Java 15: Exploring its Performance Improvements
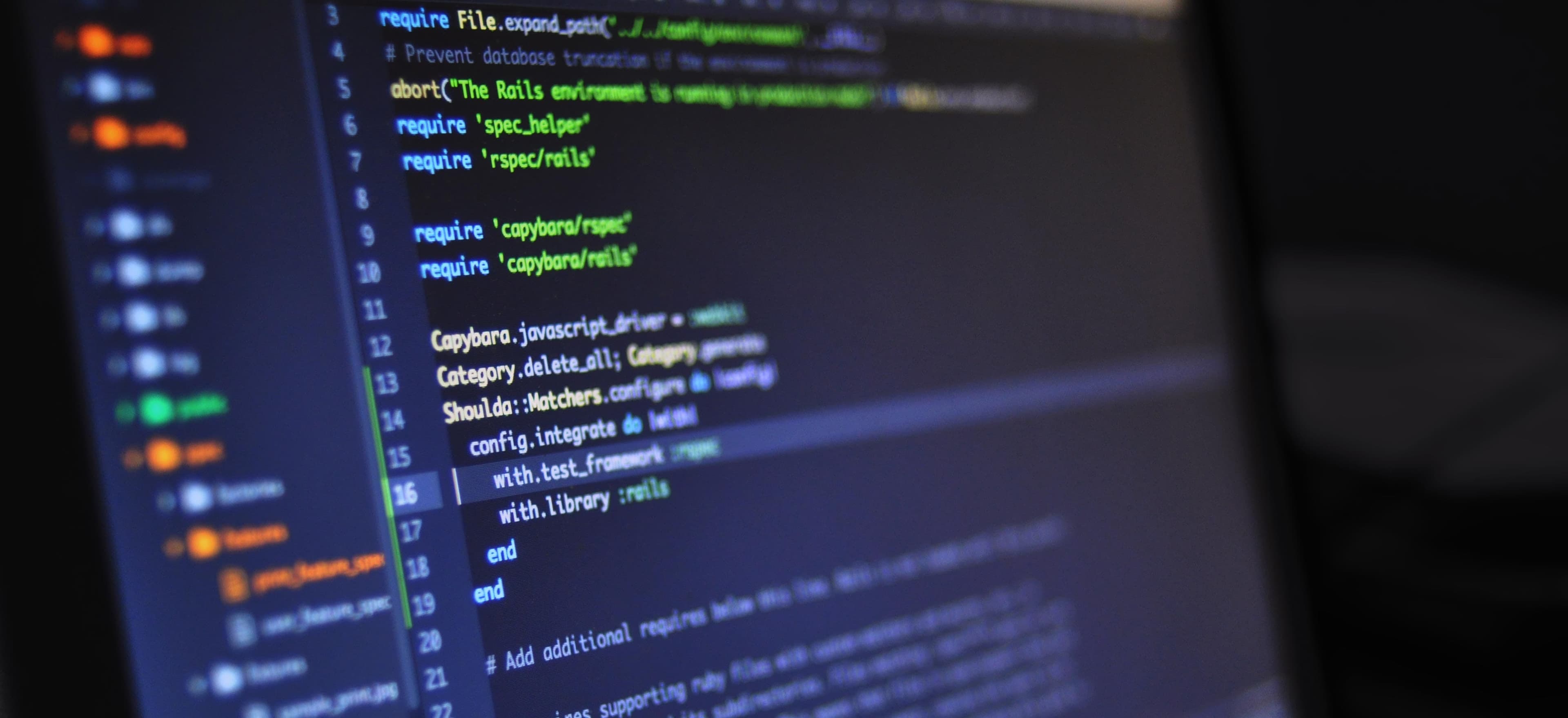
- Published on
Java 15: Exploring its Performance Improvements
Java, as a high-level programming language, has a broad range of applications, including desktop, web, and mobile app development. With each new release, Java continues to evolve, introducing new features and improvements. In Java 15, one of the key areas of focus is performance enhancements. This blog post will delve into the performance improvements introduced in Java 15 and how they can benefit developers.
ZGC: Concurrent Thread-Stack Processing
One of the notable performance enhancements in Java 15 is the introduction of Concurrent Thread-Stack Processing in Z Garbage Collector (ZGC). This feature aims to reduce the latency caused by stack scanning during the garbage collection process. The stack processing, which was previously performed along with the garbage collection pause, is now carried out concurrently. This results in shorter pause times, making ZGC even more suitable for low-latency applications.
// Concurrent Thread-Stack Processing Example in ZGC
-Xmx8G -Xms8G -XX:+UnlockExperimentalVMOptions -XX:+UseZGC
In the example above, the flags enable the ZGC with 8GB of initial and maximum heap size. The -XX:+UnlockExperimentalVMOptions
allows the use of experimental VM options, while -XX:+UseZGC
activates the ZGC. These options enable developers to leverage the performance benefits of Concurrent Thread-Stack Processing in ZGC.
Hidden Classes: Reduction in Memory and Class-Loading Overhead
Java 15 introduces Hidden Classes, a feature that enables the creation of classes that are not discoverable by the class loaders. This can offer significant performance improvements by reducing the memory and class-loading overhead associated with dynamically generated classes. Hidden Classes are particularly beneficial for frameworks and libraries that rely heavily on dynamic class generation, such as mockito and byte-buddy.
// Creating a Hidden Class in Java 15
class HiddenClassExample {
public void greet() {
System.out.println("Hello, Hidden Class!");
}
}
The introduction of Hidden Classes simplifies the process of creating classes at runtime without unnecessary exposure, thereby contributing to improved performance and efficiency in class loading.
Foreign-Memory Access API: Efficient Access to Native Memory
Java 15 features the Foreign-Memory Access API, which provides a more efficient and safe means of accessing native memory outside the Java heap. This feature allows direct, structured access to native memory, circumventing the overhead of Java Native Interface (JNI) and providing better control over memory layout and access.
// Using Foreign-Memory Access API in Java 15
MemorySegment segment = MemorySegment.allocateNative(1024);
ByteBuffer buffer = segment.asByteBuffer();
buffer.putInt(0, 42);
With the Foreign-Memory Access API, developers can perform operations on native memory with ease and efficiency, enhancing the performance of applications that interact with native libraries and systems.
Deprecation of RMI Activation System
In Java 15, the RMI (Remote Method Invocation) Activation System has been deprecated. This feature, which allowed objects to be activated in response to client requests, has faced declining usage and presented security challenges. By deprecating the RMI Activation System, Java aims to streamline the platform and remove outdated components, improving performance and security.
Summary
Java 15 introduces several key performance improvements, including Concurrent Thread-Stack Processing in ZGC, Hidden Classes for reducing memory and class-loading overhead, and the Foreign-Memory Access API for efficient access to native memory. Additionally, the deprecation of the RMI Activation System reflects Java's commitment to enhancing performance and security by eliminating outdated components. By leveraging these performance enhancements, developers can build faster, more efficient Java applications.
To stay updated with the latest features and improvements in Java, visit the Official Java SE Platform website.