Optimizing Test Suite Setup with JUnit 5
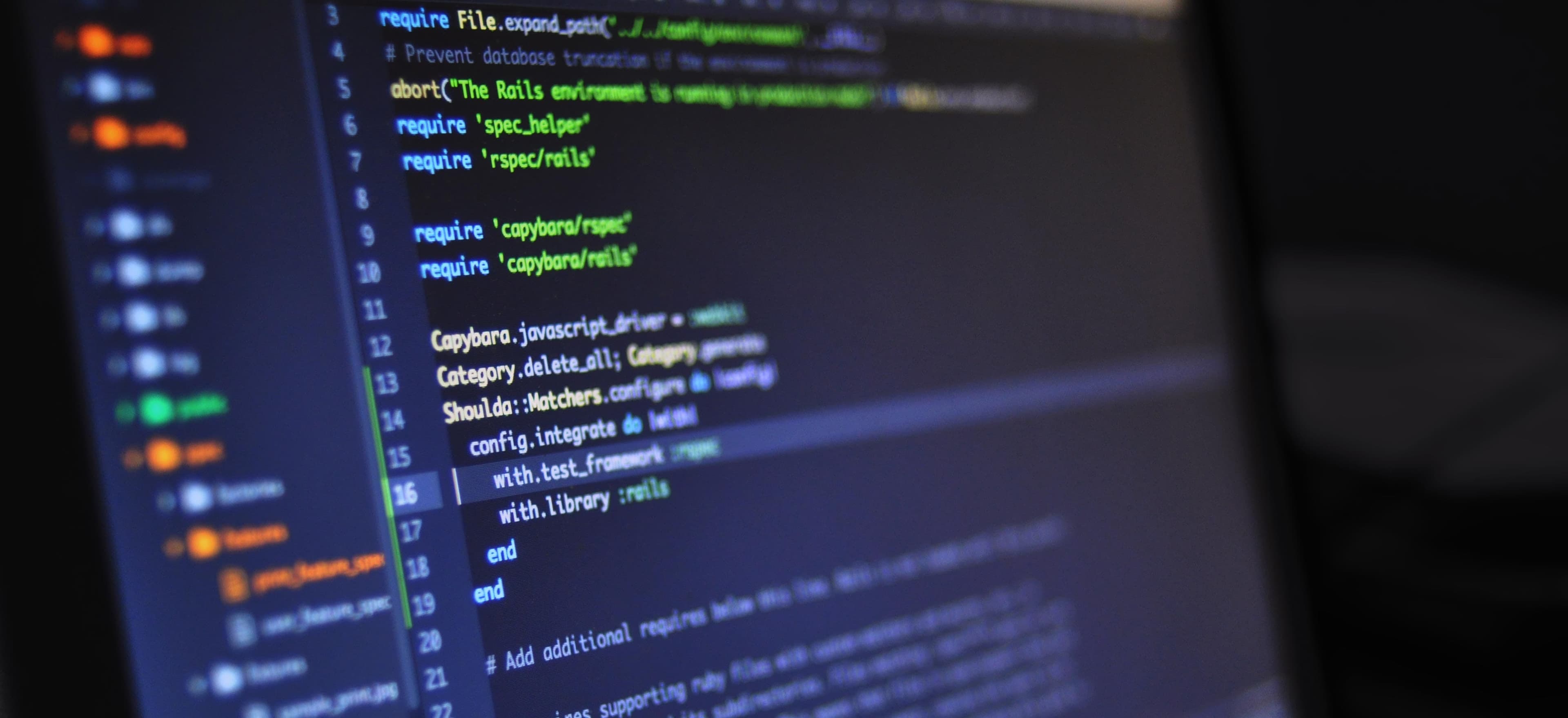
- Published on
Optimizing Test Suite Setup with JUnit 5
When it comes to writing efficient and maintainable code, automated testing plays a crucial role. Java developers often rely on JUnit, one of the most popular unit testing frameworks, to ensure the reliability of their code. With the release of JUnit 5, significant improvements have been made to make testing in Java more powerful and efficient.
In this blog post, we'll explore how to optimize test suite setup using JUnit 5 to improve the overall testing process.
Understanding Test Suite
In JUnit, a test suite is a collection of test cases that are used to test a specific behavior of a program. JUnit 4 provided the @RunWith
annotation to create test suites, but JUnit 5 has introduced a more flexible and efficient way of creating test suites.
JUnit 5 Test Suites
In JUnit 5, test suites are created using the @SelectPackages
and @SelectClasses
annotations in combination with @RunWith
and @Suite
from JUnit 4. This allows for more dynamic and fine-grained control over which tests are included in the suite.
Let's dive into an example to showcase the power of test suites in JUnit 5.
Example
Suppose we have the following test classes:
package com.example.tests;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class MathUtilsTest {
@Test
void testAddition() {
// Test addition functionality
}
}
package com.example.tests;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class StringUtilsTest {
@Test
void testConcatenation() {
// Test string concatenation functionality
}
}
Creating a Test Suite
We want to create a test suite that includes both MathUtilsTest
and StringUtilsTest
. Here's how we can achieve that using JUnit 5:
package com.example.tests;
import org.junit.platform.runner.JUnitPlatform;
import org.junit.platform.suite.api.SelectClasses;
import org.junit.runner.RunWith;
@RunWith(JUnitPlatform.class)
@SelectClasses({ MathUtilsTest.class, StringUtilsTest.class })
public class TestSuite {
}
In this example, we created a test suite TestSuite
using the @RunWith
annotation from JUnit 4 and the @SelectClasses
annotation from JUnit 5. The @SelectClasses
annotation allows us to specify the test classes we want to include in the test suite.
Benefits of Optimizing Test Suite Setup
Optimizing test suite setup with JUnit 5 offers several benefits:
1. Improved Execution Time
By selectively including only relevant test classes in the test suite, the overall execution time of the test suite is reduced. This is especially beneficial in large codebases with numerous test classes.
2. Enhanced Readability and Maintenance
A well-organized test suite enhances the readability of the test code and makes it easier to maintain. When new test cases are added, or existing ones are modified, having a well-structured test suite ensures that only relevant tests are executed.
3. Granular Control over Testing
With JUnit 5 annotations such as @Tag
and @DisplayName
, it is easier to categorize and filter tests based on specific criteria. This granularity of control allows for running subsets of tests based on different classifications, such as smoke tests, regression tests, and so on.
4. Seamless Integration with IDEs and Build Tools
JUnit 5's test suites seamlessly integrate with popular IDEs and build tools, allowing for easy execution and reporting of test results. This makes it easier for developers to run and manage tests within their preferred development environment.
Best Practices for Test Suite Optimization
To fully leverage the potential of test suite optimization with JUnit 5, it's essential to follow best practices:
1. Organize Test Classes
Organize test classes into meaningful packages and ensure clear naming conventions. This simplifies the process of selecting classes for inclusion in the test suite using @SelectPackages
.
2. Use Tags for Categorization
Employ JUnit 5's @Tag
annotation to tag test classes and methods based on their characteristics. This allows for selective inclusion or exclusion of tests based on specific tags, enhancing test suite flexibility.
3. Utilize Dynamic Test Discovery
JUnit 5's dynamic test discovery feature automatically identifies and executes test classes and methods, eliminating the need for manual maintenance of test suites as the codebase evolves.
4. Continuous Refinement
Regularly review and refine the test suite to ensure that it accurately reflects the evolving codebase. This includes adding new tests, removing outdated ones, and adjusting test class inclusion based on changing requirements.
Key Takeaways
Optimizing test suite setup with JUnit 5 is a fundamental aspect of ensuring efficient and effective testing in Java. By using the powerful features provided by JUnit 5, such as dynamic test discovery and fine-grained control over test selection, developers can significantly enhance the testing process. Incorporating best practices and leveraging the flexibility of JUnit 5 annotations empowers teams to create robust, maintainable, and scalable test suites.
Embracing these principles not only leads to faster and more reliable testing but also contributes to overall code quality and developer productivity.
Incorporating JUnit 5's advanced suite configuration capabilities enhances the testing process, making it more efficient and reliable for Java developers.
With these strategies in place, Java developers can optimize their test suites for improved testing processes. Taking advantage of JUnit 5's powerful features and incorporating best practices ensures that test suites are not only efficient but also remain flexible and adaptable.
By following these principles and utilizing JUnit 5's advanced suite configuration capabilities, developers can significantly enhance their testing processes, leading to more efficient, reliable, and maintainable code.
In conclusion, optimizing test suite setup with JUnit 5 facilitates efficient and reliable testing processes for Java developers. By embracing the advanced features and best practices, test suites can be tailored for greater efficiency, while maintaining flexibility and adaptability.