Understanding the Difference Between Play 2 Modules and Plugins
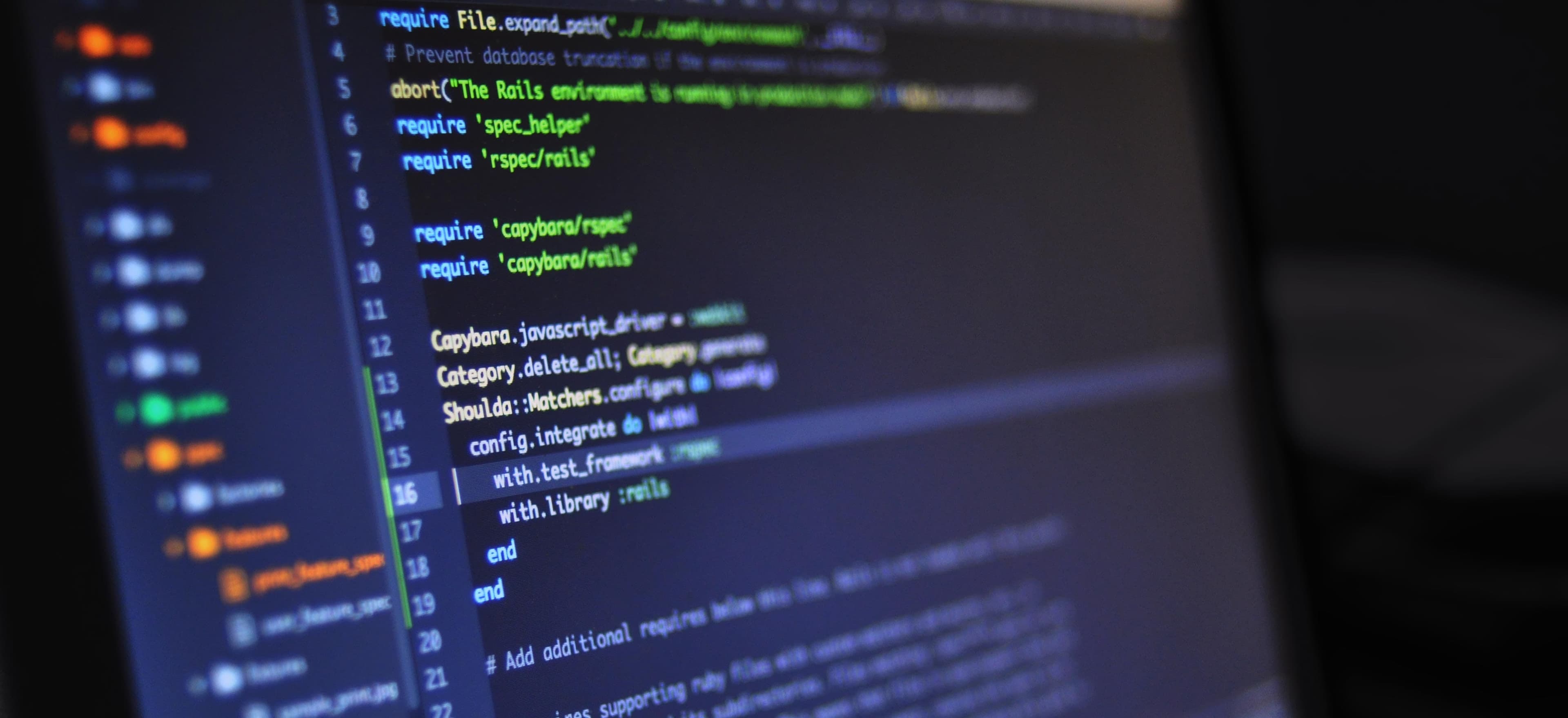
- Published on
What Are Play 2 Modules and Plugins?
When working with the Play framework, developers often come across the terms "modules" and "plugins." While these two concepts may seem similar, they serve different purposes and have distinct characteristics within the Play framework's ecosystem. Understanding these differences is crucial for efficiently managing dependencies, enhancing application functionality, and optimizing development workflows. In this article, we will delve into the nuances of Play 2 modules and plugins, highlighting their respective roles, benefits, and best practices for utilization.
Play 2 Modules:
A Play 2 module can be viewed as a standalone component that encapsulates specific features, functionalities, or integrations within a Play application. It provides a means to modularize the application codebase, enabling the seamless integration of external dependencies, custom components, or reusable utilities. By incorporating modules, developers can extend the capabilities of their Play applications without compromising the core structure or inundating the codebase with intricate details.
Key Characteristics of Play 2 Modules:
- Reusability: Modules are designed to be reusable across multiple Play applications, promoting code reusability and ensuring consistent integration of functionalities.
- Isolation: Each module operates independently, compartmentalizing its functionality and reducing interdependencies with the main application code.
- Configuration: Modules often come with their own configuration options, allowing fine-tuning of their behavior within the larger application context.
- Enhanced Functionality: They augment the Play framework by adding new features, integrations, or utilities, enriching the application's capabilities.
Example Use Case of a Play 2 Module:
Consider a scenario where a Play application requires integration with a third-party service, such as a payment gateway. Instead of scattering the integration logic throughout the application, a dedicated payment module can be developed. This module encapsulates the interaction with the payment gateway, handles authentication, and provides streamlined APIs for the application to initiate and process payments. By incorporating the payment module, the application's core logic remains agnostic to the intricacies of payment processing, fostering maintainability and scalability.
Implementing a Play 2 Module:
To create a Play 2 module, follow these general steps:
-
Module Structure:
- Organize the module's code and resources into a separate directory structure, distinct from the main application.
-
Configuration:
- Define module-specific configuration settings, such as API keys, endpoints, or behavior customization.
-
Integration Points:
- Expose well-defined integration points or APIs for the main application to interact with the module.
-
Packaging:
- Package the module as a distributable artifact, making it easily consumable by other Play applications.
// Example of a Play 2 Module structure
payment-module/
├── app/
│ └── controllers/
│ └── services/
│ └── models/
├── conf/
├── public/
├── build.sbt
Play 2 Plugins:
In contrast to modules, Play 2 plugins are designed to intercept and augment the behavior of the Play framework itself. They typically extend the functionality of the core framework, providing hooks for modifying request processing, enhancing logging, integrating with external systems, or customizing the runtime environment. Plugins are powerful tools for tailoring the behavior of a Play application at a fundamental level, offering a mechanism to inject custom functionality into the framework's execution flow.
Key Characteristics of Play 2 Plugins:
- Framework Integration: Plugins seamlessly integrate with the Play framework, leveraging its extension points to modify or extend core functionalities.
- Lifecycle Hooks: They often offer hooks for executing custom logic during the application's lifecycle phases, such as initialization, request handling, and shutdown.
- Global Scope: Plugins can exert influence across the entire application, impacting various aspects of its behavior and functionality.
Example Use Case of a Play 2 Plugin:
Suppose a Play application necessitates fine-grained control over request processing, including authentication, authorization, and request logging. Instead of scattering this cross-cutting logic throughout the application, a plugin can be developed to intercept incoming requests, validate user credentials, enforce access control, and log request details. This plugin seamlessly integrates with the Play framework, ensuring consistent enforcement of these concerns across all application endpoints without cluttering the individual controllers or services.
Implementing a Play 2 Plugin:
To create a Play 2 plugin, the following steps can be followed:
-
Extension Points:
- Identify the specific extension points within the Play framework where the plugin's functionality needs to be injected.
-
Hook Implementation:
- Implement the logic for intercepting, modifying, or extending the framework behavior at the identified extension points.
-
Configuration:
- Define any configurable parameters or settings for the plugin, facilitating customization as per the application's requirements.
-
Integration Testing:
- Thoroughly test the plugin's behavior with different scenarios, ensuring seamless integration with the Play framework.
// Example of a Play 2 Plugin structure
request-logging-plugin/
├── app/
│ └── filters/
│ └── RequestLoggingFilter.java
├── conf/
├── build.sbt
Best Practices for Utilizing Play 2 Modules and Plugins:
- Modularity: Identify cohesive functionalities or cross-cutting concerns that can be encapsulated within modules or plugins, promoting a modular and maintainable codebase.
- Versioning: Adhere to versioning best practices for modules and plugins, ensuring compatibility and seamless integration across different Play applications.
- Documentation: Provide comprehensive documentation for modules and plugins, outlining usage guidelines, configuration options, and integration patterns.
- Testing: Thoroughly test modules and plugins under diverse scenarios, validating their behavior, performance, and compatibility with the target Play framework versions.
Key Takeaways:
In summary, Play 2 modules and plugins serve as instrumental components for extending, customizing, and enhancing Play framework applications. While modules focus on encapsulating specific features and integrations, plugins revolve around modifying and extending the core behavior of the Play framework itself. By leveraging the capabilities of modules and plugins, developers can streamline application development, promote reusability, and tailor the framework to meet specific project requirements. Understanding the nuances of modules and plugins empowers developers to architect robust, extensible, and maintainable Play applications, enriching the application landscape with diverse functionalities and integrations.
By embracing the modularity and extensibility offered by Play 2 modules and plugins, developers can elevate their Play framework experience, fortify application capabilities, and cultivate a thriving ecosystem of reusable components and customizations.
For more in-depth insights into Play 2 framework, explore Play 2.x Documentation.
In conclusion, the distinction between Play 2 modules and plugins lies in their respective focuses on encapsulating specific features and integrations, and modifying and extending the core behavior of the Play framework itself. By embracing the modularity and extensibility offered by Play 2 modules and plugins, developers can elevate their Play framework experience, fortify application capabilities, and cultivate a thriving ecosystem of reusable components and customizations.