Maximizing Performance of Dockerized Spring Boot Application
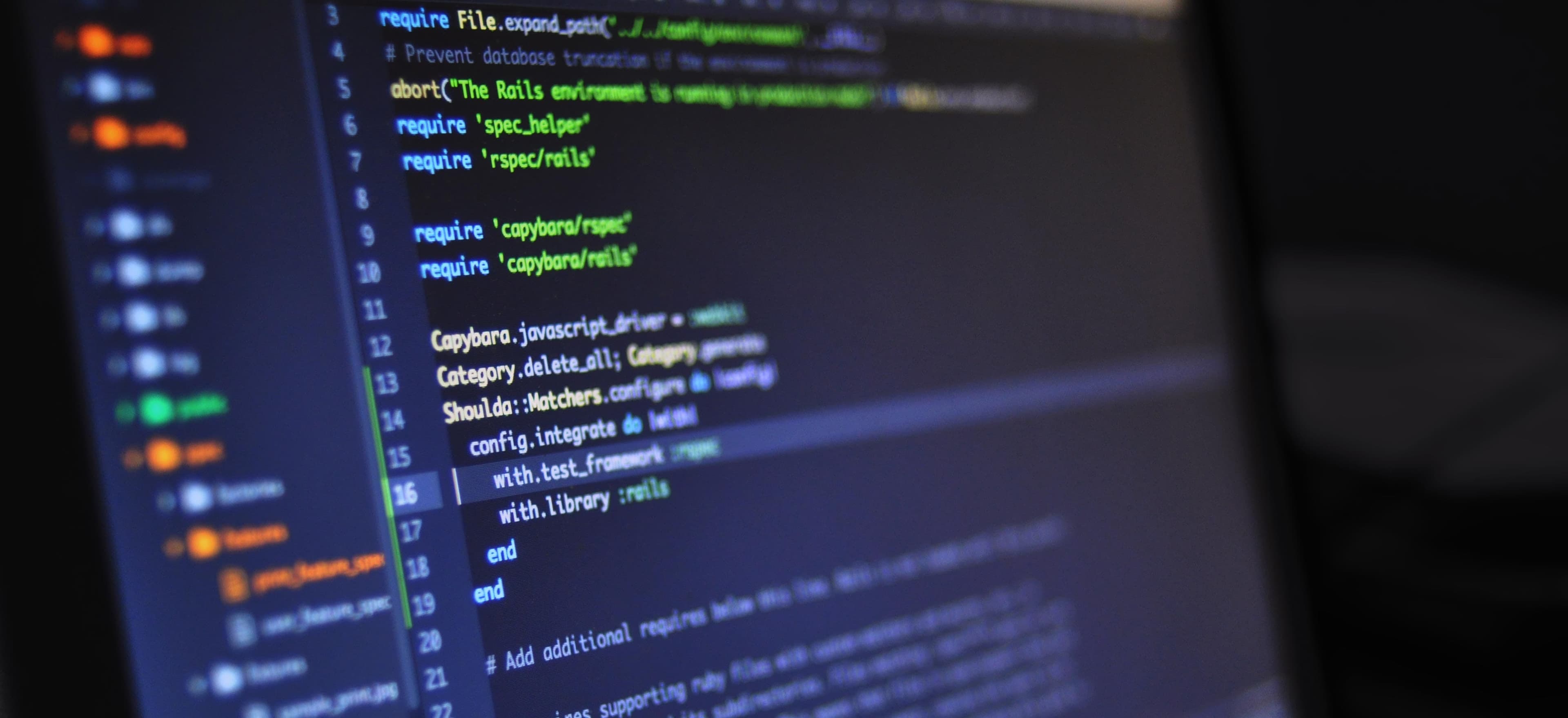
- Published on
Maximizing Performance of Dockerized Spring Boot Application
In today's fast-paced world of software development, performance optimization plays a critical role in ensuring the success of an application. When it comes to deploying Java applications, Docker has gained immense popularity due to its ability to streamline the deployment process and facilitate consistent environments across different platforms. However, optimizing the performance of a Dockerized Spring Boot application requires a strategic approach to leverage the full potential of both Spring Boot and Docker.
In this article, we will explore best practices for maximizing the performance of a Dockerized Spring Boot application. We will delve into various optimization techniques, including image size reduction, JVM memory tuning, and container orchestration, to ensure that your Spring Boot application running in a Docker container delivers exceptional performance.
Optimizing Docker Image Size
Choosing a Lean Base Image
Choosing a lean base image is crucial in minimizing the overall size of your Docker image. Instead of using a generic base image like Ubuntu or CentOS, opt for specialized base images tailored for running Java applications. Alpine Linux, for instance, provides a minimalistic base that significantly reduces the size of the final Docker image.
Multi-stage Builds
Leverage multi-stage Docker builds to separate the build environment from the runtime environment. This approach allows you to build your application using tools like Maven or Gradle in one stage and then copy the compiled artifacts to a clean base image in another stage. This effectively reduces the size of the final image, as it only contains the runtime dependencies necessary for the Spring Boot application.
# Multi-stage Dockerfile
# Build stage
FROM maven:3.6.3-openjdk-11 AS build
WORKDIR /app
COPY pom.xml .
RUN mvn dependency:go-offline
COPY src/ /app/src/
RUN mvn package
# Runtime stage
FROM adoptopenjdk:11-jre-hotspot
WORKDIR /app
COPY --from=build /app/target/app.jar /app/app.jar
CMD ["java", "-jar", "app.jar"]
Remove Unnecessary Dependencies
When creating the final Docker image, ensure that you exclude any unnecessary files, dependencies, or build artifacts. This can be achieved by carefully configuring the .dockerignore
file to prevent unnecessary files from being copied into the image.
JVM Memory Tuning
Configure JVM Heap Size
Optimizing the JVM heap size is paramount for maximizing the performance of a Spring Boot application. By default, the JVM allocates a conservative amount of memory, which may not be optimal for your specific application requirements. To tune the JVM heap size, you can use the -Xms
and -Xmx
flags to set the initial and maximum heap sizes, respectively.
java -Xms512m -Xmx1024m -jar app.jar
Garbage Collection Tuning
Fine-tuning the garbage collection settings can have a significant impact on the overall performance of the JVM. You can choose the appropriate garbage collector based on your application's characteristics and workload. For example, the G1 garbage collector is well-suited for applications with large heaps and aims to provide consistent pause times.
java -XX:+UseG1GC -jar app.jar
Container Orchestration
Kubernetes Deployment
If you are managing a cluster of Dockerized Spring Boot applications, consider using Kubernetes for container orchestration. Kubernetes provides features such as horizontal scaling, self-healing, and load balancing, which are essential for maximizing the performance and resilience of your applications.
apiVersion: apps/v1
kind: Deployment
metadata:
name: spring-boot-app
spec:
replicas: 3
selector:
matchLabels:
app: spring-boot-app
template:
metadata:
labels:
app: spring-boot-app
spec:
containers:
- name: spring-boot-app
image: your-registry/spring-boot-app:latest
ports:
- containerPort: 8080
Utilize Container Health Probes
In Kubernetes, you can define liveness and readiness probes to monitor the health of your Spring Boot application running in a Docker container. Liveness probes ensure that the application is responsive, while readiness probes indicate when the application is ready to serve traffic. By utilizing these probes, Kubernetes can make informed decisions about routing traffic to healthy instances, thus improving the overall performance and reliability of your application.
livenessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 10
periodSeconds: 30
readinessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 15
periodSeconds: 5
Closing the Chapter
Optimizing the performance of a Dockerized Spring Boot application involves a holistic approach that encompasses the Docker image size, JVM memory tuning, and container orchestration. By following best practices such as choosing a lean base image, fine-tuning JVM memory settings, and leveraging container orchestration platforms like Kubernetes, you can ensure that your Spring Boot application delivers exceptional performance in a Dockerized environment.
By implementing these optimization techniques, you can maximize the efficiency, scalability, and resilience of your Dockerized Spring Boot applications, ultimately providing a superior experience for both users and administrators.
Remember, performance optimization is not a one-time task but an ongoing process. Continuously monitor and fine-tune your Dockerized Spring Boot applications to adapt to changing workloads and evolving best practices in the industry. Stay proactive, stay optimized!