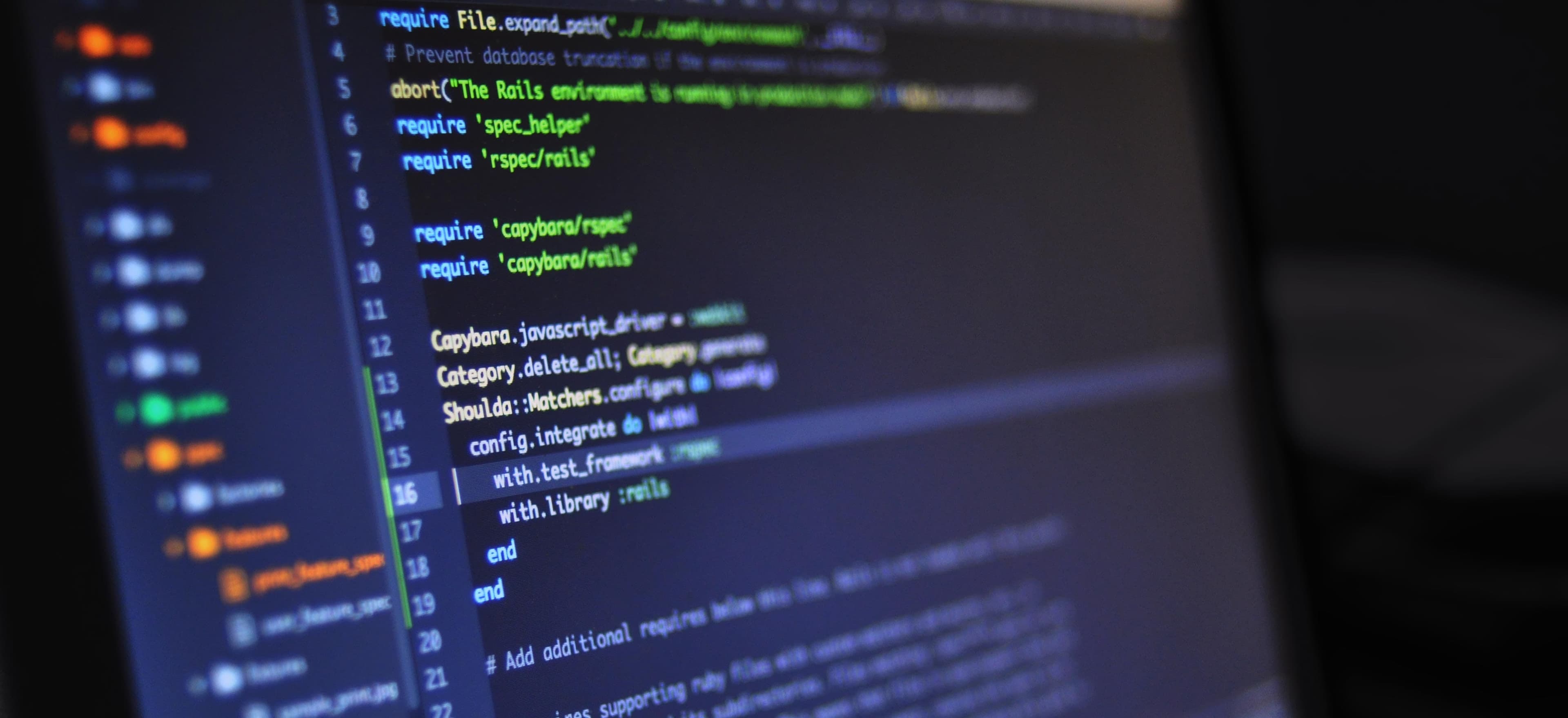
- Published on
Managing Flash Attributes in Spring MVC
In Spring MVC, flash attributes can be a useful tool for passing data from one request to another. They are typically used to show a message or provide feedback to the user after a redirect. In this article, we'll explore how to effectively manage flash attributes in a Spring MVC application.
Understanding Flash Attributes
Flash attributes are used to store temporary data that needs to survive a redirect. When a redirect is issued in a Spring MVC application, any flash attributes set in the first request are automatically made available in the subsequent request. After the redirect, the flash attributes are automatically removed, ensuring that they are only available for a single request.
Using Flash Attributes
To use flash attributes in a Spring MVC application, you can make use of the RedirectAttributes
interface. This interface provides methods for adding flash attributes that will be available in the redirected request. Let's take a look at an example of how to use flash attributes to display a success message after a form submission.
@Controller
public class UserController {
@Autowired
private UserService userService;
@PostMapping("/user/save")
public String saveUser(User user, RedirectAttributes redirectAttributes) {
userService.save(user);
redirectAttributes.addFlashAttribute("successMessage", "User saved successfully");
return "redirect:/user/list";
}
@GetMapping("/user/list")
public String listUsers() {
return "user-list";
}
}
In this example, when the saveUser
method is called, a success message is added as a flash attribute using the addFlashAttribute
method. After the user is saved, the method returns a redirect to the user list page. The success message will be available in the redirected request and can be displayed to the user.
Accessing Flash Attributes
In the redirected request, you can access the flash attributes using the RedirectAttributes
parameter in the handler method. You can then add the flash attributes to the model and display them in the view. Here's an example of how to do this:
@Controller
public class UserController {
@GetMapping("/user/list")
public String listUsers(RedirectAttributes redirectAttributes, Model model) {
// Add flash attributes to the model
model.addAllAttributes(redirectAttributes.getFlashAttributes());
return "user-list";
}
}
In this example, the listUsers
method adds all the flash attributes to the model using the addAllAttributes
method. These flash attributes can then be accessed in the user-list
view and displayed to the user.
Managing Flash Attributes with Redirect Options
When using flash attributes, you can also make use of additional redirection options provided by the RedirectAttributes
interface. For example, you can specify a custom flash attribute name or add a flash attribute conditionally based on certain criteria. Let's see how this can be done:
@Controller
public class UserController {
@PostMapping("/user/delete/{id}")
public String deleteUser(@PathVariable Long id, RedirectAttributes redirectAttributes) {
if (userService.delete(id)) {
redirectAttributes.addFlashAttribute("successMessage", "User deleted successfully");
} else {
redirectAttributes.addFlashAttribute("errorMessage", "Failed to delete user");
}
return "redirect:/user/list";
}
}
In this example, when a user is deleted, a success message or an error message is added as a flash attribute depending on the result of the deletion operation. This allows you to provide feedback to the user based on the outcome of the operation.
To Wrap Things Up
Flash attributes in Spring MVC provide a convenient way to pass temporary data between requests, especially in scenarios such as form submissions or user actions that result in a redirect. By effectively managing flash attributes, you can provide feedback to the user and enhance the user experience in your application.
In this article, we've covered how to use and manage flash attributes in a Spring MVC application, including adding flash attributes, accessing them in the redirected request, and using redirection options. Incorporating flash attributes into your Spring MVC application can improve the overall user experience and make your application more interactive and responsive.
To learn more about Spring MVC and flash attributes, you can refer to the official documentation and explore further examples and use cases. Happy coding!