AspectJ and Spring Integration: Solving Cross-Cutting Concerns
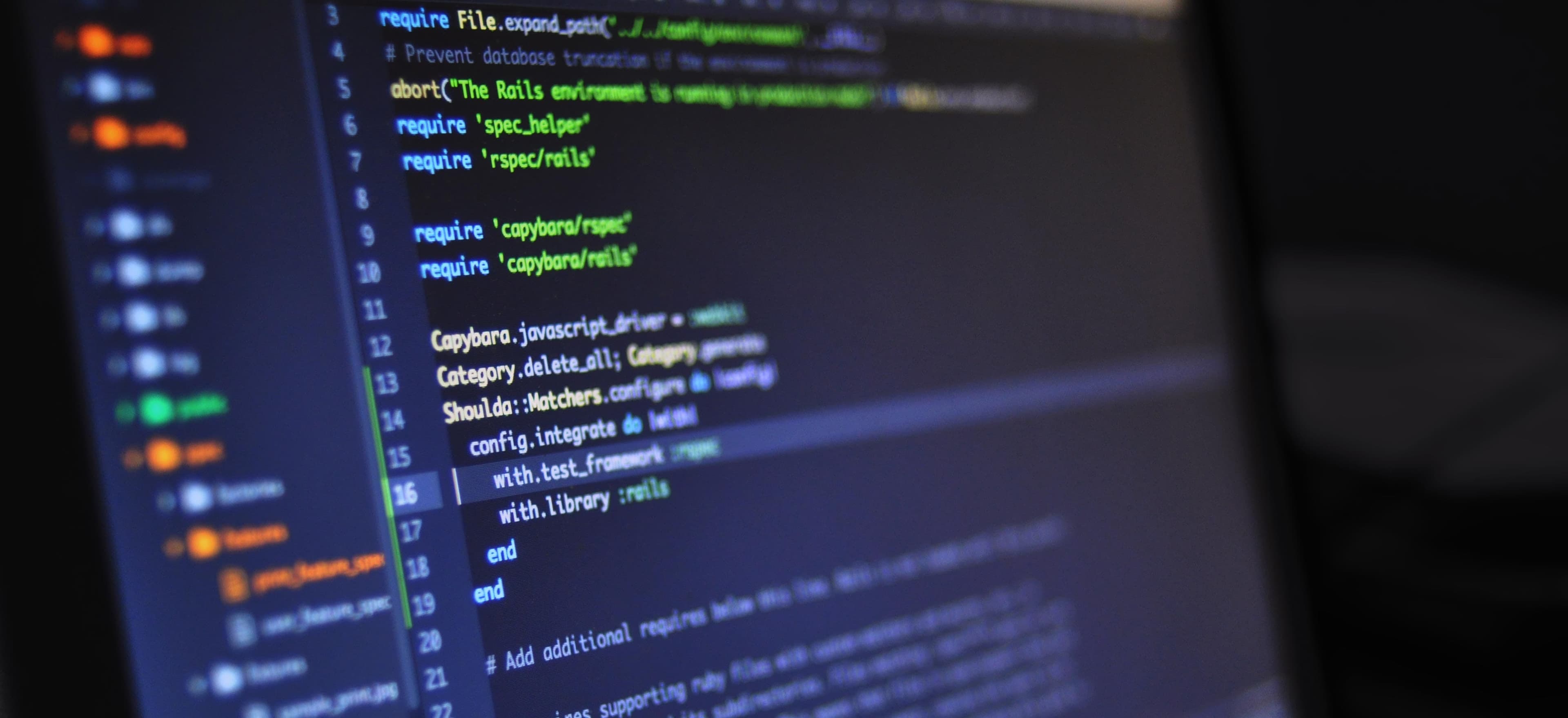
- Published on
AspectJ and Spring Integration: Solving Cross-Cutting Concerns
Aspect-Oriented Programming (AOP) is a powerful technique to modularize cross-cutting concerns in software development. In Java, AspectJ is a widely used and mature AOP framework that seamlessly integrates with the Spring framework. This blog post will delve into how AspectJ can be integrated with Spring to tackle cross-cutting concerns, providing a clear understanding of the concepts and practical implementation.
Understanding Cross-Cutting Concerns
Cross-cutting concerns are aspects of a program that affect multiple modules and lead to code scattering and tangling. Examples of cross-cutting concerns include logging, security, transaction management, and caching. These concerns do not fit neatly into a single module and are often spread across the application, leading to code duplication and reduced maintainability. AOP addresses this issue by providing a modular way to encapsulate cross-cutting functionalities.
AspectJ: A Powerful AOP Framework
AspectJ is a mature and feature-rich AOP framework for Java. It extends the Java programming language with new constructs that enable clean modularization of cross-cutting concerns. AspectJ introduces the concept of aspects, which allows developers to encapsulate cross-cutting behavior and apply it to the relevant join points in the codebase.
With AspectJ, developers can declare pointcuts to specify join points in the code where cross-cutting behavior should be applied. They can then define advices, such as before advice, after advice, and around advice, to specify the actions to be taken at those join points. This approach separates the cross-cutting concerns from the business logic, leading to cleaner and more maintainable code.
Integrating AspectJ with Spring
Spring is a popular framework for building enterprise Java applications. It provides comprehensive support for dependency injection, transaction management, and various other enterprise features. Spring AOP provides a limited subset of AOP features compared to AspectJ, but it is integrated seamlessly with the Spring IoC container.
By integrating AspectJ with Spring, developers can leverage the full power of AspectJ to address cross-cutting concerns in a Spring application. This integration allows for a more expressive and flexible way of defining aspects and applying them to Spring beans.
Practical Implementation
Let's consider a practical example of integrating AspectJ with Spring to solve a common cross-cutting concern: logging. We want to log method entry and exit for a specific service class in our Spring application.
Creating an AspectJ Aspect
We start by creating an AspectJ aspect to encapsulate the logging behavior:
import org.aspectj.lang.annotation.*;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.MyService.*(..))")
public void logMethodEntry() {
System.out.println("Entering method");
}
@After("execution(* com.example.service.MyService.*(..))")
public void logMethodExit() {
System.out.println("Exiting method");
}
}
In this aspect, we define two advices: logMethodEntry
and logMethodExit
. The @Before
and @After
annotations specify the join points at which these advices should be applied. In this case, the advices will be applied before and after the execution of methods in the MyService
class.
Applying the Aspect to a Spring Bean
Next, we apply the LoggingAspect
to the MyService
bean in the Spring configuration:
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
@Bean
public MyService myService() {
return new MyService();
}
@Bean
public LoggingAspect loggingAspect() {
return new LoggingAspect();
}
}
The @EnableAspectJAutoProxy
annotation enables support for using AspectJ annotations to define aspects in the Spring application context. We then declare the MyService
bean and the LoggingAspect
bean, allowing the aspect to be woven into the MyService
bean.
Testing the Integration
With the aspect configured and applied to the Spring bean, we can now test the logging behavior:
public class Main {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
MyService myService = context.getBean(MyService.class);
myService.doSomething();
}
}
When we run the Main
class, we should see the following output:
Entering method
Doing something
Exiting method
The Bottom Line
In this blog post, we explored how AspectJ can be integrated with the Spring framework to tackle cross-cutting concerns in Java applications. We discussed the concept of cross-cutting concerns, the features of AspectJ, and the seamless integration of AspectJ with Spring.
By leveraging AspectJ's powerful AOP capabilities, developers can cleanly modularize cross-cutting concerns and apply them to Spring beans, leading to more maintainable and readable code. The practical example demonstrated how easy it is to integrate AspectJ with Spring and solve a common cross-cutting concern.
AspectJ's integration with Spring provides a robust solution for addressing cross-cutting concerns in enterprise Java applications, allowing developers to focus on the core business logic while seamlessly applying aspects to the codebase.
For more in-depth information on AspectJ and Spring integration, refer to the official documentation:
Start harnessing the power of AspectJ and Spring integration to streamline your Java application development and promote clean, modular code organization.