Navigating the Challenges of Defining Bounded Contexts
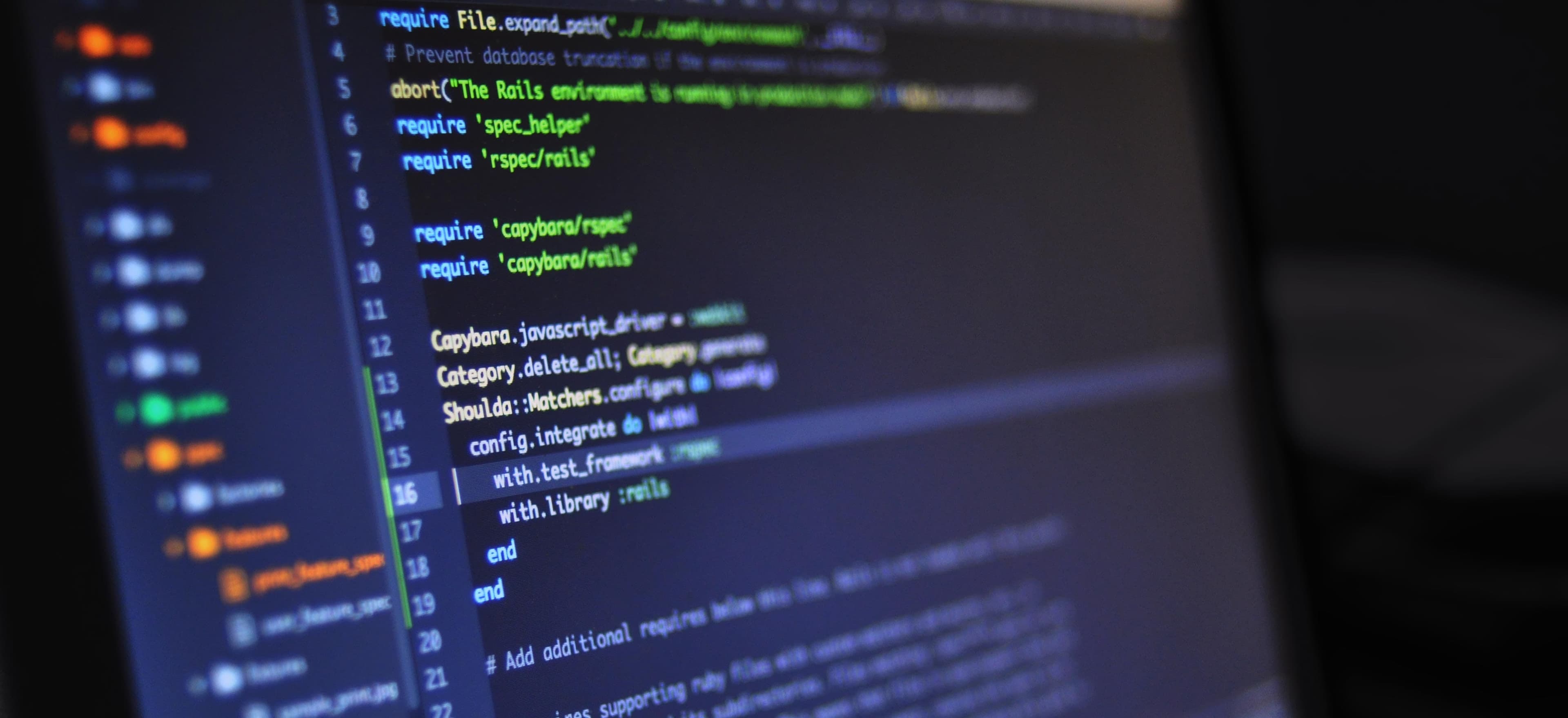
- Published on
Navigating the Challenges of Defining Bounded Contexts
In the realm of software development, particularly within the paradigms of Domain-Driven Design (DDD), the concept of bounded contexts plays a pivotal role. Bounded contexts help teams to delineate systems clearly, making it easier to manage communication and interaction between various components. However, defining these bounded contexts can be fraught with challenges. In this blog post, we will explore those challenges and provide practical strategies for effectively defining bounded contexts.
What is a Bounded Context?
To kick things off, a bounded context refers to a conceptual boundary within which a particular model is defined and applicable. Essentially, it sets the linguistic and functional scope of a particular domain. As a domain grows, different models may need to be created to accommodate various sub-domains, making it vital to clearly define each bounded context.
For example, in an e-commerce platform, you might have distinct bounded contexts for:
- Product Management: Handling product catalogs, descriptions, and pricing.
- Order Processing: Managing orders, payment processing, and shipment tracking.
- Customer Relationship Management: Focusing on customer interactions and data.
The Challenges of Defining Bounded Contexts
Defining bounded contexts involves more than just drawing boxes around different functionalities. It requires deep domain knowledge, clear communication, and active participation from multiple stakeholders. Here are some common challenges encountered:
1. Overlapping Domains
In many cases, domains do not operate in isolation. The Product Management context might touch upon service-level data from the Order Processing domain, potentially leading to tension and confusion in responsibilities.
To navigate this, teams should:
- Engage Stakeholders: Regularly meet with stakeholders from various domains to clarify expectations.
- Use Context Mapping: Create visual representations that delineate how different contexts interact. Learn more about context mapping here.
2. Language & Communication Barriers
Each sub-domain often develops its own terminology. When writing code or discussing the system's architecture, you may encounter misunderstandings. For instance, what one team calls "product," another might refer to as "inventory item."
To alleviate confusion:
- Establish Ubiquitous Language: Ensure that terms are consistently used across all teams. Consider creating a shared glossary.
- Regular Workshops: Hosting terminology workshops can foster understanding and collaboration.
3. Changing Business Needs
The growth and evolution of a business can lead to shifting requirements and new opportunities, making initial bounded contexts inadequate. For example, as an e-commerce platform scales, new services or regions may necessitate the re-evaluation of existing contexts.
To stay adaptable:
- Frequent Reviews: Conduct regular evaluations of bounded contexts and the models they encompass.
- Feedback Loops: Establish feedback mechanisms from all teams to enable continual improvement.
Strategies to Define Bounded Contexts
Having established the challenges, let's delve into some effective strategies for defining and managing bounded contexts.
1. Identify Core Domain and Subdomains
Starting with a clear identification of your core domain helps in differentiating subdomains. Employ strategic analysis tools like event storming or domain storytelling to unravel the intricacies.
Example Code Implementation for Domain Modeling
Consider the foundational domain class for a product:
public class Product {
private String id;
private String name;
private double price;
// Product constructor
public Product(String id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
// Getters for properties
public String getId() { return id; }
public String getName() { return name; }
public double getPrice() { return price; }
// Business logic example
public void applyDiscount(double percentage) {
if (percentage > 0 && percentage < 100) {
this.price -= this.price * (percentage / 100);
}
}
}
The above class lays a good foundation for the Product Management context. It encapsulates the primary attributes and a bit of business logic, setting the stage for further development.
2. Solidify Context Boundaries with Microservices
Employing microservices architecture is a practical way to implement bounded contexts. Each context can become its own service with well-defined APIs.
Example Microservice Class
@RestController
@RequestMapping("/products")
public class ProductService {
// A service for managing products
private final ProductRepository productRepository;
@Autowired
public ProductService(ProductRepository productRepository) {
this.productRepository = productRepository;
}
@PostMapping("/")
public ResponseEntity<Product> createProduct(@RequestBody Product product) {
Product createdProduct = productRepository.save(product);
return ResponseEntity.status(HttpStatus.CREATED).body(createdProduct);
}
}
This example showcases a REST controller for the product microservice. It handles the product creation functionality, encapsulated within its bounded context.
3. Utilize Domain Events
Domain events act as notification signals among bounded contexts, promoting loose coupling and better integration.
Example Domain Event
public class ProductCreatedEvent {
private final Product product;
public ProductCreatedEvent(Product product) {
this.product = product;
}
public Product getProduct() { return product; }
}
This Java class represents a product creation event. It can be dispatched to notify other contexts, like the Order Processing context.
4. Continuous Refactoring
With the inevitability of change in any system, continuous refactoring is essential. This includes revisiting the relationships and interfaces of existing contexts.
- Adopt Agile Methodologies: Embrace practices such as Scrum or Kanban, allowing for iterative improvements.
- Technical Debt Awareness: Regularly assess the state of the code and make the necessary hash-ups without fear of breaking changes.
The Bottom Line
Defining bounded contexts is not merely a one-time exercise but an ongoing effort that requires care, attention, and adaptability. As systems evolve, so must the definitions and boundaries of contexts. Engage your stakeholders, encourage a culture of communication, and iteratively refine your models.
By understanding the inherent challenges and implementing practical strategies, you can successfully navigate the complexities associated with bounded contexts in your software architecture. For more insights on this topic, check out Domain-Driven Design Distilled by Eric Evans, a classic guide in mastering DDD concepts.
Stay connected guys, and let’s embrace the art of software development together!