Optimizing Configuration in MicroProfile Projects
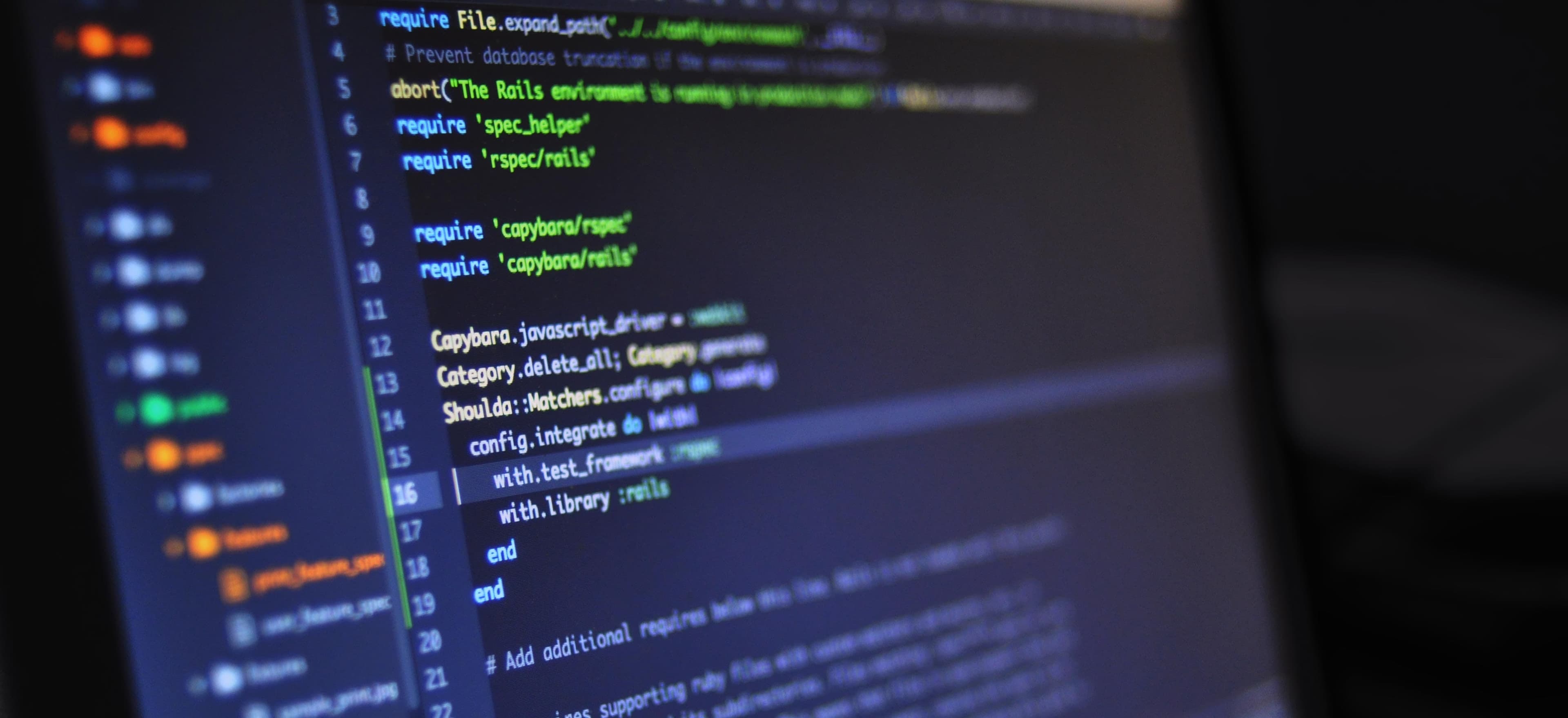
- Published on
Optimizing Configuration in MicroProfile Projects
MicroProfile is a popular programming model that's optimized for building microservices using Java. When working on MicroProfile projects, optimizing configuration is crucial for enhancing performance and ensuring seamless scalability. In this article, we'll explore some best practices for optimizing configuration in MicroProfile projects, along with relevant code examples and explanations.
Understanding MicroProfile Config
MicroProfile Config provides a standard way to externalize configuration from microservices. It allows developers to retrieve configuration properties from various sources such as environment variables, system properties, property files, and more. By utilizing MicroProfile Config, developers can easily access and manage configuration properties without hardcoding them into the application code.
Best Practices for Optimizing Configuration in MicroProfile Projects
1. Externalize Configuration Properties
Instead of hardcoding configuration properties within the codebase, externalize them using MicroProfile Config. This allows for greater flexibility, easier maintenance, and improved reusability of the configuration properties.
Here's an example of how to use MicroProfile Config to retrieve a configuration property:
@Inject
@ConfigProperty(name = "database.url")
private String databaseUrl;
In this example, the @ConfigProperty
annotation is used to inject the value of the database.url
configuration property into the databaseUrl
field.
2. Utilize Config Sources
MicroProfile Config supports multiple config sources, including system properties, environment variables, and property files. By leveraging these config sources, developers can dynamically manage configuration properties based on the environment in which the microservice is deployed.
<dependency>
<groupId>org.eclipse.microprofile.config</groupId>
<artifactId>microprofile-config-api</artifactId>
</dependency>
<dependency>
<groupId>org.eclipse.microprofile.config</groupId>
<artifactId>microprofile-config-implementation</artifactId>
</dependency>
In this code snippet, the microprofile-config-api
and microprofile-config-implementation
dependencies are added to the project's pom.xml
file to enable the usage of MicroProfile Config.
3. Refreshable Configurations
In a dynamic microservices environment, it's essential to support config property updates without restarting the microservice. MicroProfile Config provides a way to achieve this with refreshable configurations.
@ConfigProperty(name = "database.url")
private Instance<String> databaseUrl;
By using Instance
to inject a configuration property, the value can be refreshed at runtime without restarting the microservice.
4. Use Type-safe Configuration
Avoid using string literals to retrieve configuration properties. Instead, define a dedicated configuration interface with type-safe methods for accessing the properties.
public interface DatabaseConfig {
@ConfigProperty(name = "database.url")
String url();
@ConfigProperty(name = "database.username")
String username();
@ConfigProperty(name = "database.password")
String password();
}
By creating a type-safe configuration interface, developers can easily access and manage configuration properties with the benefits of compile-time safety and refactoring support.
5. Encryption and Decryption of Sensitive Data
When dealing with sensitive configuration properties such as passwords or API keys, it's crucial to encrypt and decrypt them to prevent exposure. MicroProfile Config allows for integrating custom encryption and decryption mechanisms for securing sensitive configuration data.
6. Implementing Custom Config Sources
In some scenarios, developers may need to retrieve configuration properties from custom sources such as a database or a remote configuration store. MicroProfile Config provides extensibility for implementing custom config sources to meet specific project requirements.
Final Thoughts
Optimizing configuration in MicroProfile projects is essential for maintaining flexibility, scalability, and security. By externalizing configuration properties, leveraging config sources, supporting refreshable configurations, using type-safe access, and implementing custom encryption, developers can ensure that their MicroProfile projects are well-equipped for dynamic and secure environments.
With MicroProfile Config, developers can efficiently manage configuration properties across microservices, making it an indispensable tool for building robust and scalable microservices in Java.
By following these best practices, developers can streamline the configuration management process and enhance the overall performance and maintainability of their MicroProfile projects.
For more in-depth information on MicroProfile Config, refer to the official MicroProfile Config documentation.
Start optimizing your MicroProfile projects with these best practices today!
Checkout our other articles