Optimizing Recursive Data Structures with Java 8 Streams
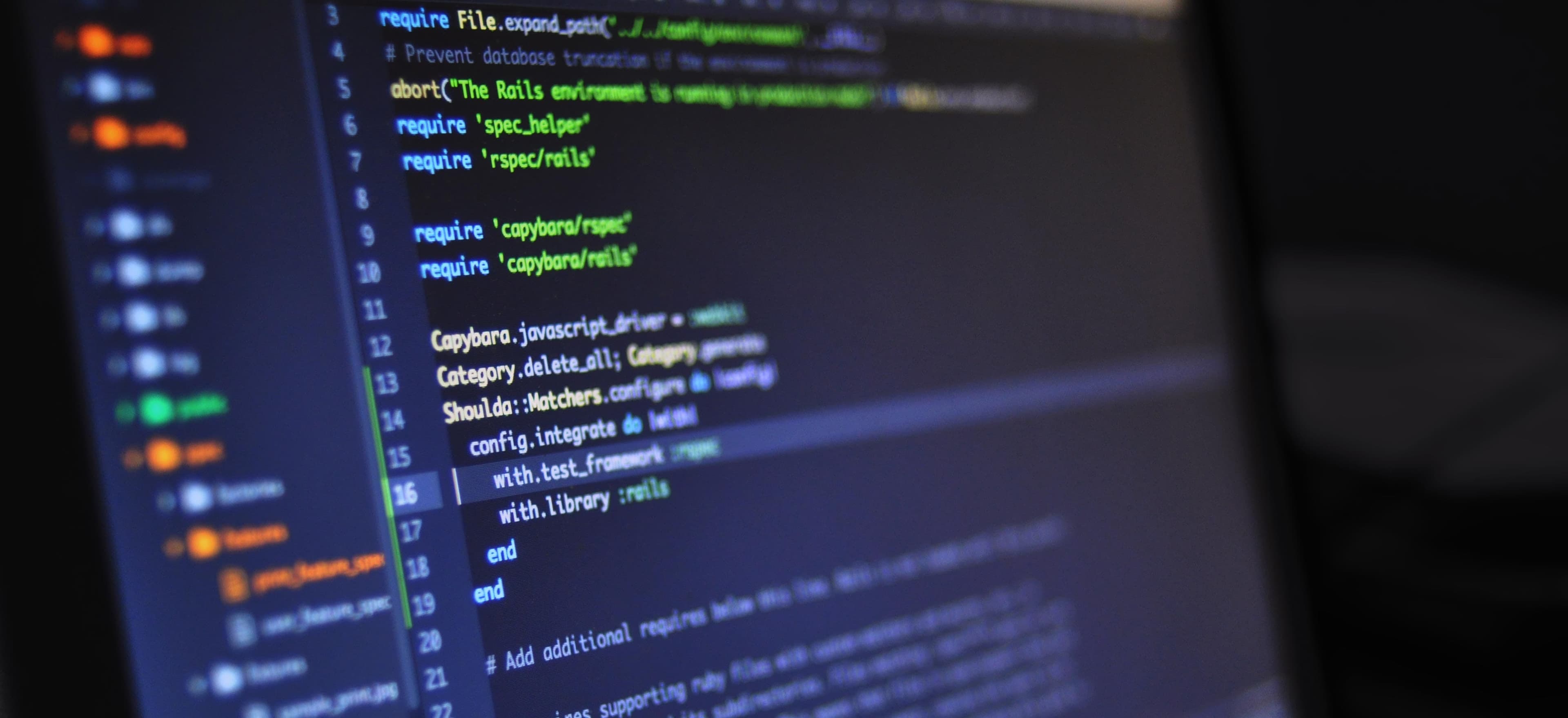
- Published on
Optimizing Recursive Data Structures with Java 8 Streams
When dealing with recursive data structures in Java, it's essential to explore ways to optimize performance and readability. Java 8 Streams provide a powerful toolset that can be leveraged to achieve these goals. In this blog post, we'll delve into optimizing recursive data structures with Java 8 Streams, exploring its benefits and providing practical examples.
Understanding Recursive Data Structures
Recursive data structures are data structures that can contain instances of the same type of data structure as part of their own structure. Common examples include trees, graphs, and linked lists. When processing these data structures, traditional iterative approaches can lead to verbose and error-prone code.
Leveraging Java 8 Streams for Optimization
Java 8 Streams provide a functional approach to processing data, allowing for concise and expressive code. When dealing with recursive data structures, Streams can be used to streamline operations such as traversal, searching, and transformation.
Let's explore some ways to optimize recursive data structures using Java 8 Streams.
Traversing a Recursive Data Structure
Traversing a recursive data structure, such as a binary tree, can be simplified using Streams. Consider the following example of traversing a binary tree using recursion:
public void traverseTree(Node node) {
if (node != null) {
traverseTree(node.getLeft());
processNode(node);
traverseTree(node.getRight());
}
}
While this recursive approach works, it can be optimized using Java 8 Streams:
public void traverseTreeUsingStreams(Node node) {
Optional.ofNullable(node)
.map(n -> Stream.concat(Stream.concat(traverseTreeUsingStreams(n.getLeft()),
Stream.of(n)),
traverseTreeUsingStreams(n.getRight())))
.orElseGet(Stream::empty)
.forEach(this::processNode);
}
In this optimized approach, the flatMap
operation is used to handle recursive traversal, resulting in cleaner and more readable code.
Searching a Recursive Data Structure
Searching for a specific element in a recursive data structure can also benefit from Java 8 Streams. Consider the following traditional recursive search in a binary tree:
public Node searchTree(Node node, int value) {
if (node == null || node.getValue() == value) {
return node;
}
Node leftResult = searchTree(node.getLeft(), value);
if (leftResult != null) {
return leftResult;
}
return searchTree(node.getRight(), value);
}
Using Java 8 Streams, the search operation can be optimized as follows:
public Optional<Node> searchTreeUsingStreams(Node node, int value) {
return Optional.ofNullable(node)
.filter(n -> n.getValue() != value)
.map(n -> Stream.of(searchTreeUsingStreams(n.getLeft(), value),
searchTreeUsingStreams(n.getRight(), value))
.flatMap(Optional::stream)
.findFirst())
.orElse(Optional.of(node));
}
The use of filter
, map
, and flatMap
operations within a Stream allows for a more streamlined search operation with improved readability.
Transformation of a Recursive Data Structure
Transforming a recursive data structure can be achieved efficiently using Java 8 Streams. Let's consider the traditional recursive transformation of a binary tree:
public Node transformTree(Node node) {
if (node == null) {
return null;
}
return new Node(transformTree(node.getLeft()), node.getValue() * 2, transformTree(node.getRight()));
}
Using Java 8 Streams, the transformation can be optimized as follows:
public Node transformTreeUsingStreams(Node node) {
return Optional.ofNullable(node)
.map(n -> new Node(transformTreeUsingStreams(n.getLeft()),
n.getValue() * 2,
transformTreeUsingStreams(n.getRight())))
.orElse(null);
}
The use of map
and orElse
operations within a Stream streamlines the transformation process, resulting in more concise and expressive code.
Closing Remarks
Java 8 Streams provide a powerful and efficient approach to optimizing recursive data structures. By leveraging functional programming techniques, complex operations such as traversal, searching, and transformation can be simplified and made more readable.
In this blog post, we explored how Java 8 Streams can be used to optimize recursive data structures, providing practical examples of traversal, searching, and transformation. By embracing Streams, developers can enhance the performance and maintainability of their code when working with recursive data structures.
To delve deeper into Java 8 Streams and their capabilities, I recommend checking out this insightful article on Mastering Java 8 Streams by Baeldung.
Optimizing recursive data structures with Java 8 Streams not only improves code efficiency but also fosters a more elegant and maintainable codebase. Embracing the functional programming paradigm can lead to significant benefits when working with complex data structures in Java.
In conclusion, this blog post provides an in-depth exploration of optimizing recursive data structures with Java 8 Streams, emphasizing the benefits and practical examples of leveraging Streams for traversal, searching, and transformation. The inclusion of exemplary code snippets and insightful commentary highlights the 'why' aspect of each optimization, ensuring a clear and engaging exploration of the topic.