Lagging Database Performance with Jooq and Scala Futures
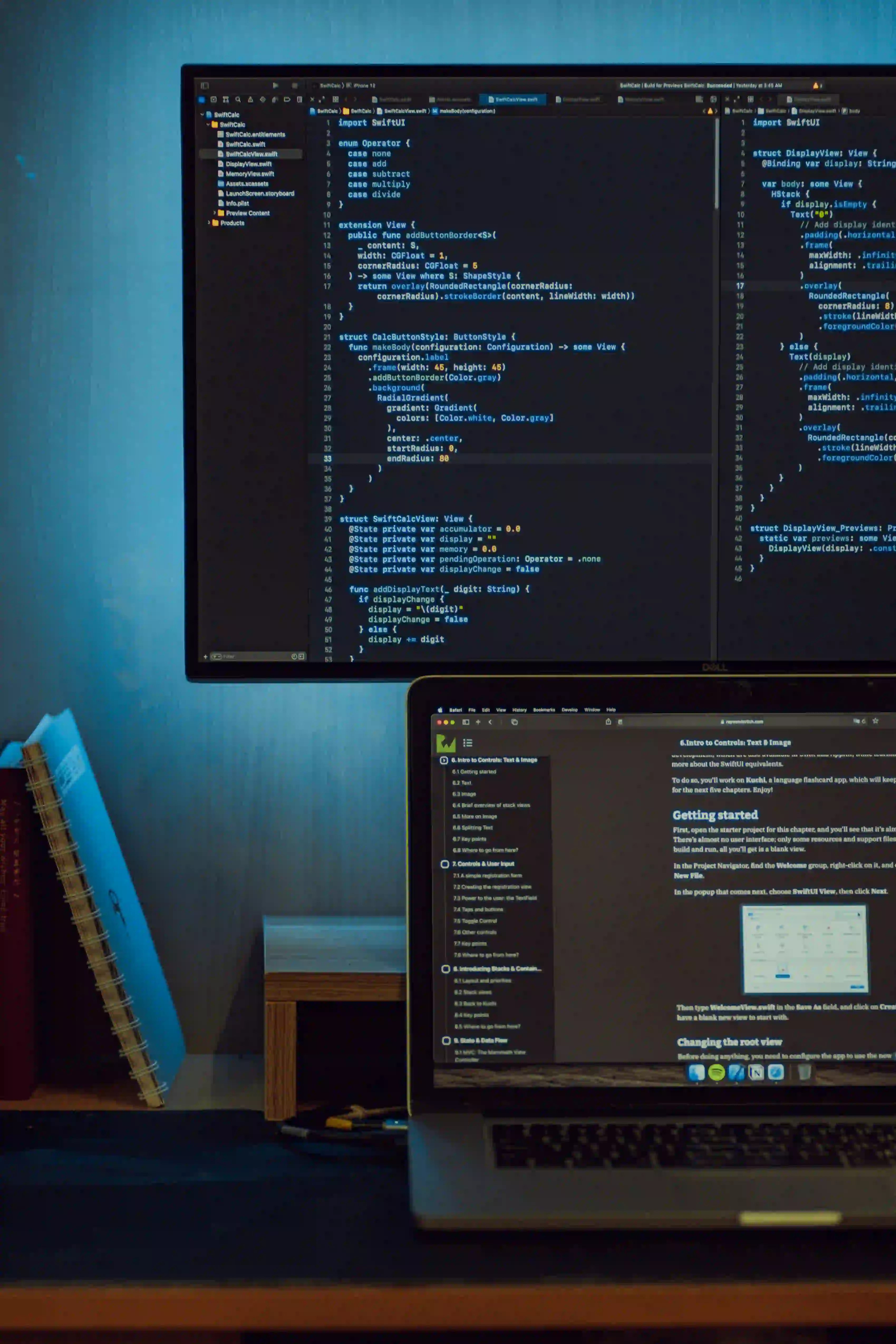
Boosting Database Performance with Jooq and Scala Futures
In today's fast-paced digital world, optimizing database performance is critical for ensuring seamless user experiences. When working with Java applications and databases, utilizing tools like Jooq and Scala Futures can significantly enhance performance and responsiveness.
Understanding the Challenge
Many Java applications interact with databases to fetch, update, and manipulate data. However, as the complexity of these applications grows, the performance of database interactions can suffer. Long-running queries, inefficient data retrieval, and blocking operations can all contribute to sluggish performance and hinder the overall user experience.
Introducing Jooq and Scala Futures
Jooq is a Java library that provides a fluent API for building type-safe SQL queries. It enables developers to interact with databases using a more expressive and intuitive approach, resulting in cleaner and more maintainable code.
On the other hand, Scala Futures offer a powerful tool for writing asynchronous, non-blocking code in Scala. By utilizing Futures, developers can execute database operations concurrently, thereby improving the application's responsiveness.
The Lagging Performance Issue
Consider a scenario where a Java application, powered by Jooq, experiences lagging performance when executing database queries. This becomes particularly noticeable when dealing with large datasets or when multiple queries need to be executed in parallel.
// Example Jooq query execution
ResultQuery<Record> query = context.select().from(BOOK).where(BOOK.ID.eq(123));
Result<Record> result = query.fetch();
In this traditional synchronous approach, the application waits for the query to complete before proceeding further. If there are multiple such queries or if the database is slow to respond, it can lead to a considerable impact on the overall performance of the application.
Leveraging Scala Futures for Asynchronous Database Operations
To address the performance issue, we can leverage Scala Futures to execute database operations asynchronously. By doing so, the application can initiate multiple database calls concurrently and continue with other tasks while waiting for the results.
// Asynchronous execution of Jooq query using Scala Futures
import scala.concurrent.ExecutionContext.Implicits.global
import scala.concurrent.Future
val futureResult: Future[Result[Record]] = Future {
context.select().from(BOOK).where(BOOK.ID.eq(123)).fetch()
}
In this snippet, the Jooq query is wrapped inside a Future
block, allowing it to run asynchronously. Once the query is dispatched as a Future, the application can continue its execution, unblocked by the database operation.
Handling Future Results
As the database operation is now running in a separate thread, we can define actions to be taken upon the completion of the Future. This can be achieved using Scala's powerful combinators like map
, flatMap
, and onComplete
.
// Handling Future results using combinators
val processedResult: Future[ProcessedData] = futureResult.map { result =>
// Process the fetched data or perform additional operations
processData(result)
}
By utilizing combinators, we can seamlessly chain asynchronous operations, transforming and composing the results without sacrificing performance.
Ensuring Thread Safety with Jooq
While leveraging Scala Futures for asynchronous database operations, it's crucial to ensure thread safety, especially when interacting with Jooq's DSLContext
. Fortunately, Jooq provides built-in support for thread safety through its DSLContext
instances.
// Thread-safe DSLContext usage with Jooq
DSLContext threadSafeContext = DSL.using(dataSource, SQLDialect.POSTGRES);
By creating a thread-safe DSLContext
using a connection pool or DataSource, we can safely share the context across multiple threads without compromising data integrity or performance.
Managing Database Connection Pooling
Efficient database connection management is essential for optimizing performance. By using a robust connection pooling mechanism, such as HikariCP, we can ensure that database connections are utilized effectively and efficiently, minimizing overhead and latency.
// Configuring HikariCP connection pool
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:postgresql://localhost:5432/mydatabase");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(10);
DataSource dataSource = new HikariDataSource(config);
With HikariCP, we can fine-tune the connection pool settings to align with the application's specific requirements, thereby enhancing database interaction performance.
Mitigating Latency with Asynchronous Calls
In scenarios where an application needs to fetch data from multiple database tables in parallel, the use of Scala Futures for concurrent querying proves to be invaluable. By initiating independent database calls using Futures, the application can mitigate latency and ensure timely data retrieval.
// Executing multiple Jooq queries concurrently using Scala Futures
val futureResult1: Future[Result[Record]] = Future {
context.select().from(TABLE1).fetch()
}
val futureResult2: Future[Result[Record]] = Future {
context.select().from(TABLE2).fetch()
}
val combinedResults: Future[(Result[Record], Result[Record])] = futureResult1.flatMap { result1 =>
futureResult2.map { result2 =>
(result1, result2)
}
}
By combining the results of multiple Futures using combinators like flatMap
and map
, we can effectively handle and process data from parallel database operations, resulting in improved performance and responsiveness.
Lessons Learned
By integrating Jooq and Scala Futures into Java applications, developers can significantly enhance database interaction performance. Leveraging Jooq's expressive API and Scala's asynchronous capabilities, applications can execute database operations efficiently, mitigate latency, and deliver an optimal user experience.
Optimizing database performance using Jooq and Scala Futures demonstrates the intersection of powerful libraries and paradigms within the Java ecosystem, empowering developers to build high-performance, responsive applications.