Building Spring Boot Applications on WildFly: Solving Class Loading Issues
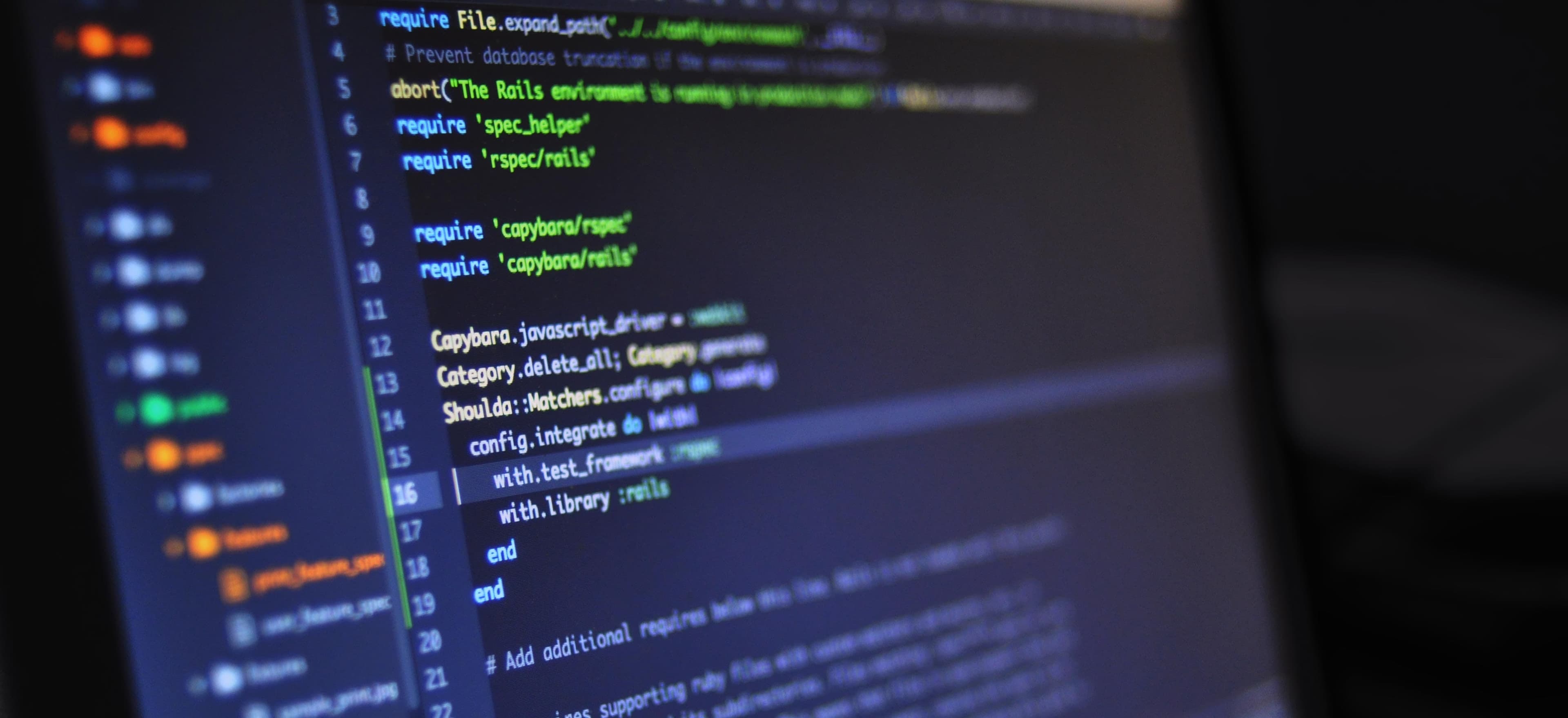
- Published on
Building Spring Boot Applications on WildFly: Solving Class Loading Issues
When it comes to building and deploying Java applications, Spring Boot has emerged as a popular framework due to its convenience and productivity features. However, integrating Spring Boot applications with WildFly, an application server that supports Java EE, can introduce challenges, particularly with class loading. In this post, we will explore the issues of class loading and provide practical solutions for successfully deploying Spring Boot applications on WildFly.
Understanding the Class Loading Issue
WildFly uses a modular class loading architecture that differs from the traditional flat classpath used by Spring Boot. This variance can lead to class loading conflicts, where classes required by the Spring Boot application are not accessible to the WildFly class loader, or vice versa.
The Problem
When deploying a Spring Boot application on WildFly, the class loading strategy employed by Spring Boot may clash with the WildFly class loading mechanism. This clash can result in class not found exceptions, link errors, or other runtime issues.
The Solution
To resolve class loading conflicts when using Spring Boot with WildFly, we can leverage a few strategies such as packaging the application as an executable JAR, using WildFly modules, and configuring the class loading behavior.
Packaging Spring Boot Application as an Executable JAR
Spring Boot provides a convenient way to package applications as executable JAR files, containing all the necessary dependencies. This approach ensures that the class loading behavior is controlled within the application itself, reducing the reliance on the class loading mechanism of the application server.
By using the spring-boot-maven-plugin
, the application can be packaged as an executable JAR using the following configuration in the pom.xml
file:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>${spring-boot.version}</version>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Why It Works
Packaging the Spring Boot application as an executable JAR encapsulates all the dependencies within the JAR file, ensuring that the application uses its own class loading mechanism rather than relying on the class loader provided by WildFly.
Using WildFly Modules
WildFly modules offer a way to organize and manage dependencies for applications deployed on the server. By creating a custom module for the Spring Boot dependencies, we can ensure that the required classes are accessible to the application without conflicting with the classloading strategy of WildFly.
To create a WildFly module for Spring Boot dependencies, we can follow these steps:
- Define module structure: Create a module directory within the WildFly installation and define the module XML file specifying the dependencies.
- Copy dependencies: Copy the required JAR files of the Spring Boot dependencies to the module directory.
- Reference module: Reference the custom module in the deployment descriptor of the application to ensure the classes are loaded from the module.
Why It Works
By creating a custom module for Spring Boot dependencies, we can isolate the class loading of these dependencies, preventing conflicts with the native WildFly class loader.
Configuring Class Loading Behavior
WildFly provides configuration options to customize class loading behavior for applications. By defining module dependencies and class loading paths in the deployment descriptor, we can ensure that the required classes are resolved correctly without conflicting with the class loading strategy of WildFly.
<jboss-deployment-structure>
<deployment>
<dependencies>
<module name="com.example.springboot" />
</dependencies>
</deployment>
</jboss-deployment-structure>
Why It Works
By explicitly defining module dependencies and class loading paths, we can control how classes are loaded, preventing conflicts with the default class loading mechanism of WildFly.
Closing the Chapter
Deploying Spring Boot applications on WildFly requires careful handling of class loading to avoid conflicts and runtime issues. By packaging the application as an executable JAR, using WildFly modules, and configuring the class loading behavior, we can ensure a smooth integration of Spring Boot with WildFly.
In conclusion, understanding the class loading issues and employing the appropriate strategies is essential for seamless deployment and execution of Spring Boot applications on WildFly. With the right approach, developers can harness the power of both Spring Boot and WildFly to build robust and scalable Java applications.
For further understanding, refer to the official documentation and WildFly developer guide for advanced class loading techniques and best practices.