Optimizing Message Properties for Efficient Code Retrieval
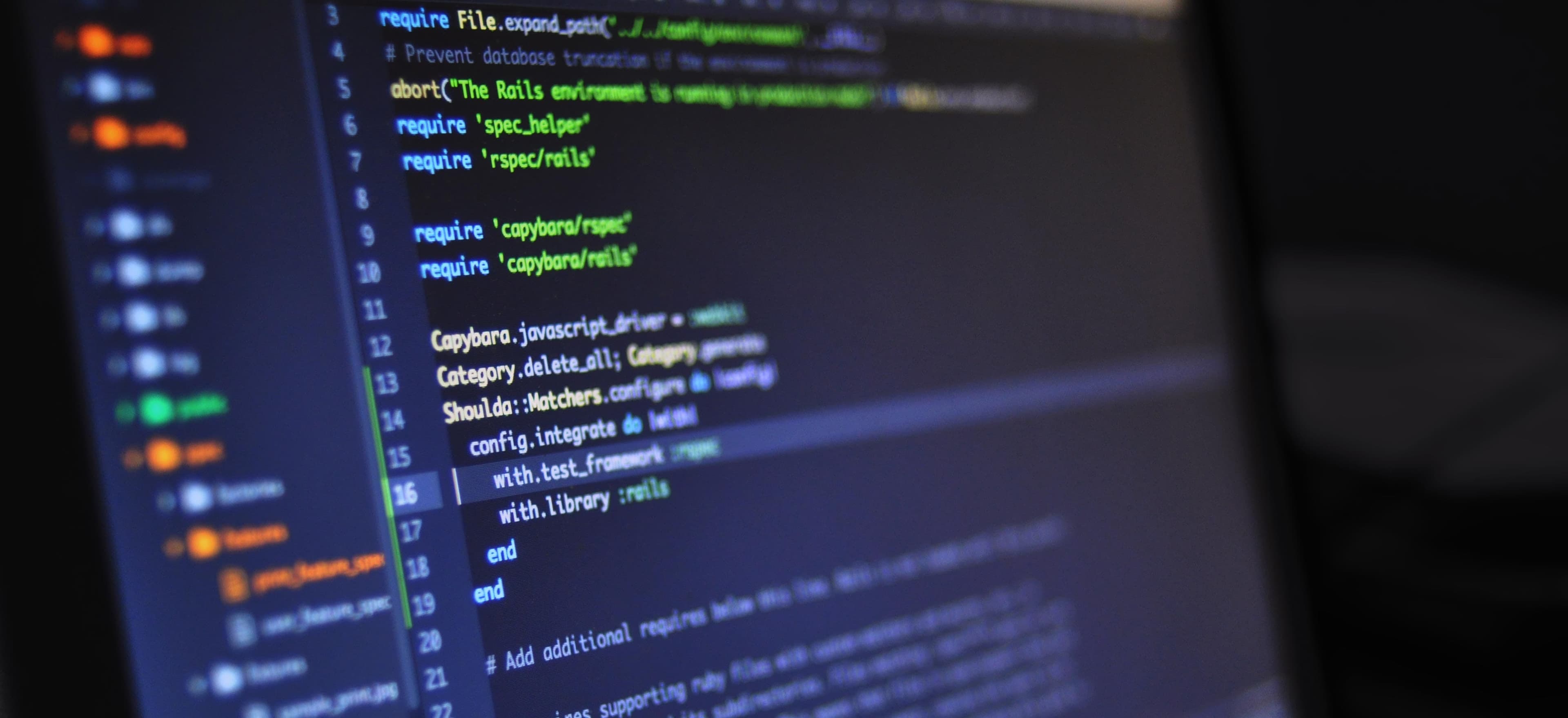
- Published on
Optimizing Message Properties for Efficient Java Code Retrieval
When working with Java applications, it's crucial to ensure that the retrieval of message properties is as efficient as possible. Message properties are often utilized for internationalization and localization, enabling applications to support multiple languages and regional differences. In this article, we will explore techniques for optimizing the retrieval of message properties in Java, which can lead to improved performance and maintainability.
Understanding Message Properties
In Java, message properties are commonly stored in .properties
files. These files contain key-value pairs, where the keys are used to look up the corresponding messages in the desired language. When an application needs to display a message, it accesses the appropriate .properties
file for the current locale and retrieves the message using its associated key.
Let's consider an example where we have a messages.properties
file containing the following entries:
greeting_en = Hello!
greeting_fr = Bonjour!
In this scenario, greeting_en
and greeting_fr
are the keys, each associated with a message in English and French, respectively.
Traditional Approach: Utilizing ResourceBundle
In Java, the standard way to retrieve message properties from .properties
files is by using the ResourceBundle
class. The following code demonstrates the typical approach for message retrieval:
import java.util.ResourceBundle;
import java.util.Locale;
public class MessageRetrievalExample {
public static void main(String[] args) {
ResourceBundle messages = ResourceBundle.getBundle("messages", Locale.FRANCE);
String greeting = messages.getString("greeting_fr");
System.out.println(greeting);
}
}
While using ResourceBundle
is straightforward and serves the purpose, it may not always offer the best performance, especially in scenarios where message retrieval is frequent or where a large number of message properties are involved.
Optimization Technique 1: Utilizing Properties Files Directly
In cases where performance is critical, bypassing the ResourceBundle
abstraction and working directly with properties files can lead to improved efficiency. By reading and caching properties files manually, we can reduce the overhead associated with ResourceBundle
lookups.
The following example demonstrates how to directly load and retrieve properties from a .properties
file:
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import java.util.Locale;
public class DirectPropertiesAccessExample {
public static void main(String[] args) {
Properties properties = new Properties();
try (InputStream input = DirectPropertiesAccessExample.class.getClassLoader().getResourceAsStream("messages_fr.properties")) {
properties.load(input);
} catch (IOException e) {
e.printStackTrace();
}
String greeting = properties.getProperty("greeting_fr");
System.out.println(greeting);
}
}
Why Direct Access May Provide Optimization
Directly accessing and caching properties files can offer performance benefits due to the avoidance of the resource bundle lookup process. In situations where message retrieval occurs frequently, the overhead of resource bundle instantiation and access can be mitigated.
By manually managing the properties file loading and caching, we exercise granular control over the retrieval process, potentially resulting in faster access times and decreased resource consumption.
Optimization Technique 2: Utilizing Caching Mechanisms
Another effective optimization strategy involves implementing a caching mechanism for message properties. By caching frequently accessed properties, we can minimize file I/O operations and enhance overall retrieval efficiency.
One popular library for implementing caching in Java applications is Guava, which provides a robust and easy-to-use caching framework.
Let's utilize Guava's LoadingCache
to implement a simple caching mechanism for message properties:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.ExecutionException;
public class MessagePropertyCachingExample {
private static LoadingCache<String, String> propertiesCache = CacheBuilder.newBuilder()
.build(new CacheLoader<String, String>() {
@Override
public String load(String key) {
// Logic to load properties from files goes here
return loadPropertyFromFile(key);
}
});
public static void main(String[] args) {
try {
String greeting = propertiesCache.get("greeting_fr");
System.out.println(greeting);
} catch (ExecutionException e) {
e.printStackTrace();
}
}
// Simulated method to load properties from file
private static String loadPropertyFromFile(String key) {
// Logic to read and return property value
return "Bonjour!";
}
}
In the above example, we create a LoadingCache
instance that automatically loads and caches properties based on specified keys. The CacheLoader
implementation defines the logic for loading properties from files when they aren't yet cached.
Benefits of Caching
Introducing a caching mechanism as demonstrated with Guava's LoadingCache
can significantly improve message property retrieval performance. By caching frequently accessed properties, we reduce the need for repetitive file I/O operations, leading to faster access times and reduced system overhead.
The Bottom Line
Efficient retrieval of message properties is crucial for the performance and usability of Java applications, particularly those supporting internationalization and localization. By understanding the traditional ResourceBundle
approach and exploring optimization techniques such as direct properties file access and caching mechanisms, developers can enhance the efficiency of message property retrieval.
Optimizing message property retrieval not only improves performance but also contributes to the overall user experience of the application, ensuring that messages are delivered swiftly and accurately in the desired language.
In conclusion, while the traditional ResourceBundle
approach suffices for many scenarios, considering the optimization techniques discussed in this article can be valuable for applications with high message retrieval demands, ultimately leading to responsive and resource-efficient software.
By implementing these optimization techniques, Java developers can elevate the performance and efficiency of their applications when dealing with message properties.
Checkout our other articles