Optimizing Data Loading with Spring Batch
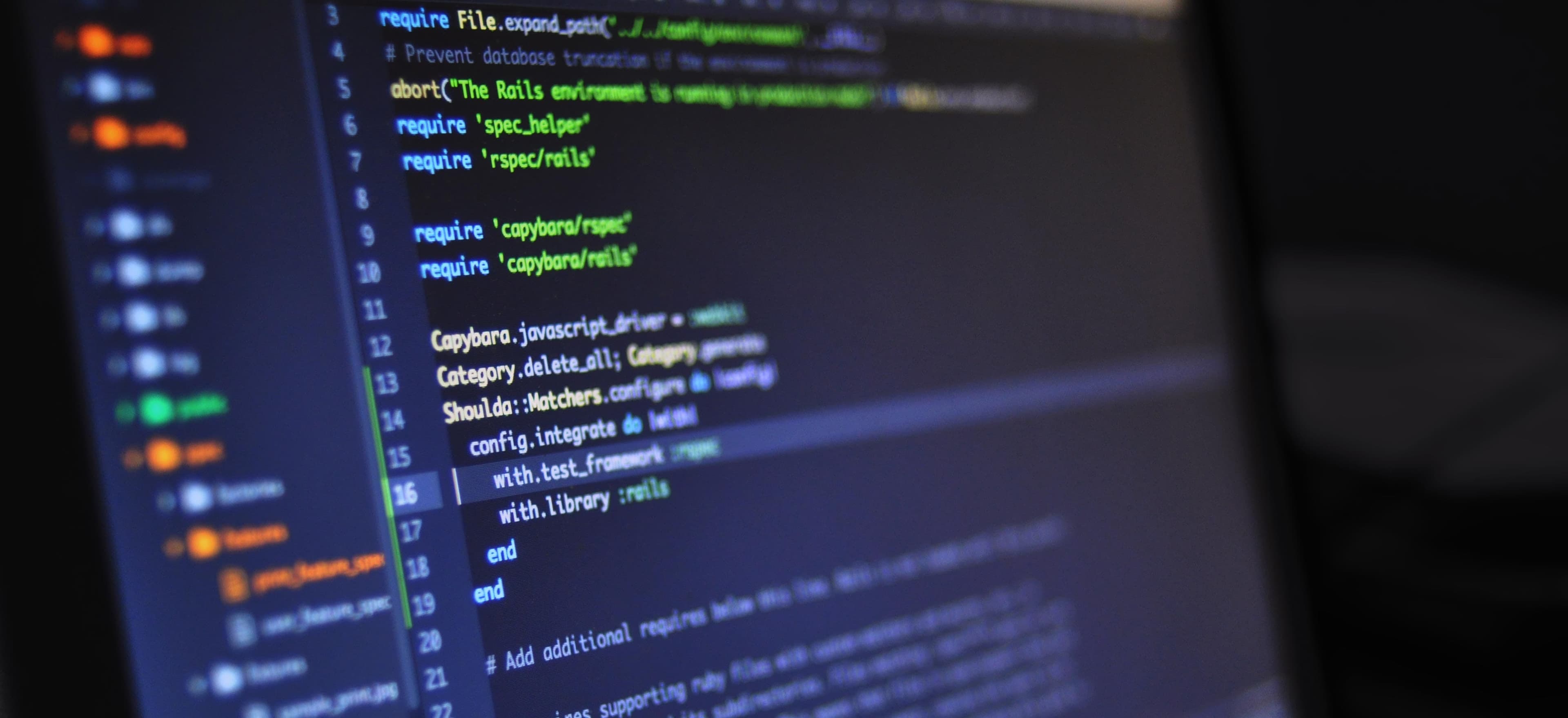
- Published on
Optimizing Data Loading with Spring Batch
Loading and processing large volumes of data is a common task in enterprise applications. It's crucial to handle this process efficiently to ensure optimal performance. Spring Batch, a lightweight, comprehensive batch framework, provides reusable functions to process vast amounts of records, including logging/tracing, transaction management, job processing statistics, job restart, skip, and resource management. In this blog post, we'll explore strategies and best practices for optimizing data loading using Spring Batch.
Understanding Spring Batch
Spring Batch provides a set of APIs and components to process and analyze large volumes of data. It is built on the principles of batch processing and addresses common batch processing concerns such as reading large datasets, processing data efficiently, and writing the processed data.
Key Components of Spring Batch
Spring Batch includes the following key components:
-
Job: A job encapsulates a batch process. It consists of one or more steps, each of which can involve the reading, processing, and writing of data.
-
Step: A step is an independent and sequential phase of a job. It consists of an item reader, processor, and writer, allowing the sequential processing of data.
-
ItemReader: An ItemReader reads data from a data source. Spring Batch provides various ItemReader implementations to read data from diverse sources such as files, databases, and web services.
-
ItemProcessor: An ItemProcessor processes input data and returns the processed data. It can be used for data transformation, validation, or filtering.
-
ItemWriter: An ItemWriter writes the processed data to a destination. Spring Batch includes various ItemWriter implementations for writing data to different destinations such as databases, files, and APIs.
Strategies for Optimizing Data Loading
Chunk Processing
One of the key optimizations in Spring Batch is chunk processing. In chunk processing, instead of processing one item at a time, the framework processes data in chunks, significantly reducing the overhead of committing data and communication with the data source.
By default, Spring Batch uses a chunk-oriented processing model. We can configure the chunk size to define the number of items to be read, processed, and written in a single transaction. This allows us to fine-tune the processing throughput based on the characteristics of the data source and the processing logic.
Let's take a look at an example configuration for chunk processing in Spring Batch:
@Bean
public Step dataLoadingStep(ItemReader<Data> itemReader,
ItemProcessor<Data, ProcessedData> itemProcessor,
ItemWriter<ProcessedData> itemWriter) {
return stepBuilderFactory.get("dataLoadingStep")
.<Data, ProcessedData>chunk(1000)
.reader(itemReader)
.processor(itemProcessor)
.writer(itemWriter)
.build();
}
In the above code snippet, we've defined a step for data loading with a chunk size of 1000, indicating that 1000 items will be read, processed, and written within a single transaction.
Parallel Processing
When dealing with large datasets, parallel processing can significantly improve data loading performance. Spring Batch allows us to parallelize step execution by partitioning the data and processing each partition in parallel.
By leveraging Spring Batch's partitioning features, we can divide the data into multiple partitions, where each partition is processed independently. This is particularly beneficial when processing data from diverse sources or when applying complex processing logic.
Let's consider an example of partitioning in Spring Batch:
@Bean
public Step partitionedStep(ItemReader<Data> itemReader,
ItemProcessor<Data, ProcessedData> itemProcessor,
ItemWriter<ProcessedData> itemWriter) {
return stepBuilderFactory.get("partitionedStep")
.partitioner(slaveStep().getName(), partitioner)
.step(slaveStep())
.gridSize(4) // Number of partitions
.taskExecutor(taskExecutor)
.build();
}
@Bean
public Step slaveStep() {
return stepBuilderFactory.get("slaveStep")
.<Data, ProcessedData>chunk(1000)
.reader(itemReader)
.processor(itemProcessor)
.writer(itemWriter)
.build();
}
In this example, we've defined a partitioned step that uses a partitioner to divide the data into multiple partitions. The slave step is then executed for each partition in parallel, with the specified grid size and task executor.
Paging and Batching
When interacting with external data sources, such as databases or remote APIs, paging and batching can improve the efficiency of data retrieval and processing. Spring Batch provides built-in support for paging and batching, allowing us to fetch and process data in manageable chunks.
By using paging and batching, we can avoid loading the entire dataset into memory at once, thus reducing memory consumption and improving overall performance. This becomes crucial when working with large datasets that cannot be accommodated in memory.
Let's see an example of using paging and batching in Spring Batch:
@Bean
public JdbcPagingItemReader<Data> pagingItemReader(DataSource dataSource) {
return new JdbcPagingItemReaderBuilder<Data>()
.dataSource(dataSource)
.selectClause("SELECT *")
.fromClause("FROM data_table")
.fetchSize(1000)
.rowMapper(new BeanPropertyRowMapper<>(Data.class))
.build();
}
In the above code snippet, we've created a JdbcPagingItemReader
to read data from a database table in a paged manner, fetching 1000 records at a time. This allows for efficient retrieval and processing of large datasets without overwhelming the memory.
Error Handling and Retry
Error handling and retry mechanisms are essential for ensuring the robustness of data loading operations. Spring Batch provides built-in support for handling errors and implementing retry strategies, allowing us to gracefully manage exceptional scenarios during data processing.
By configuring error handling and retry policies, we can specify how the framework should handle errors such as data validation failures, network timeouts, or transient database issues. This ensures that data loading processes can recover from failures and continue processing without manual intervention.
Let's consider an example of configuring error handling and retry in Spring Batch:
@Bean
public Step dataLoadingStep(ItemReader<Data> itemReader,
ItemProcessor<Data, ProcessedData> itemProcessor,
ItemWriter<ProcessedData> itemWriter) {
return stepBuilderFactory.get("dataLoadingStep")
.<Data, ProcessedData>chunk(1000)
.reader(itemReader)
.processor(itemProcessor)
.writer(itemWriter)
.faultTolerant()
.skipLimit(100)
.skip(DataIntegrityViolationException.class)
.retryLimit(3)
.retry(ConnectTimeoutException.class)
.build();
}
In this example, we've configured the data loading step with fault-tolerant behavior, specifying a skip limit and defining the exceptions to be skipped. We've also set a retry limit and specified the exceptions for which retries should be attempted. This ensures that the data loading process can handle errors gracefully and recover from transient issues.
Final Considerations
Efficient data loading is crucial for the performance and reliability of batch processing applications. Spring Batch provides powerful features and optimizations to handle large datasets effectively. By leveraging strategies such as chunk processing, parallel processing, paging and batching, and error handling/retry, we can optimize data loading operations and ensure the scalability and robustness of batch processing jobs.
In conclusion, understanding and implementing these strategies in Spring Batch can greatly enhance the efficiency and reliability of data loading processes in enterprise applications.
To dive deeper into Spring Batch optimization techniques, you can explore the official Spring Batch documentation and examine real-world examples and best practices.
Optimizing data loading with Spring Batch is not only about improving performance but also about building resilient and scalable batch processing solutions.
Remember, efficient data loading is the backbone of high-performance batch processing applications!
Checkout our other articles