Optimizing JDBC Connections for Better Application Performance
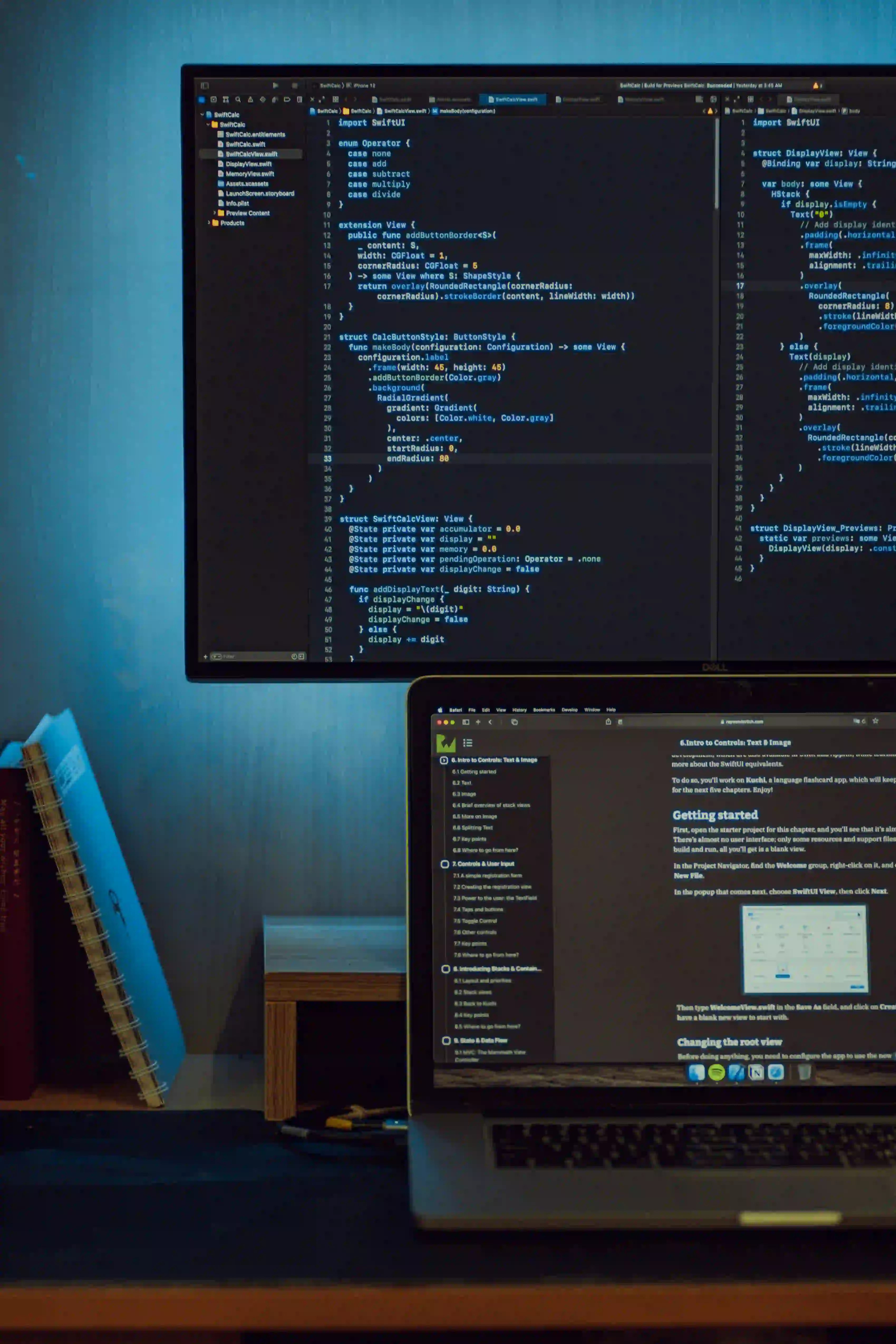
Optimizing JDBC Connections for Better Application Performance
In the world of Java development, the use of JDBC (Java Database Connectivity) is almost inevitable when it comes to interacting with databases. However, inefficient use of JDBC connections can often lead to performance bottlenecks in your application. In this post, we will delve into the best practices and techniques for optimizing JDBC connections, ultimately enhancing the overall performance of your Java application.
Understanding the Importance of Optimized JDBC Connections
JDBC connections serve as the lifeline between your application and the database. Inefficient handling of these connections can lead to issues such as connection leaks, unnecessary overhead, and decreased application performance. Therefore, it is imperative to optimize the usage of JDBC connections to ensure smooth and efficient operation of your application.
Using Connection Pooling
One of the fundamental techniques for optimizing JDBC connections is the use of connection pooling. Instead of creating a new connection every time a database interaction is required, connection pooling allows reusing existing connections, thereby reducing the overhead of creating and tearing down connections.
Let's take a look at a simple example of using the HikariCP connection pool, a widely-used and highly efficient connection pooling library for Java applications:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
import java.sql.Connection;
import java.sql.SQLException;
public class ConnectionPoolExample {
private static final HikariDataSource dataSource;
static {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("username");
config.setPassword("password");
dataSource = new HikariDataSource(config);
}
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
// Other methods for releasing connections, configuration, etc.
}
In this example, we configure and initialize the HikariCP data source, and then use it to obtain connections when needed. The pooling library takes care of managing and recycling the connections, leading to improved performance and resource utilization.
Proper Connection Handling and Resource Cleanup
In Java, it's crucial to handle resources, such as JDBC connections, in a proper and efficient manner. Failing to close connections after their use can lead to resource leaks and potential performance degradation. The try-with-resources statement in Java can be utilized for automatic resource management and cleanup.
try (Connection connection = ConnectionPoolExample.getConnection();
PreparedStatement statement = connection.prepareStatement("SELECT * FROM users")) {
// Execute query, process results, etc.
} catch (SQLException e) {
// Handle exceptions
}
By encapsulating the JDBC resources within the try-with-resources block, Java ensures that the resources are closed after the block is executed, regardless of whether an exception occurs. This approach minimizes the risk of resource leaks and contributes to better resource utilization and performance.
Efficient Statement and ResultSet Handling
Another area where optimization can significantly impact JDBC performance is the handling of statements and result sets. Reusing prepared statements, using batch updates for multiple queries, and properly closing result sets after processing are essential practices for efficient JDBC operations.
try (Connection connection = ConnectionPoolExample.getConnection();
PreparedStatement statement = connection.prepareStatement("INSERT INTO users (name, age) VALUES (?, ?)")) {
for (User user : userList) {
statement.setString(1, user.getName());
statement.setInt(2, user.getAge());
statement.addBatch();
}
statement.executeBatch();
} catch (SQLException e) {
// Handle exceptions
}
In this example, we utilize batch updates to efficiently insert multiple user records into the database using a single prepared statement, thus reducing the overhead of multiple individual queries.
Tuning Connection Parameters and Database Configuration
Optimizing JDBC connections also involves fine-tuning various connection parameters and database configurations. Adjusting settings such as connection timeouts, maximum connection pool size, and fetch size can have a significant impact on the performance of database interactions.
Moreover, optimizing the database itself by creating indexes, minimizing expensive query operations, and using database-specific performance optimization techniques can contribute to improved overall performance of JDBC operations.
My Closing Thoughts on the Matter
In conclusion, optimizing JDBC connections is essential for ensuring the efficient performance of Java applications that interact with databases. Leveraging connection pooling, proper resource handling, efficient statement and result set management, and tuning connection parameters and database configurations are crucial steps toward achieving optimal JDBC performance.
By adhering to these best practices and techniques, you can mitigate the potential bottlenecks associated with JDBC connections and significantly improve the overall performance and scalability of your Java applications.
To further explore the realm of JDBC and database optimization, delve into Oracle's official JDBC documentation and HikariCP's GitHub repository for comprehensive insights and advanced features.
Remember, the efficiency of JDBC connections is not merely an option but a necessity for maintaining a robust and high-performing Java application.