Understanding Immutability in Functional Java
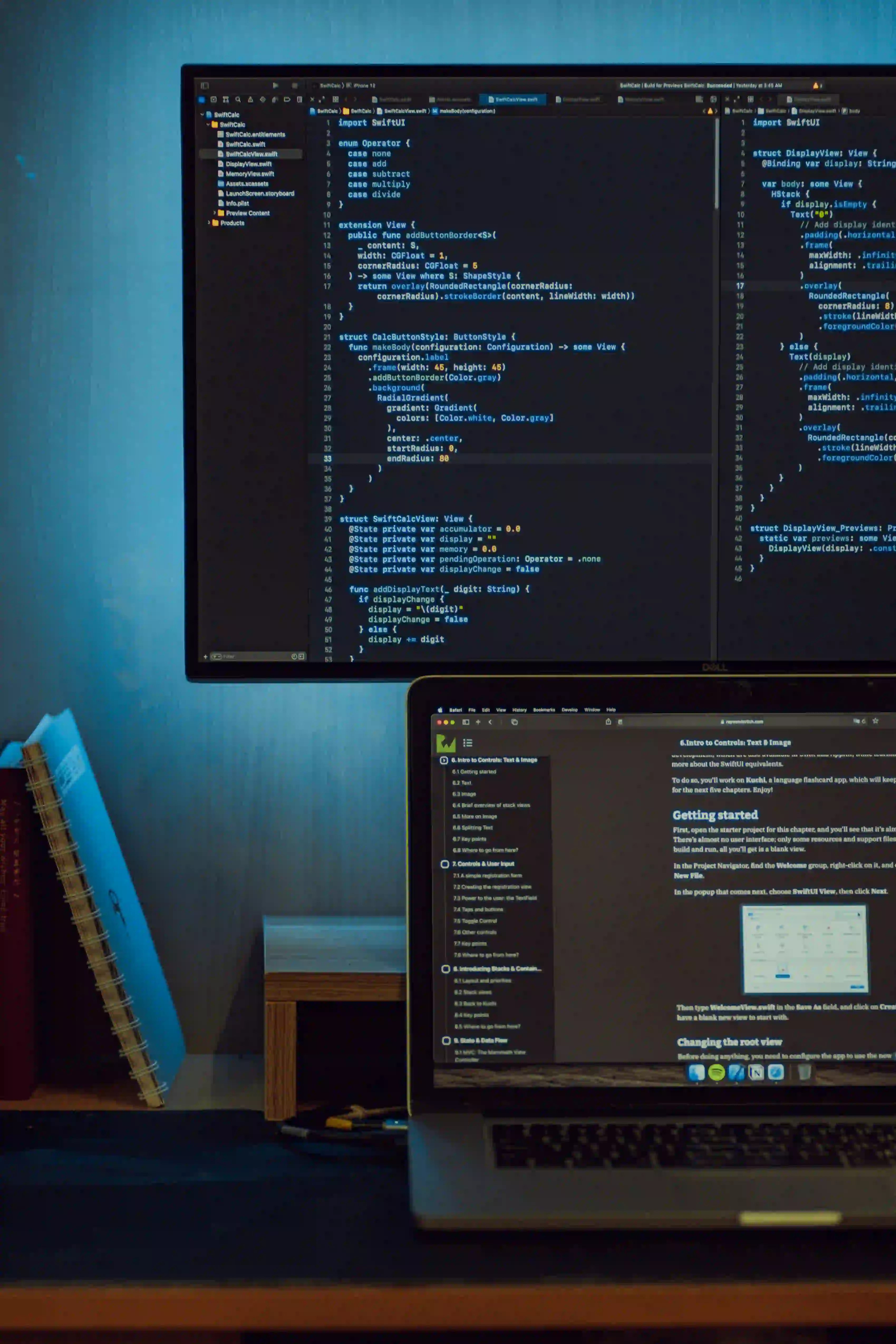
Understanding Immutability in Functional Java
Immutability is a fundamental concept in functional programming and plays a crucial role in Java. In this article, we'll delve into the concept of immutability in Java, its importance, and how to achieve it effectively.
What is Immutability?
In simple terms, an object is immutable if its state cannot be modified after it is created. Once the value is set, it cannot be changed. Immutable objects are inherently thread-safe and can be shared without the need for synchronization. In a multi-threaded environment, immutability ensures that the object remains consistent and cannot be altered by other threads.
Importance of Immutability
Thread Safety
Immutable objects are inherently thread-safe as their state cannot be modified. This eliminates the need for synchronization, reducing the risk of concurrency issues such as race conditions and deadlocks.
Concurrent Programming
In concurrent programming, immutable objects simplify the overall design and make it easier to reason about the code. They facilitate the creation of thread-safe data structures without the complexity of locks or other synchronization mechanisms.
Parallel Processing
In a parallel processing environment, immutability allows for safe and efficient data sharing among parallel tasks. Immutable objects can be freely shared among tasks without the risk of data corruption.
Clean Code
Immutability promotes cleaner, more maintainable code. It reduces the complexity of state management and the potential for unexpected side effects, resulting in more predictable and easier-to-understand code.
Achieving Immutability in Java
Final Keyword
In Java, you can use the final
keyword to create immutable fields. Once initialized, the value of a final
field cannot be changed. For example:
public class ImmutableObject {
private final String name;
public ImmutableObject(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
The name
field in the ImmutableObject
class is immutable, ensuring that its value remains constant after the object is constructed.
Immutable Classes
To create an immutable class in Java, follow these guidelines:
- Make the class
final
to prevent inheritance. - Declare all fields as
private
andfinal
. - Do not provide setter methods. Instead, initialize the fields through the constructor.
- If the class contains mutable objects (e.g., collections), ensure they are defensively copied to prevent external modification.
Here's an example of an immutable class in Java:
public final class ImmutablePerson {
private final String name;
private final int age;
public ImmutablePerson(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
The ImmutablePerson
class is immutable, as its fields are final
and cannot be modified after instantiation.
Immutable Collections
The Java Collections Framework provides immutable collection implementations through the Collections
utility class. These implementations return unmodifiable views of the underlying collections, ensuring that the original collection remains unchanged.
For example, to create an immutable list:
List<String> mutableList = new ArrayList<>();
mutableList.add("Java");
mutableList.add("is");
mutableList.add("awesome");
List<String> immutableList = Collections.unmodifiableList(mutableList);
The immutableList
is an unmodifiable view of mutableList
, preventing any changes to the original collection.
Immutable Libraries
Several third-party libraries, such as Guava and Apache Commons, offer immutable data structures and utility classes to facilitate immutability in Java. These libraries provide additional functionalities and performance optimizations for working with immutable objects.
Benefits of Immutability in Java
Safe Sharing
Immutable objects can be safely shared across threads and components without the risk of concurrent modifications. This simplifies the development of concurrent and parallel applications.
Defensive Copying
When passing immutable objects as arguments, there's no need for defensive copying since the original object cannot be modified. This eliminates the need for defensive programming against unexpected changes.
Performance Optimization
Immutable objects can be cached and reused, leading to potential performance improvements, especially in scenarios where objects are frequently used or shared.
Reliability and Predictability
Immutable objects lead to more reliable and predictable code by reducing the likelihood of unexpected state changes. This makes debugging and maintenance easier.
Best Practices for Immutability
Document Immutability
When designing classes, document their immutability explicitly. Include clear documentation to inform the users and maintainers of the class about its immutable nature.
Use Immutable Libraries
Take advantage of third-party libraries that offer comprehensive support for immutability. Libraries like Guava and Apache Commons provide a wide range of immutable data structures and utility classes.
Immutable by Default
Favor immutability by default when designing classes and data structures. Make mutability an explicit design choice only when necessary.
Performance Considerations
While immutability offers several benefits, it's essential to consider its impact on performance and memory usage. Evaluate the trade-offs and ensure that immutability aligns with the specific requirements of your application.
In Conclusion, Here is What Matters
Immutability is a powerful concept in Java that promotes thread safety, clean code, and reliable concurrent and parallel programming. By designing classes and data structures with immutability in mind, developers can simplify the handling of state and reduce the complexity of multi-threaded applications. Embracing immutability not only enhances the robustness of the code but also contributes to improved performance and reliability.
In summary, immutability is not merely a design principle; it's a fundamental enabler for building resilient, scalable, and maintainable Java applications.
Remember, embracing immutability is not just about following rules; it’s about cultivating a mindset that values stability, simplicity, and reliability in our code.
So, what are your thoughts on immutability in Java? Have you experienced the benefits of immutability in your projects? Let's keep the conversation going!