Optimizing Performance with Parallel and Asynchronous Programming
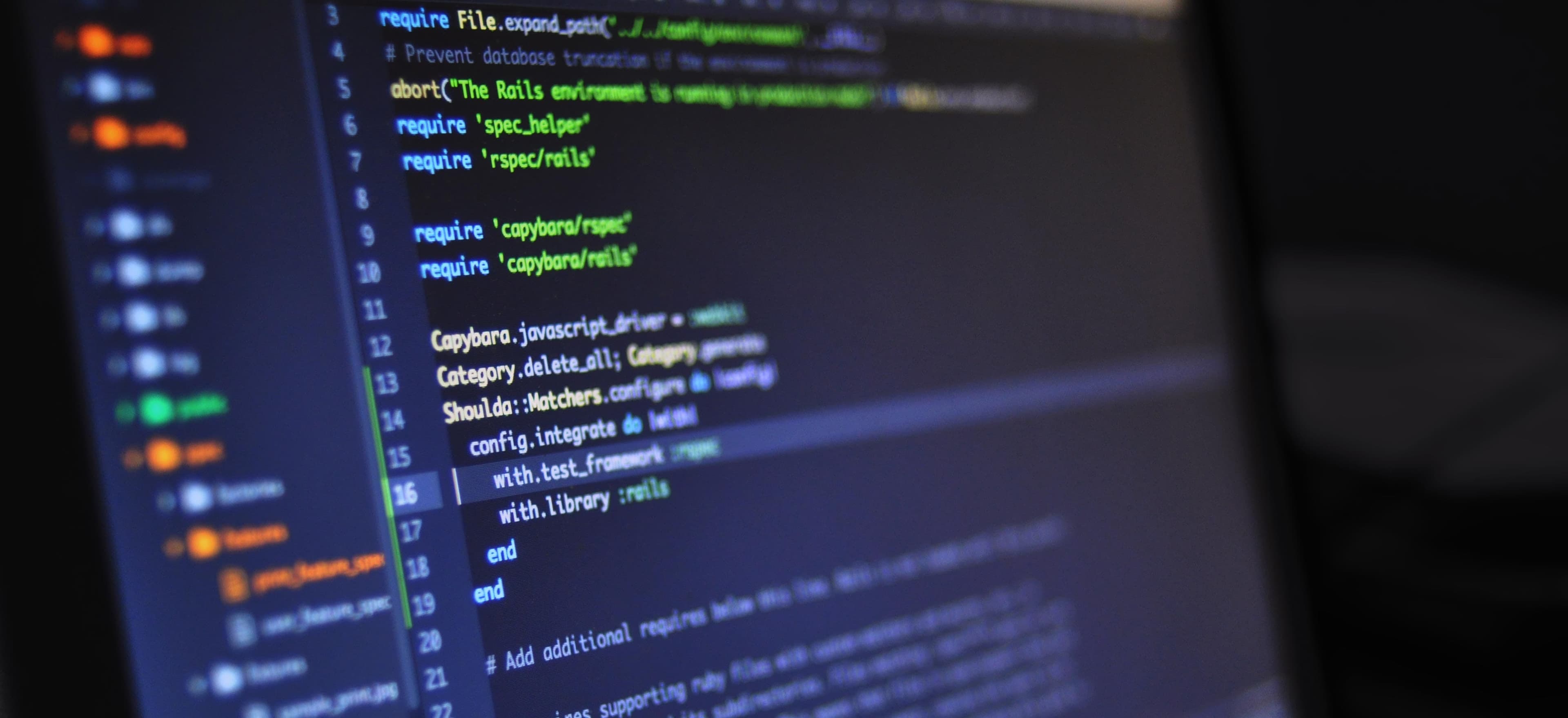
- Published on
Optimizing Performance with Parallel and Asynchronous Programming in Java
In the world of Java programming, optimizing performance is a critical aspect of developing high-quality applications. With the ever-increasing demand for faster and more responsive software, developers must explore advanced techniques to make their applications more efficient. In this blog post, we will delve into the concepts of parallel and asynchronous programming in Java and how these techniques can be leveraged to optimize performance.
Understanding Parallel and Asynchronous Programming
Parallel Programming
Parallel programming involves breaking down a task into subtasks that can be executed simultaneously, making use of multiple CPU cores to speed up the overall computation. In Java, parallel programming is typically achieved using the java.util.concurrent
package, which provides a rich set of classes and interfaces for writing concurrent code.
Asynchronous Programming
Asynchronous programming, on the other hand, focuses on handling I/O-bound operations and long-running tasks without blocking the main execution thread. This is especially beneficial in scenarios where waiting for I/O operations to complete can result in a significant waste of resources.
Leveraging Parallel Streams in Java
Java 8 introduced the concept of parallel streams, which allows for seamless parallelization of operations on collections. By simply invoking the parallelStream()
method on a collection, Java can leverage multiple threads to process the elements in parallel, provided that the operations are associative and stateless.
Let's consider an example where we have a list of numbers and we want to compute the sum of their squares. We can achieve this using parallel streams:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sumOfSquares = numbers.parallelStream()
.mapToInt(x -> x * x)
.sum();
In this example, the parallelStream()
method allows Java to perform the mapping and summation operations in parallel, resulting in improved performance for large collections.
It's important to note that not all operations are suitable for parallelization. Operations that involve blocking I/O, access shared mutable state, or have dependencies between elements may not yield the desired performance improvements and could even introduce concurrency issues.
Harnessing CompletableFuture for Asynchronous Operations
Java 8 also introduced the CompletableFuture
class, which provides a powerful way to work with asynchronous computations. It allows developers to express and compose asynchronous operations in a more declarative and composable manner.
Consider a scenario where we need to perform two asynchronous tasks and combine their results. We can use CompletableFuture
to achieve this concisely:
CompletableFuture<String> task1 = CompletableFuture.supplyAsync(() -> "Hello");
CompletableFuture<String> task2 = CompletableFuture.supplyAsync(() -> "World");
CompletableFuture<String> combinedResult = task1.thenCombine(task2, (result1, result2) -> result1 + " " + result2);
String finalResult = combinedResult.join();
In this example, we use supplyAsync()
to execute the tasks asynchronously. The thenCombine()
method then allows us to specify how the results of the two tasks should be combined when both are complete.
By employing CompletableFuture
, developers can effectively manage asynchronous operations, avoid callback hell, and streamline the handling of asynchronous tasks.
Pitfalls and Best Practices
While parallel and asynchronous programming can greatly enhance performance, it is crucial to approach these techniques with care to avoid potential pitfalls.
-
Thread Safety: When writing parallel code, special attention must be given to ensuring thread safety, especially when dealing with shared mutable state. The use of thread-safe data structures and synchronization mechanisms is essential to prevent data corruption and race conditions.
-
Monitoring and Tuning: Profiling and monitoring the application's performance is crucial when leveraging parallel and asynchronous programming. Identifying bottlenecks and optimizing resource utilization can significantly impact the overall efficiency of the application.
-
Graceful Degradation: It's important to design applications with graceful degradation in mind. While parallel and asynchronous programming can boost performance on multi-core systems, the application should still function efficiently on single-core or lower-powered devices.
By adhering to best practices and maintaining a thorough understanding of the underlying concepts, developers can harness the full potential of parallel and asynchronous programming while mitigating potential risks.
In Conclusion, Here is What Matters
In conclusion, parallel and asynchronous programming offer powerful tools for optimizing performance in Java applications. By leveraging parallel streams for parallelization of operations and CompletableFuture
for asynchronous task management, developers can create more efficient and responsive software.
However, it is imperative to approach these techniques with caution, considering thread safety, monitoring performance, and designing for graceful degradation. With the right approach and a solid understanding of parallel and asynchronous programming, developers can unlock the potential for significant performance gains in their Java applications.
In an ever-evolving technological landscape, staying abreast of advanced programming techniques is essential to delivering high-performing and scalable solutions.
Incorporating these concepts and best practices into your Java programming arsenal can elevate the quality and responsiveness of your applications, boosting user satisfaction and maintaining a competitive edge in the market.
Explore more about Java parallel streams in the official documentation.
For a deeper understanding of CompletableFuture in Java, refer to the insightful tutorial by Baeldung on Understanding CompletableFuture in Java.