Optimizing Memory Usage for Efficient Performance
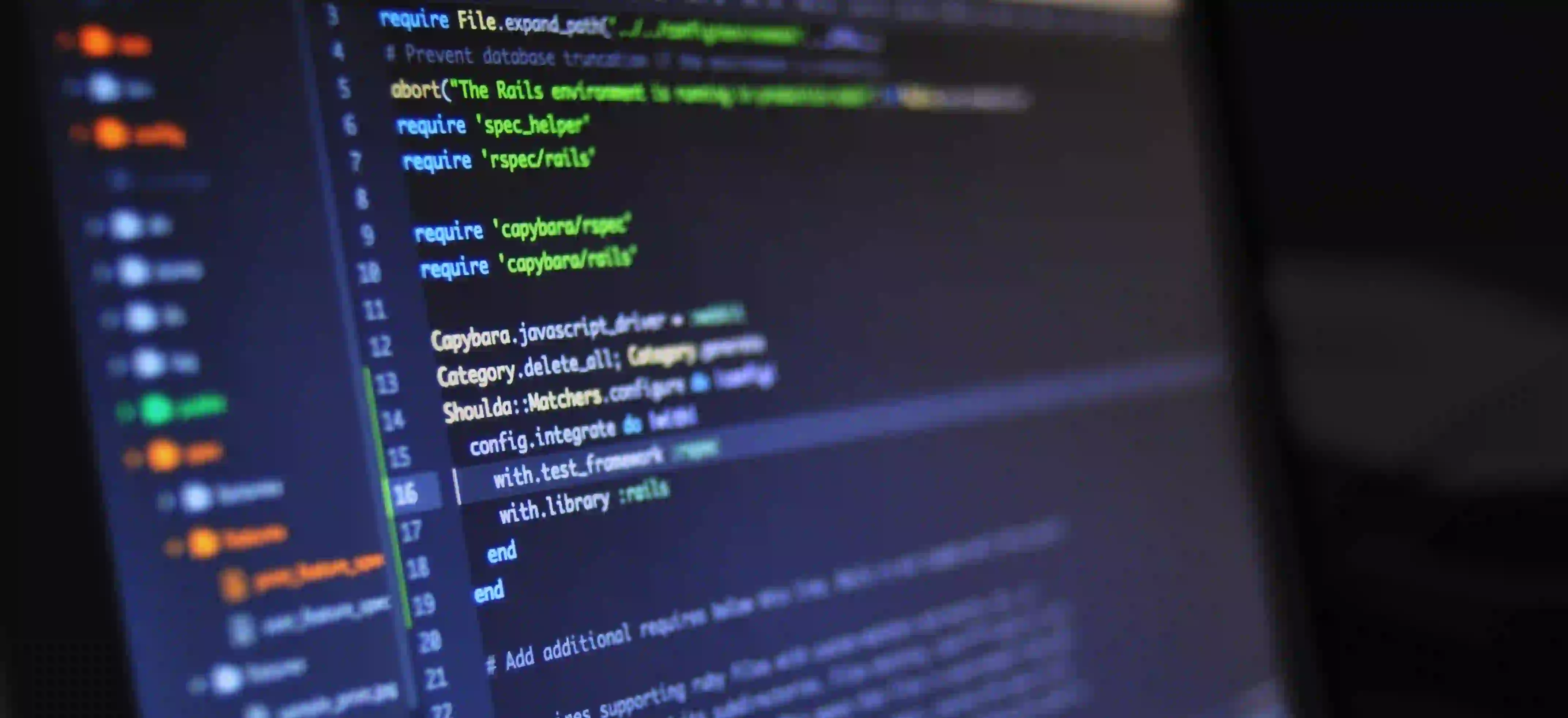
Optimizing Memory Usage for Efficient Performance
In Java programming, optimizing memory usage is crucial for ensuring efficient performance in applications. By managing memory allocation and usage effectively, developers can reduce the risk of memory leaks, improve garbage collection, and enhance overall application performance. In this article, we will explore various techniques and best practices for optimizing memory usage in Java applications.
1. Use Primitive Types Instead of Wrapper Classes
When working with numerical values in Java, it is often more memory-efficient to use primitive types such as int
, double
, float
, etc., instead of their corresponding wrapper classes (Integer
, Double
, Float
, etc.). Primitive types consume less memory as they directly store the value, while wrapper classes carry additional overhead due to the encapsulation of the primitive value within an object.
Example:
// Less memory-efficient
Integer wrapperValue = 10;
// More memory-efficient
int primitiveValue = 10;
2. Minimize Object Creation
Excessive object creation can lead to increased memory usage and impact application performance. To minimize object instantiation, consider reusing objects or utilizing object pools when dealing with frequently used objects.
Example:
// Inefficient object creation within a loop
List<String> stringList = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
String newString = new String("Value" + i);
stringList.add(newString);
}
// Optimized reuse of StringBuilder
StringBuilder reusableBuilder = new StringBuilder();
for (int i = 0; i < 1000; i++) {
reusableBuilder.setLength(0); // Clear the existing content
reusableBuilder.append("Value").append(i);
String result = reusableBuilder.toString();
stringList.add(result);
}
In the optimized example, the StringBuilder
is reused for constructing strings, reducing the number of object instantiations.
3. Use Immutable Objects
Immutable objects not only provide thread safety but also offer memory efficiency. Since immutable objects cannot be modified once created, they eliminate the need for additional memory allocations caused by mutations.
4. Optimize Collections Usage
When working with collections, choose the most suitable implementation based on the requirements to minimize memory overhead. For instance, using ArrayList
may be memory-efficient for larger lists due to lower per-element overhead compared to LinkedList
.
5. Effective Garbage Collection Management
Understanding and fine-tuning the garbage collection process is essential for memory optimization. Balancing the heap size, choosing appropriate garbage collector algorithms, and minimizing unnecessary object retention can significantly impact memory utilization.
6. Utilize Caching Mechanisms
Caching frequently accessed or computed data can reduce the need for frequent recomputation or retrieval, thereby conserving memory and improving application performance. Java provides various caching libraries such as Ehcache and Guava that can be integrated into applications.
7. Monitor and Analyze Memory Usage
Utilize tools like VisualVM, JConsole, or Java Mission Control to monitor and analyze memory usage in real-time. Identifying memory hotspots and analyzing memory consumption patterns can help in pinpointing areas for optimization.
Lessons Learned
Optimizing memory usage in Java applications is a critical aspect of ensuring efficient performance and resource utilization. By adhering to best practices such as using primitive types, minimizing object creation, leveraging immutable objects, optimizing collections usage, managing garbage collection effectively, utilizing caching mechanisms, and monitoring memory usage, developers can enhance the scalability and reliability of their applications while conserving system resources.
By actively considering memory optimization techniques throughout the development lifecycle, Java applications can achieve improved responsiveness, reduced latency, and overall better performance.
Remember, optimizing memory usage is not just about efficient resource utilization but also about creating a more robust and scalable application.
References:
- Primitive Data Types in Java
- Understanding Garbage Collection in Java
- Ehcache
- Guava Caching
- VisualVM
- JConsole
- Java Mission Control