Troubleshooting Common NFC Issues in Android
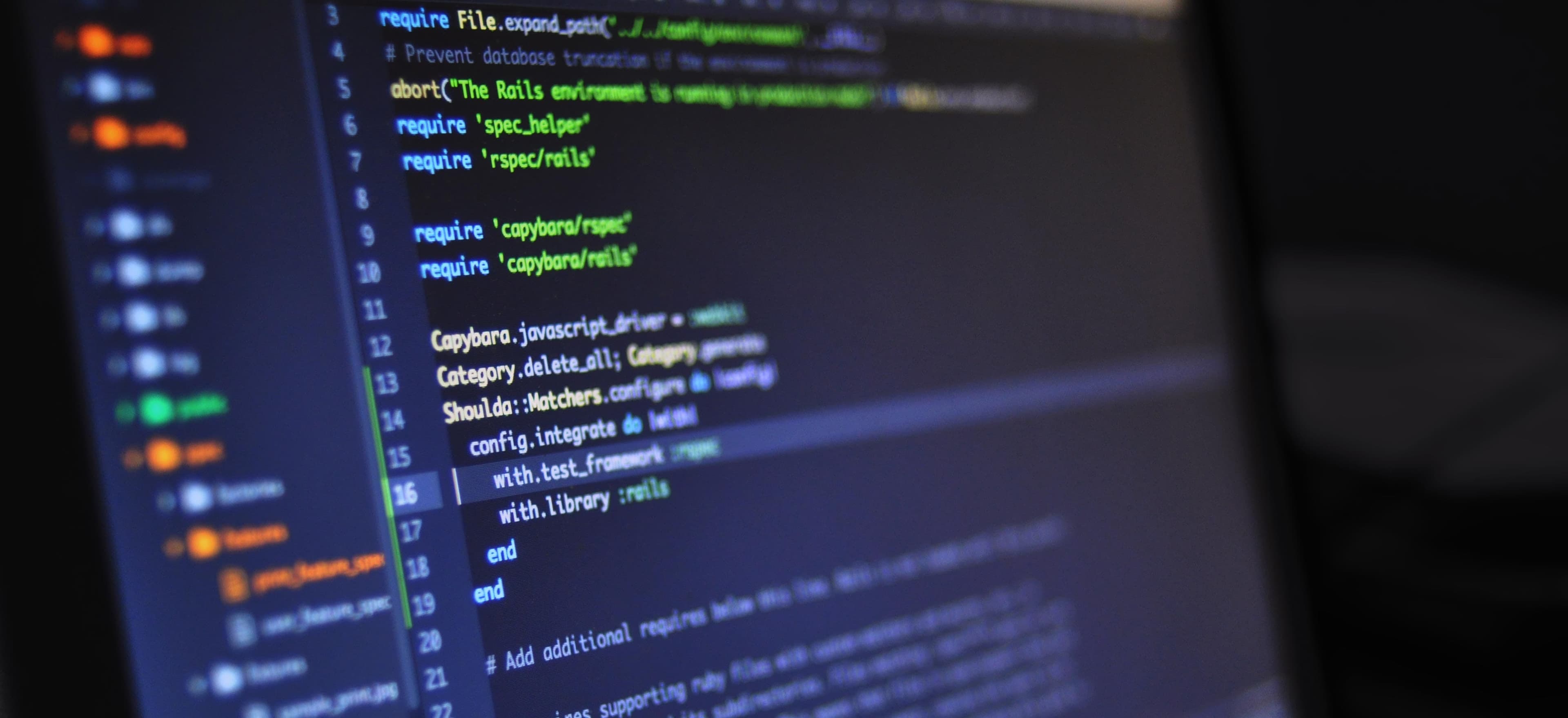
- Published on
Troubleshooting Common NFC Issues in Android
NFC (Near Field Communication) is a powerful technology that allows devices to communicate and share data when they are in close proximity. In Android, NFC is widely used for various purposes, such as mobile payments, sharing contacts, and pairing Bluetooth devices. However, like any technology, NFC can sometimes encounter issues that need to be addressed.
In this article, we will discuss some common NFC issues that Android developers and users may encounter, along with troubleshooting steps to resolve them.
1. NFC Not Enabled
Issue
One common issue users face is that NFC is not enabled on their device, preventing them from using NFC-related features and applications.
Resolution
To enable NFC on an Android device, users can follow these steps:
- Go to the device's Settings.
- Select "Connected devices" or "Wireless & networks."
- Toggle the NFC switch to enable it.
Code Sample (if applicable)
NfcAdapter nfcAdapter = NfcAdapter.getDefaultAdapter(context);
if (nfcAdapter != null && !nfcAdapter.isEnabled()) {
// NFC is not enabled, prompt the user to enable it
}
Why
This code snippet checks if NFC is enabled on the device and prompts the user to enable it if it's not. This proactive approach can enhance the user experience and prevent issues related to NFC functionality.
2. NFC Communication Failure
Issue
Another common issue is the failure of NFC communication between devices, leading to unsuccessful data transfer or transaction.
Resolution
To troubleshoot NFC communication failures, consider the following:
- Ensure that the devices are held close enough for NFC communication to occur (typically within 4 centimeters).
- Check for physical obstructions, such as cases or covers, that may interfere with NFC signals.
- Verify that both devices have NFC functionality and it is enabled.
Why
Proximity is crucial for successful NFC communication. By addressing physical obstructions and verifying NFC functionality, you can improve the chances of successful data exchange.
3. Handling NFC Intent
Issue
Developers may encounter issues with handling NFC intents in their Android applications, resulting in the inability to receive or process NFC data.
Resolution
When working with NFC intents, ensure that the intent filters are appropriately set up in the manifest file to capture NFC events. Additionally, handle the NFC intents in the foreground of the application to process the received data promptly.
Code Sample (if applicable)
@Override
protected void onNewIntent(Intent intent) {
if (NfcAdapter.ACTION_NDEF_DISCOVERED.equals(intent.getAction())) {
// Process the NFC NDEF payload
}
}
Why
Properly setting up intent filters and processing NFC intents in a timely manner is essential for seamless communication between the Android device and NFC tags or other devices.
4. NFC Tag Not Detected
Issue
Users may experience difficulty in detecting NFC tags, impeding interactions with NFC-enabled objects or devices.
Resolution
When troubleshooting NFC tag detection issues, consider the following:
- Verify that the NFC tag is compatible with the device and meets the required standards (e.g., NDEF format for data exchange).
- Ensure that the NFC tag is held within close proximity to the device's NFC antenna, as specified by the device manufacturer.
Why
Compatibility and proximity are key factors in the successful detection of NFC tags. By confirming these aspects, users can overcome issues related to tag detection.
5. Handling Unsupported NFC Formats
Issue
Unsupported NFC formats, such as proprietary or non-standardized data, can lead to compatibility issues and data misinterpretation.
Resolution
To handle unsupported NFC formats, developers should implement robust data validation and error-handling mechanisms to gracefully manage unexpected NFC data formats. Additionally, educating users about supported NFC formats can help mitigate data interpretation issues.
Why
Implementing robust data validation and error-handling mechanisms can prevent application crashes or incorrect data processing, ultimately enhancing the overall NFC user experience.
In Conclusion, Here is What Matters
In this blog post, we covered several common NFC issues in Android and provided practical troubleshooting steps to address them. By enabling NFC, ensuring proper device proximity, handling NFC intents, verifying tag compatibility, and managing unsupported NFC formats, users and developers can overcome NFC-related challenges and optimize the functionality of NFC-enabled applications.
For further insights into NFC technology and its applications in Android development, consider exploring Google's official documentation and the NFC Forum's resource library.
By addressing these common NFC issues, Android users and developers can harness the full potential of NFC technology for seamless data exchange and enhanced user experiences.
Checkout our other articles