Handling Form Validation in Java EE MVC
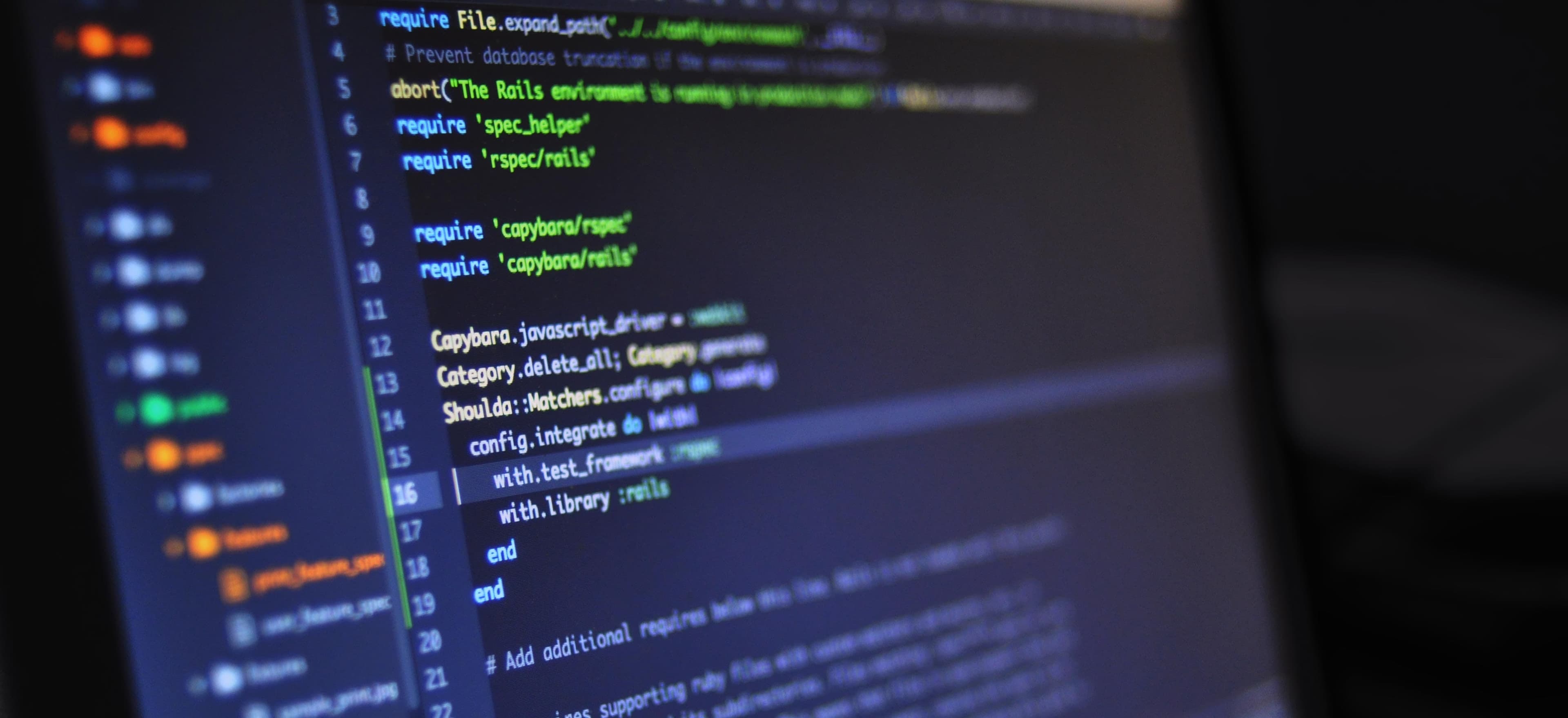
- Published on
Handling Form Validation in Java EE MVC
In developing web applications, form validation plays a crucial role in ensuring data accuracy and consistency. Java EE (Enterprise Edition) MVC (Model-View-Controller) provides a robust framework for building web applications, and it offers built-in features for handling form validation. In this article, we will explore how to effectively handle form validation in Java EE MVC.
Overview of Form Validation in Java EE MVC
Form validation in Java EE MVC is typically performed using validation annotations that are part of the Bean Validation API. This API allows developers to express constraints on object models via annotations. When a form is submitted, the data is automatically validated based on these constraints.
Setting Up the Project
Before diving into form validation, let's set up a basic Java EE MVC project. If you don't have a project set up, you can refer to the official documentation on Creating a new Java EE MVC application.
Assuming you have a basic Java EE MVC project set up, we can now proceed to implement form validation.
Implementing Form Validation
To demonstrate form validation, let's consider a simple registration form for a web application. The form includes fields for the user's name, email, and password. We want to ensure that the submitted data meets certain criteria, such as being non-null and meeting specific length requirements.
Creating the Model
First, let's create a model class that represents the data submitted through the registration form. We'll use validation annotations provided by the Bean Validation API to enforce constraints on the model attributes.
import javax.validation.constraints.*;
public class User {
@NotNull
@Size(min = 2, max = 30)
private String name;
@NotNull
@Email
private String email;
@NotNull
@Size(min = 8, max = 20)
private String password;
// Getters and setters
}
In this example, we've used @NotNull
to ensure that the fields are not empty, @Size
to define the length constraints, and @Email
to validate the email format.
Handling Form Submission in the Controller
In the controller class responsible for processing the registration form, we can leverage the form validation capabilities provided by Java EE MVC. By injecting the model with the @Valid
annotation, we can trigger the validation process.
import javax.mvc.Controller;
import javax.mvc.Models;
import javax.mvc.View;
import javax.validation.Valid;
import javax.ws.rs.*;
import javax.inject.Inject;
@Controller
@Path("registration")
@View("registrationForm.jsp")
public class RegistrationController {
@Inject
private Models models;
@POST
@Path("submit")
public String submitForm(@Valid @BeanParam User user) {
// Process the validated user data
return "confirmation.jsp";
}
}
By annotating the User
parameter with @Valid
, the Bean Validation API will automatically validate the submitted form data based on the constraints defined in the User
model class.
Displaying Validation Errors in the View
To provide feedback to the user in case of validation errors, we need to handle and display the error messages in the view. We can achieve this by leveraging the automatic population of the BindingResult
in the request attributes.
<form action="submit" method="post">
<input type="text" name="name" value="${user.name}">
<span class="error">${bindingResult.user.name}</span>
<input type="text" name="email" value="${user.email}">
<span class="error">${bindingResult.user.email}</span>
<input type="password" name="password">
<span class="error">${bindingResult.user.password}</span>
<input type="submit" value="Submit">
</form>
In the view, we can access the validation error messages through the bindingResult
object, which is automatically populated with the error messages for each field based on the defined constraints.
A Final Look
In this article, we've explored how to handle form validation in Java EE MVC using the Bean Validation API. By leveraging validation annotations, we can ensure data integrity and provide meaningful feedback to users when validation errors occur.
Form validation is an essential aspect of web application development, and Java EE MVC simplifies the process by integrating with the Bean Validation API, allowing for declarative validation constraints.
By following the principles outlined in this article, you can create robust and reliable form validation logic in your Java EE MVC applications, ensuring a smooth user experience and data consistency.
Checkout our other articles