBC URL: The JDBC URL should be formatted according to your database type. Here’s an example for PostgreSQL:
jdbc:postgresql://<DATABASE_URL>
-
Spring configuration example:
Here's how you might configure your application.properties
for PostgreSQL:
spring.datasource.url=${JDBC_DATABASE_URL}
spring.datasource.username=${DATABASE_USERNAME}
spring.datasource.password=${DATABASE_PASSWORD}
Why? Using environment variables to set up configuration allows Heroku to manage sensitive information.
5. Memory Limits Exceeded
Error Description
Heroku free dynos come with limited memory. If your application exceeds this limit, it will crash or restart frequently.
Solution
- Optimize your application: Profile your application to look for memory leaks or inefficiencies.
- Use lower memory settings: If applicable, lower the memory footprint of your application.
Monitoring Resource Usage is essential. Use the following command to check your app’s performance:
heroku ps
Why? Monitoring helps you identify and address the resource consumption of your application.
6. Timeouts during Requests
Error Description
Sometimes you might experience request timeouts if your web requests take too long to process. Heroku has a default timeout of 30 seconds for web requests.
Solution
- Optimize long-running tasks: Consider using background processing for lengthy requests. Libraries like Spring Batch or Async APIs can help.
- Check database queries: Ensure that your database queries are efficient and indexed properly.
The Bottom Line
Deploying Java applications on Heroku is generally a straightforward process, but beginners can encounter various errors that may hinder productivity. Understanding these common issues—from buildpack problems to database connection errors—will empower you to fix or avoid them altogether.
Heroku's intuitive interface and robust infrastructure make it an excellent platform for Java developers, offering the ability to scale applications effortlessly. As you continue your journey, remember to leverage logging, optimize your code, and utilize the support resources available.
For more information and troubleshooting techniques, refer to the official Heroku Java Support page and explore additional resources relevant to your specific project requirements.
Happy coding!