Integrating Moxy for Smooth JSON Binding
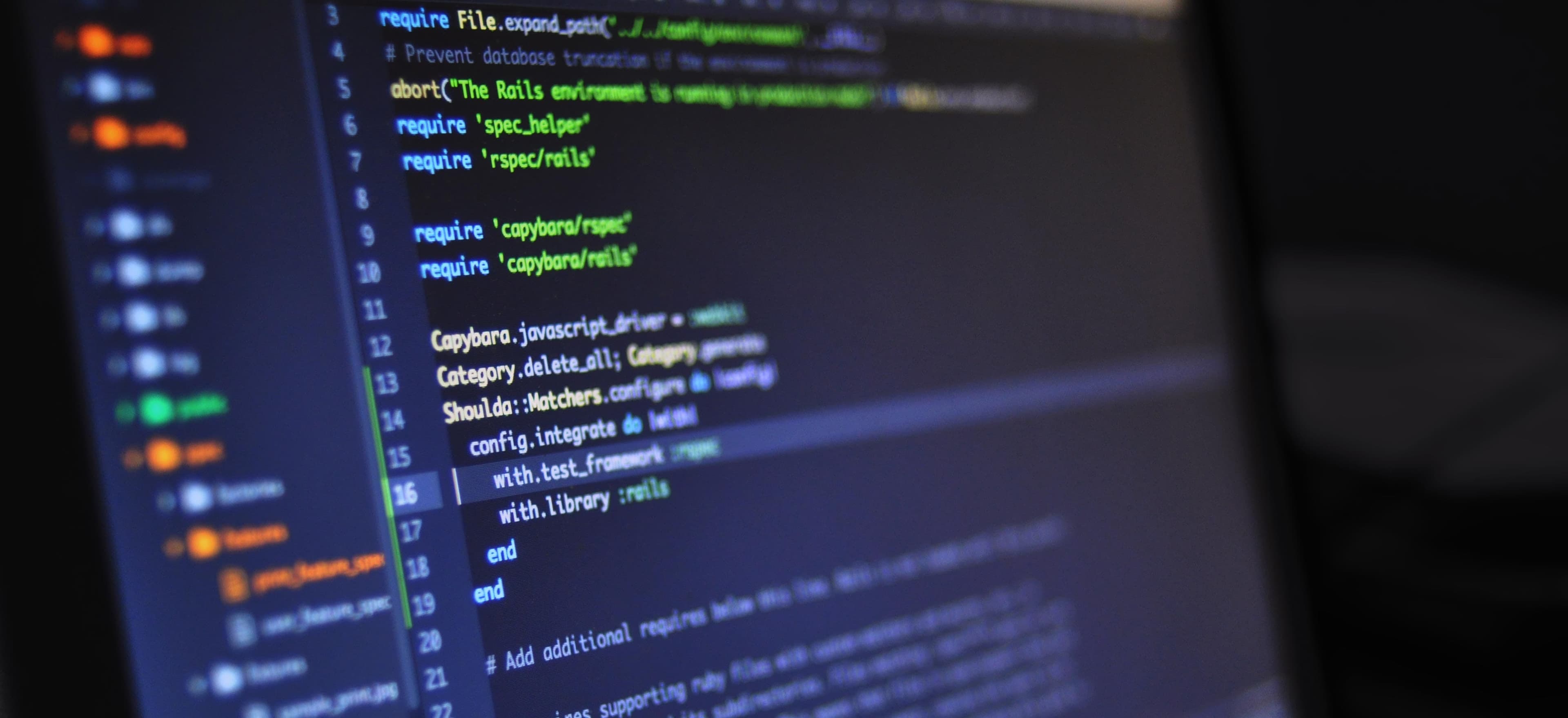
- Published on
Integrating Moxy for Smooth JSON Binding
In the world of Java development, working with JSON data is a common task. Whether you are building a web application, a RESTful API, or simply need to handle data exchange between different systems, being able to efficiently serialize and deserialize JSON is crucial. This is where Moxy, a component of EclipseLink, comes into play. In this blog post, we will discuss how to integrate Moxy into your Java project to achieve smooth JSON binding.
What is Moxy?
Moxy is a Java library that provides support for binding Java objects to XML and JSON representations. It is a part of EclipseLink, which is an advanced, open-source Object-Relational Mapping (ORM) framework. Moxy utilizes JAXB (Java Architecture for XML Binding) annotations to customize the mapping between Java objects and their JSON representations. By leveraging Moxy, developers can seamlessly convert Java objects to JSON and vice versa, with minimal effort and maximum flexibility.
Setting Up Moxy in Your Project
To integrate Moxy into your Java project, you can leverage Maven or Gradle to add the required dependencies. Let's consider a Maven-based project for the purpose of illustration. In your pom.xml
file, include the following dependencies:
<dependencies>
<!-- Other dependencies -->
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>eclipselink</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>org.eclipse.persistence.moxy</artifactId>
<version>3.0.0</version>
</dependency>
</dependencies>
By adding these dependencies, you ensure that Moxy and its associated libraries are included in your project.
Annotating Java Objects for JSON Binding
Moxy leverages JAXB annotations to customize the JSON binding behavior. Let's consider a simple example where we have a Person
class that we want to serialize to JSON. We can use Moxy annotations to achieve this:
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlElement;
@XmlRootElement
@XmlAccessorType(XmlAccessType.FIELD)
public class Person {
@XmlElement
private String name;
@XmlElement
private int age;
// Getters and setters
}
In the example above, we use the @XmlRootElement
annotation to specify that the Person
class is the root element for serialization. Additionally, the @XmlElement
annotations are used to indicate the fields that should be included in the JSON representation. This level of annotation-based customization allows for precise control over the JSON binding process.
Configuring Moxy for Your Application
Once you have annotated your Java objects, you need to configure Moxy within your application. One way to achieve this is by creating a MoxyJsonContextResolver
class that extends javax.ws.rs.ext.ContextResolver
for JAX-RS applications. This class provides configuration for Moxy's JSON binding behavior.
import javax.ws.rs.ext.ContextResolver;
import javax.ws.rs.ext.Provider;
import org.eclipse.persistence.jaxb.JAXBContext;
import org.eclipse.persistence.jaxb.JAXBContextProperties;
@Provider
public class MoxyJsonContextResolver implements ContextResolver<JAXBContext> {
private final JAXBContext context;
public MoxyJsonContextResolver() {
this.context = createMoxyJsonContext();
}
@Override
public JAXBContext getContext(Class<?> objectType) {
return context;
}
private JAXBContext createMoxyJsonContext() {
Map<String, Object> properties = new HashMap<>();
properties.put(JAXBContextProperties.JSON_INCLUDE_ROOT, false);
return (JAXBContext) org.eclipse.persistence.jaxb.JAXBContextFactory.createContext(new Class[]{Person.class}, properties);
}
}
In the MoxyJsonContextResolver
class, we create a JAXBContext
object with specific properties to customize the JSON binding behavior. We disable including the root element in the JSON representation using the JAXBContextProperties.JSON_INCLUDE_ROOT
property, and we specify the classes to be used for JSON serialization.
Using Moxy for JSON Binding
After the setup and configuration, using Moxy for JSON binding is straightforward. Let’s see how we can serialize a Person
object to JSON and deserialize JSON back to a Person
object.
Serializing to JSON
import org.eclipse.persistence.jaxb.JAXBContext;
import org.eclipse.persistence.jaxb.MarshallerProperties;
// Create a JAXBContext
JAXBContext jc = (JAXBContext) JAXBContextFactory.createContext(new Class[]{Person.class}, null);
// Create a Marshaller
Marshaller marshaller = jc.createMarshaller();
marshaller.setProperty(MarshallerProperties.MEDIA_TYPE, "application/json");
marshaller.setProperty(MarshallerProperties.JSON_INCLUDE_ROOT, false);
// Serialize the Person object to JSON
StringWriter writer = new StringWriter();
marshaller.marshal(personObj, writer);
String jsonString = writer.toString();
In the above code snippet, we create a JAXBContext
and a Marshaller
, and then serialize the Person
object to JSON. We set the media type to JSON and disable including the root element in the JSON representation.
Deserializing from JSON
import org.eclipse.persistence.jaxb.JAXBContext;
import org.eclipse.persistence.jaxb.UnmarshallerProperties;
// Create a JAXBContext
JAXBContext jc = (JAXBContext) JAXBContextFactory.createContext(new Class[]{Person.class}, null);
// Create an Unmarshaller
Unmarshaller unmarshaller = jc.createUnmarshaller();
unmarshaller.setProperty(UnmarshallerProperties.MEDIA_TYPE, "application/json");
// Deserialize JSON to a Person object
StringReader reader = new StringReader(jsonString);
Person person = (Person) unmarshaller.unmarshal(reader);
In the code above, we create a JAXBContext
and an Unmarshaller
, and then deserialize the JSON string back to a Person
object.
Summary
In this blog post, we have explored the integration of Moxy for smooth JSON binding in Java applications. We discussed setting up Moxy in a Maven project, annotating Java objects for JSON binding, configuring Moxy for the application, and using Moxy for both JSON serialization and deserialization. With Moxy, developers can efficiently handle JSON data without sacrificing flexibility or customization.
By leveraging Moxy's powerful capabilities, developers can streamline JSON binding, simplify data exchange, and enhance the interoperability of Java applications. Whether you are working on a web service, a microservice, or any other Java application that deals with JSON data, Moxy can be a valuable addition to your toolkit.
As the world of Java development continues to evolve, Moxy stands as a reliable and efficient solution for JSON binding, offering a seamless integration that empowers developers to focus on building robust and innovative applications. With its flexibility and ease of use, Moxy is a valuable asset for any Java developer looking to optimize their JSON handling capabilities.
Checkout our other articles