Customizing UserDetailsService in Spring Security
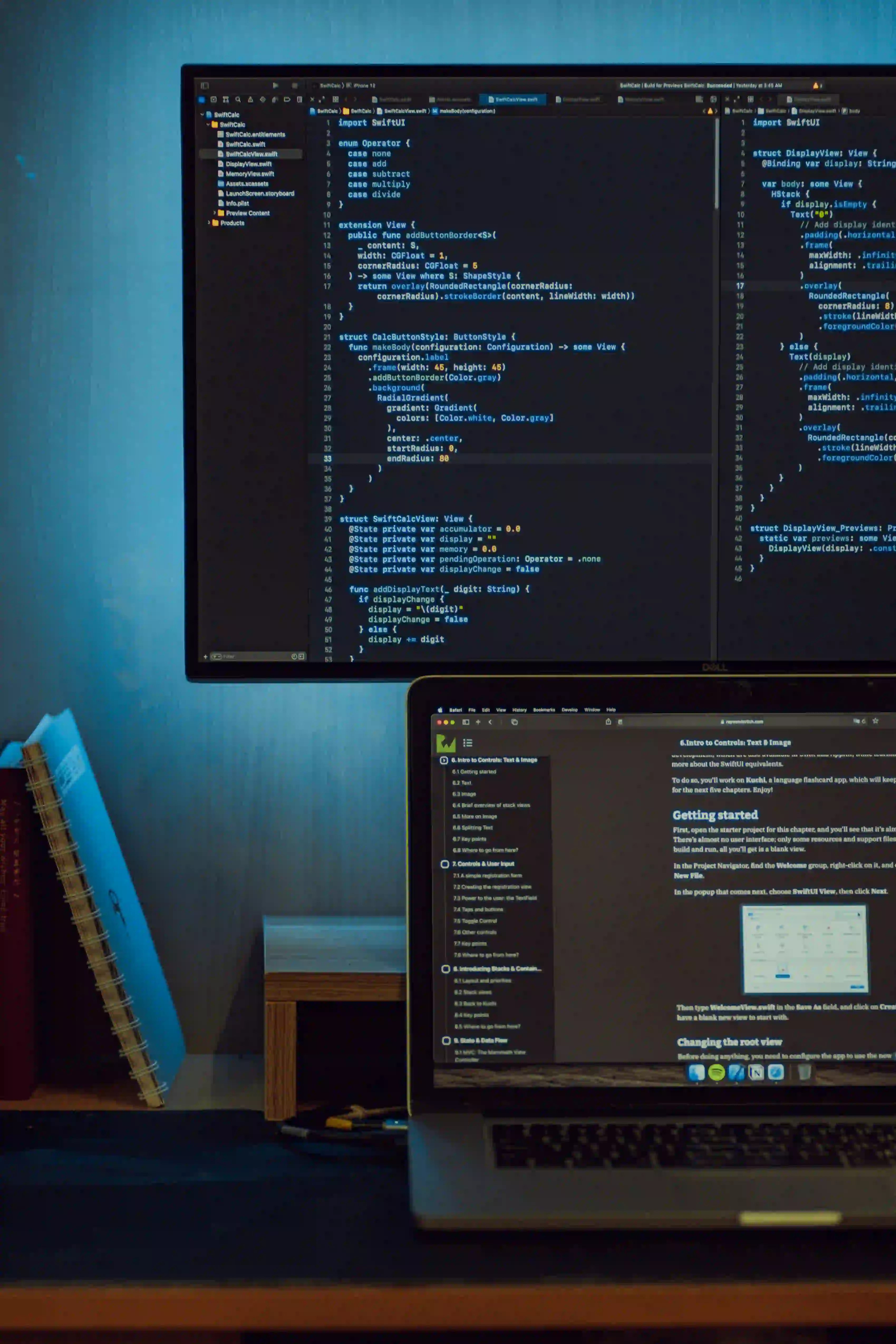
Customizing UserDetailsService in Spring Security
In any web application, security is a vital aspect that cannot be overlooked. Spring Security provides a robust framework for implementing security in Java applications, offering various features for authentication, authorization, and protection against common security threats. One of the key components of Spring Security is the UserDetailsService
, which is responsible for retrieving user information during the authentication process.
In this article, we will explore the concept of UserDetailsService
in Spring Security and demonstrate how to customize it to meet specific authentication requirements.
Understanding UserDetailsService
The UserDetailsService
interface is used to retrieve user-related data for authentication and authorization. When a user attempts to authenticate, Spring Security calls the loadUserByUsername
method of the UserDetailsService
to load user details based on the provided username. The returned UserDetails
object includes information such as the user's username, password, and granted authorities.
By default, Spring Security provides the InMemoryUserDetailsManager
and JdbcUserDetailsManager
implementations of UserDetailsService
, which are suitable for simple authentication scenarios. However, in real-world applications, user information is often stored in a custom data source such as a database, LDAP server, or external service.
Customizing UserDetailsService
To customize the UserDetailsService
in Spring Security, you can create your own implementation that retrieves user information from a custom data source. This allows you to integrate with existing user databases and external systems, providing a flexible and tailored authentication mechanism for your application.
Let's walk through a simple example of customizing the UserDetailsService
to retrieve user details from a database using JPA (Java Persistence API).
First, create a class that implements the UserDetailsService
interface and overrides the loadUserByUsername
method:
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserRepository userRepository;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userRepository.findByUsername(username)
.orElseThrow(() -> new UsernameNotFoundException("User not found with username: " + username));
return org.springframework.security.core.userdetails.User.builder()
.username(user.getUsername())
.password(user.getPassword())
.authorities(user.getRoles())
.build();
}
}
In this example, we use the UserRepository
to retrieve the user details based on the provided username. If the user is not found, we throw a UsernameNotFoundException
. We then construct a UserDetails
object using the retrieved user information, including the username, password, and authorities.
Next, you need to configure Spring Security to use your custom UserDetailsService
. This can be achieved by extending the WebSecurityConfigurerAdapter
class and overriding the configure
method:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomUserDetailsService customUserDetailsService;
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(customUserDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
In this configuration, we inject our custom UserDetailsService
implementation and specify the password encoder to be used for authentication. The BCryptPasswordEncoder
is a widely used password hashing algorithm that provides strong security for storing user passwords.
By customizing the UserDetailsService
and configuring it in Spring Security, you have full control over how user information is retrieved and authenticated in your application.
Closing the Chapter
In conclusion, customizing the UserDetailsService
in Spring Security allows you to integrate with custom user data sources and tailor the authentication process to the specific requirements of your application. By creating your own implementation of UserDetailsService
and configuring it with Spring Security, you can ensure secure and efficient user authentication within your Java web application.
Spring Security offers extensive customization capabilities beyond what was covered in this article. To delve deeper into the topic, consider exploring advanced features such as authentication providers, authentication managers, and user details services to gain a comprehensive understanding of implementing secure authentication in Spring applications.
For more in-depth information on Spring Security, consider referring to the official Spring Security documentation.
Customizing UserDetailsService
is just one aspect of the broader security landscape in Java applications, and understanding its role and implementation nuances is fundamental for building robust and secure systems.