The Problem of Mental Overload
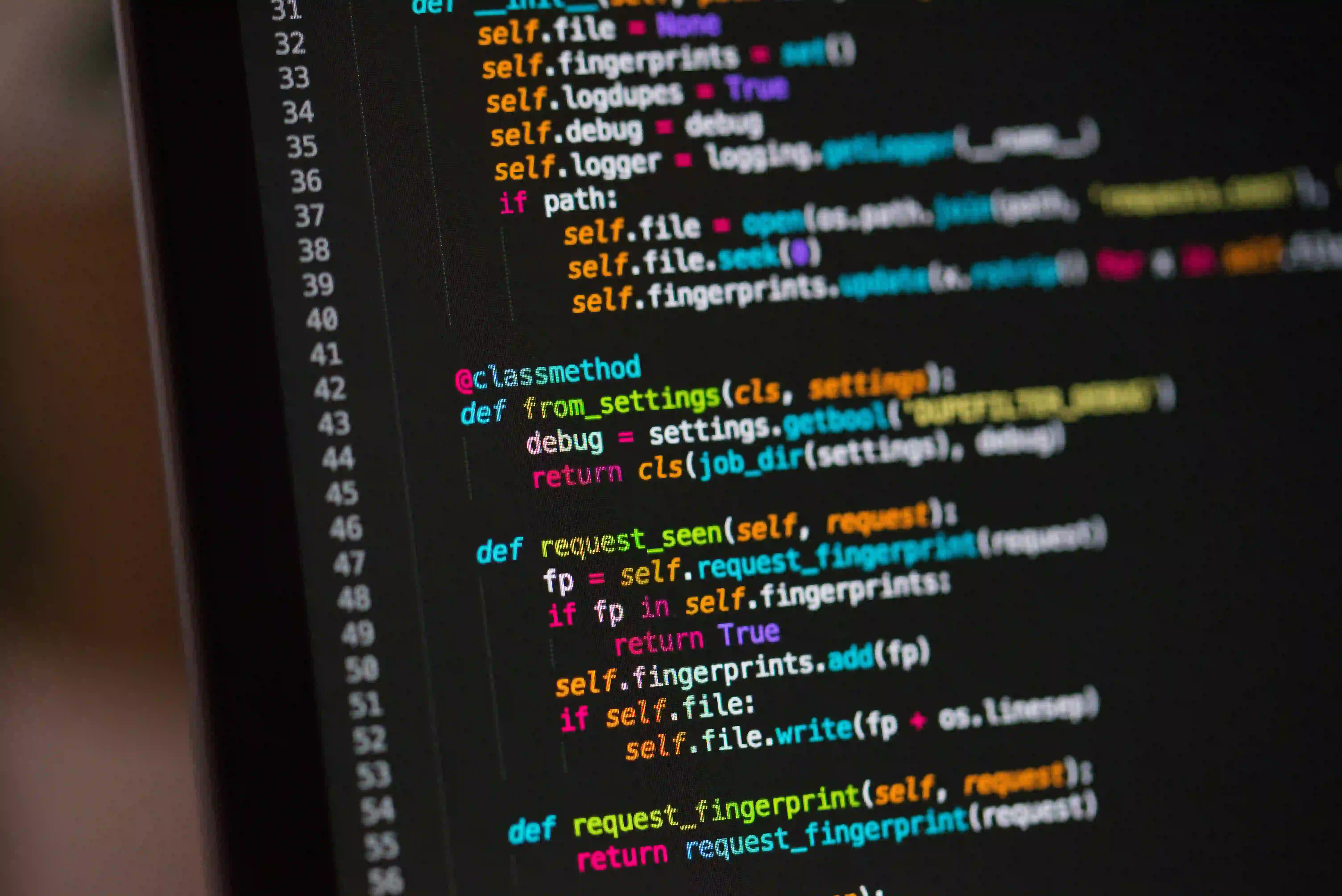
The Problem of Mental Overload
In the fast-paced world of software development, the need for efficient and manageable code has become increasingly important. As a Java developer, it is crucial to ensure that your code is not only functional but also maintainable and easy to understand. However, with the complex nature of Java applications, developers often find themselves facing the problem of mental overload. This occurs when the cognitive burden of understanding and navigating code becomes overwhelming, leading to decreased productivity and increased potential for errors.
Understanding the Impact of Mental Overload
Mental overload in Java development can manifest in several ways. It can be the result of overly complex code structures, convoluted logic, or a lack of clear documentation. When developers are faced with code that is difficult to comprehend, they spend more time deciphering the code than actually making meaningful contributions. This not only hampers their productivity but also leads to frustration and burnout.
The Role of Clean and Maintainable Code
Writing clean, maintainable code is essential to combat mental overload. By adhering to best practices and design principles, such as encapsulation, abstraction, and adherence to SOLID principles, developers can significantly reduce the cognitive load required to understand the codebase. This, in turn, enables better focus, improved productivity, and a lower probability of introducing bugs.
Encapsulation Example
public class EncapsulationExample {
private String data;
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
}
The encapsulation example showcases the use of private fields and public methods to control access to the class's data. By encapsulating the 'data' field, the internal implementation details are hidden, leading to less mental overload when interacting with the class.
Embracing Simplicity in Code Design
Complex solutions to problems often lead to convoluted code that is hard to comprehend. Embracing simplicity in code design, where each component has a clear and focused purpose, can greatly alleviate mental overload. This involves breaking down large and intricate tasks into smaller, manageable units, each responsible for a specific functionality.
Simple Design Example
public class SimpleCalculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
The 'SimpleCalculator' class demonstrates a straightforward design with methods dedicated to specific arithmetic operations. This clear and focused approach reduces mental overhead by providing a concise and understandable interface.
The Importance of Documentation
Comprehensive and clear documentation plays a pivotal role in mitigating mental overload. Well-documented code serves as a guide for developers, offering insights into the purpose and usage of various components. In Java development, documentation tools such as Javadoc provide a structured and standardized approach to documenting code, enhancing its comprehensibility.
Leveraging Effective Naming Conventions
Naming conventions are a fundamental aspect of writing understandable code. Using self-explanatory and consistent names for variables, methods, and classes can significantly reduce the cognitive effort required to grasp the code's functionality. By following widely-accepted naming conventions, such as those outlined in the Java Code Conventions, developers can contribute to a more uniform and understandable codebase.
Overcoming Mental Overload with Refactoring
Refactoring, the process of restructuring existing code without changing its external behavior, is a powerful tool for combating mental overload. By continuously improving the code's structure, eliminating redundancy, and enhancing clarity, developers can gradually reduce cognitive burdens and make the codebase more approachable.
A Final Look
In the realm of Java development, combating mental overload is an essential pursuit. By prioritizing clean and maintainable code, embracing simplicity in design, leveraging documentation, adhering to effective naming conventions, and embracing the continuous process of refactoring, developers can alleviate mental overload and foster a more efficient and enjoyable coding experience.
As you delve into the intricate world of Java development, keep in mind that the clarity and maintainability of your code are just as vital as its functionality. Strive to reduce mental overload, and you will elevate not only the quality of your code but also your overall productivity and satisfaction as a developer.
Now, it's time to put these principles into practice and embark on your journey towards crafting more elegant and comprehensible Java code. Happy coding!
Learn more about clean code principles
Explore Java best practices