Common Performance Issues in JVM Applications
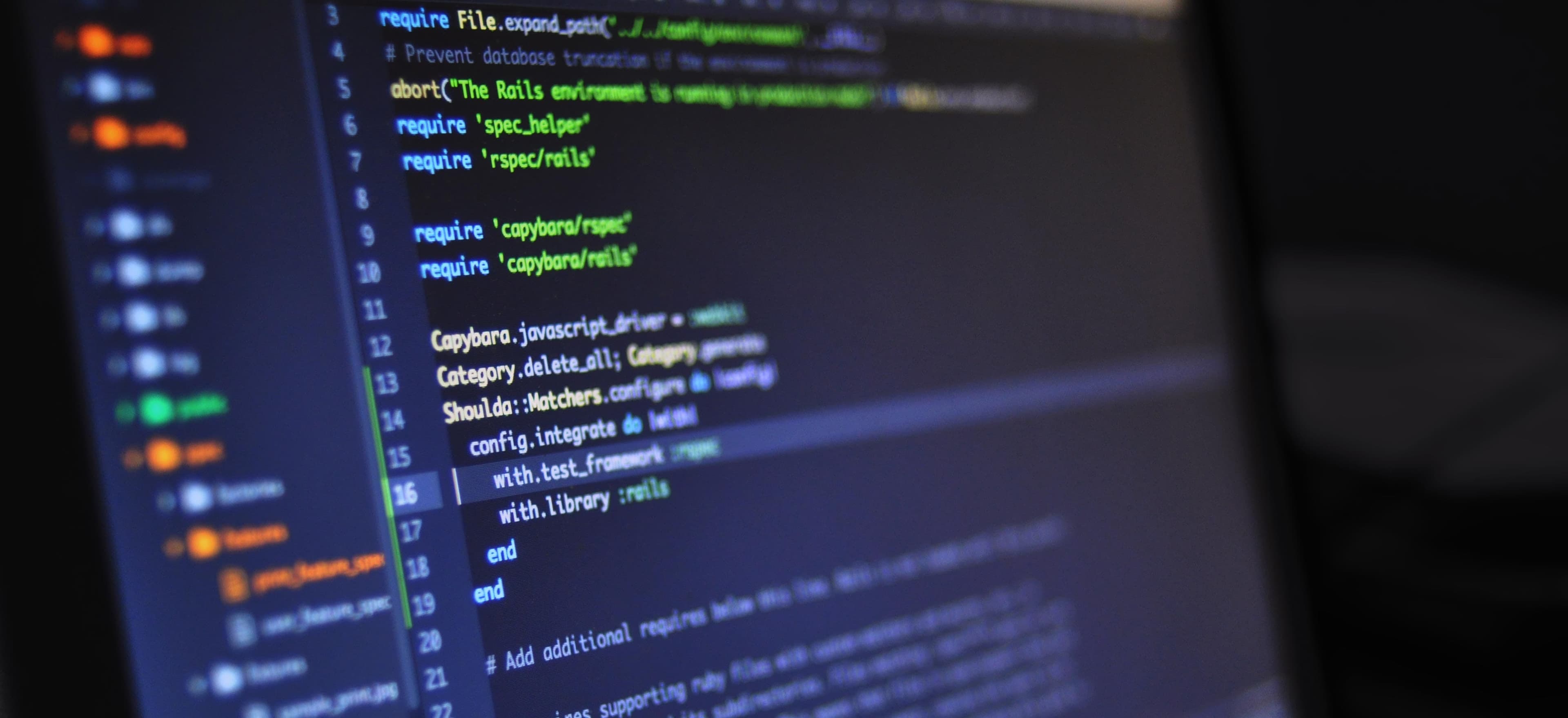
- Published on
Common Performance Issues in JVM Applications
When it comes to Java applications, performance is a critical aspect that can make or break the user experience. Despite the advantages of the Java Virtual Machine (JVM), performance issues can still arise, impacting the efficiency and responsiveness of applications. In this article, we'll explore some of the common performance issues in JVM applications and discuss potential solutions to address them.
1. Memory Leaks
Memory leaks are a prevalent issue in Java applications, where objects are no longer needed but are still held in memory. This can lead to increased garbage collection overhead and eventually result in OutOfMemoryError.
Solution:
Using a memory profiler, such as VisualVM or YourKit, can help identify memory leaks by analyzing heap dumps and object retention. Additionally, employing efficient coding practices like using try-with-resources for resources that need to be explicitly closed can prevent memory leaks.
try (BufferedReader br = new BufferedReader(new FileReader(file))) {
// Read file contents
} catch (IOException e) {
// Handle exception
}
2. Inefficient Garbage Collection
Inefficient garbage collection can cause significant performance degradation due to long pauses, especially in large-scale applications.
Solution:
Tuning garbage collection settings using flags like -XX:+UseConcMarkSweepGC
and -XX:NewRatio
can improve garbage collection performance. Utilizing a garbage collection log analysis tool, such as GCTimeLog, can provide insights into garbage collection patterns and help fine-tune the configuration.
3. Suboptimal Database Access
Inefficient database access, such as performing too many queries or fetching excessive data, can severely impact application performance.
Solution:
Utilizing techniques like batching SQL statements, caching frequently accessed data, and employing appropriate indexing can significantly enhance database access performance. Implementing an Object-Relational Mapping (ORM) framework like Hibernate can also optimize database interactions.
4. Excessive Synchronization
Excessive use of synchronization can lead to contention and thread contention, consequently hampering application performance.
Solution:
Using finer-grained locking or employing concurrent data structures from the java.util.concurrent
package can alleviate synchronization overhead and enhance application scalability. Additionally, leveraging lock-free algorithms and practices can further mitigate synchronization issues.
5. Inefficient Algorithm and Data Structures
Poorly designed algorithms and inefficient data structures can lead to suboptimal performance, especially when dealing with large datasets.
Solution:
Analyzing and optimizing algorithms and data structures to ensure they exhibit the desired time and space complexity is essential. Utilizing built-in data structures and algorithms from the Java Collections Framework, like HashMap
and Collections.sort()
, can contribute to improved performance.
// Example of using HashMap for efficient data retrieval
Map<String, Integer> wordFrequencyMap = new HashMap<>();
// Perform operations on the wordFrequencyMap
6. Substandard I/O Operations
Inefficient I/O operations, such as reading from or writing to files in a non-optimized manner, can significantly impact application performance.
Solution:
Leveraging NIO (New I/O) and asynchronous I/O can enhance the efficiency of I/O operations by utilizing non-blocking I/O channels and selectors. Employing buffered I/O streams for reading and writing data can also improve I/O performance.
// Example of using NIO for efficient I/O
Path filePath = Paths.get("file.txt");
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(filePath, StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
// Perform asynchronous I/O operations
Final Considerations
In conclusion, addressing performance issues in JVM applications requires a combination of proactive monitoring, efficient coding practices, and targeted optimization strategies. By identifying and mitigating common performance bottlenecks, Java applications can deliver enhanced responsiveness and scalability, ultimately improving the overall user experience.
For additional insights into JVM performance tuning, exploring tools like JVisualVM and Java Mission Control can provide valuable performance analysis and optimization capabilities.
Remember, pinpointing and rectifying performance issues is an iterative process, and continuous monitoring and fine-tuning are vital for maintaining optimal application performance.
Incorporating these best practices and solutions can pave the way for robust and high-performing JVM applications that meet the demands of modern software environments.
In this article, we covered the most common performance issues related to Java applications running on the JVM. By accurately identifying these issues, we provided comprehensive solutions aimed at optimizing the application’s performance. We hope these insights will equip you with the knowledge and strategies to enhance the performance of your Java applications effectively. Thank you for reading, and stay tuned for more insightful content!
Checkout our other articles