Converting JSON to XML using XStream
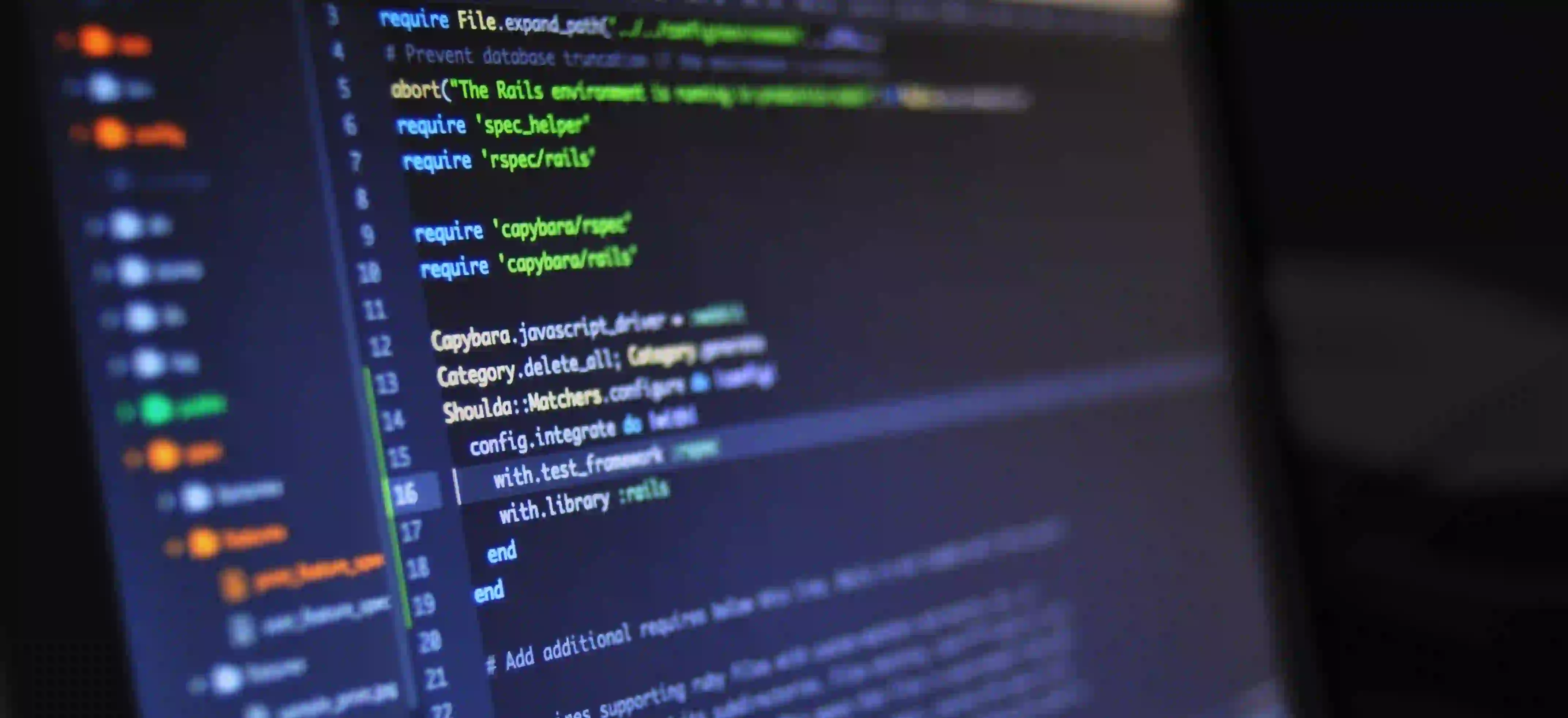
Converting JSON to XML using XStream in Java
In this post, we will explore how to convert JSON data to XML format using XStream, a powerful and easy-to-use Java library for serializing objects to and from XML and JSON. XStream provides a simple API for converting objects to XML and JSON and vice versa, making it an excellent choice for handling data interchange formats in Java applications.
Dependencies
First, let's include the XStream library in our Maven project. Add the following dependency to your pom.xml
file:
<dependency>
<groupId>com.thoughtworks.xstream</groupId>
<artifactId>xstream</artifactId>
<version>1.4.11.1</version>
</dependency>
Converting JSON to XML
Suppose we have the following JSON data that we want to convert to XML:
{
"person": {
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Main St",
"city": "Anytown"
}
}
}
We can use XStream to convert this JSON data to XML. First, let's create a Java class that represents the structure of the JSON data:
public class Person {
private String name;
private int age;
private Address address;
// Getters and setters
}
public class Address {
private String street;
private String city;
// Getters and setters
}
Using XStream to Convert JSON to XML
Now, let's use XStream to perform the conversion. We will create an instance of XStream and use its toXML
method to serialize the Person
object to XML:
import com.thoughtworks.xstream.XStream;
public class JsonToXmlConverter {
public static void main(String[] args) {
XStream xstream = new XStream();
xstream.alias("person", Person.class); // Set alias for root element
String json = "{\"person\":{\"name\":\"John Doe\",\"age\":30,\"address\":{\"street\":\"123 Main St\",\"city\":\"Anytown\"}}}";
Person person = (Person) xstream.fromXML(json);
String xml = xstream.toXML(person);
System.out.println(xml);
}
}
In this example, we first create an instance of XStream
. We then set an alias for the root element to match the JSON structure. Next, we create a Person
object from the JSON data using the fromXML
method. Finally, we use the toXML
method to serialize the Person
object to XML.
A Final Look
In this post, we have seen how to use XStream to convert JSON data to XML in a Java application. XStream provides a convenient and straightforward way to handle data interchange between JSON and XML formats. By following the examples and code snippets provided in this post, you can easily integrate JSON to XML conversion functionality into your Java projects.
For more information on XStream, you can visit the official XStream website.
Happy coding!