Solving Common Android Interview Questions
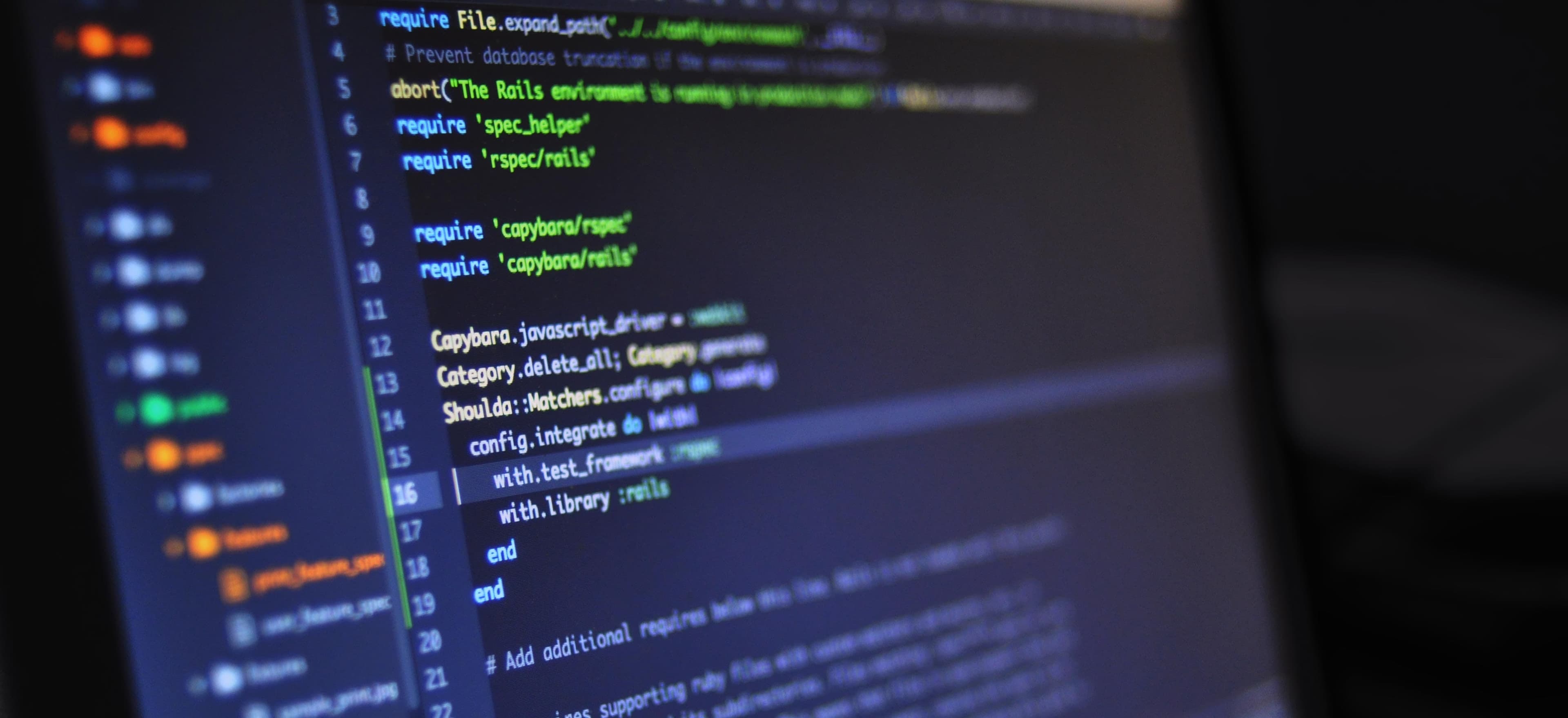
- Published on
Solving Common Android Interview Questions
Are you preparing for an Android developer interview? Are you ready to nail those tricky technical questions? This blog post will equip you with the skills to tackle some of the most common Android interview questions. Let’s dive in and explore the solutions to these questions.
Question 1: Explain the Activity Lifecycle in Android
The Activity in Android goes through various states during its lifetime. The understanding of this lifecycle is crucial for developing robust Android applications. Here’s a brief overview:
- onCreate(): This is where the activity is initialized.
- onStart(): The activity becomes visible to the user.
- onResume(): The activity is brought to the foreground and becomes interactive.
- onPause(): Another activity comes to the foreground, putting the current activity in the paused state.
- onStop(): The activity is no longer visible to the user.
- onDestroy(): The activity is being destroyed.
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
protected void onStart() {
super.onStart();
// Add your code here
}
@Override
protected void onResume() {
super.onResume();
// Add your code here
}
@Override
protected void onPause() {
// Add your code here
super.onPause();
}
@Override
protected void onStop() {
// Add your code here
super.onStop();
}
@Override
protected void onDestroy() {
// Add your code here
super.onDestroy();
}
When answering this question in an interview, it’s critical to explain the significance of each state and how an Android developer can leverage them to create a seamless user experience.
Question 2: What is ADB in Android?
ADB (Android Debug Bridge) is a versatile command-line tool that lets developers communicate with a device. It facilitates a variety of device actions, such as installing and debugging apps, and provides access to a Unix shell that you can use to run a variety of commands on a device.
Developers can use ADB to push and pull files, install and uninstall apps, capture screenshots, and more.
Here's an example of using ADB to install an APK on a device:
adb install app.apk
When answering this question, it’s important to mention the practical uses of ADB and its significance in Android application development and debugging.
Question 3: Describe the Difference Between Serializable and Parcelable
When it comes to passing data between Android components, such as activities or fragments, the Serializable and Parcelable interfaces come into play.
The Serializable interface uses standard Java serialization to serialize an object. While it's easy to implement, it is a relatively slow process and can affect performance when dealing with large amounts of data.
On the other hand, the Parcelable interface is a faster and preferred way of passing data between components. It requires a bit more effort to implement, but it offers better performance, especially when dealing with large datasets.
When discussing this in an interview, it’s crucial to explain when to use Serializable and Parcelable, and justify the use of Parcelable for better performance.
Question 4: Explain the Role of Content Providers in Android
Content Providers offer a structured and secure way of managing data. They allow different applications to access the same data across multiple processes, ensuring data security and integrity.
Content Providers are often used to share data with other applications, query and manage a large dataset, and provide data to widgets using a consistent API.
Here is an example of accessing the contacts using a Content Provider:
Cursor cursor = getContentResolver().query(Contacts.CONTENT_URI, null, null, null, null);
When elaborating on Content Providers, it’s important to emphasize their role in data sharing and the security they provide when accessing data across applications.
Question 5: What are the Advantages of Using Kotlin for Android Development?
Kotlin, a statically typed programming language, has gained immense popularity in the Android development community due to its concise syntax, null safety, and interoperability with Java.
Advantages of using Kotlin for Android development include reduced boilerplate code, improved code readability, enhanced developer productivity, and seamless integration with existing Java code.
Mention the advantages of Kotlin in terms of writing cleaner, more concise and maintainable code to effectively answer this question.
By understanding and practicing the solutions to these common Android interview questions, you will be better prepared to demonstrate your expertise and proficiency in Android development. Keep in mind to not just memorize the answers, but to truly understand the concepts behind them. Good luck with your interview preparation!
Remember, as an Android developer, continuous learning and staying updated with the latest trends and developments in the Android ecosystem can greatly benefit your career.
If you're looking to further polish your Android developer skills, consider checking out this comprehensive Android Developer Guide.
Happy coding!
Checkout our other articles