Handling Command Line Options with Getopt4j
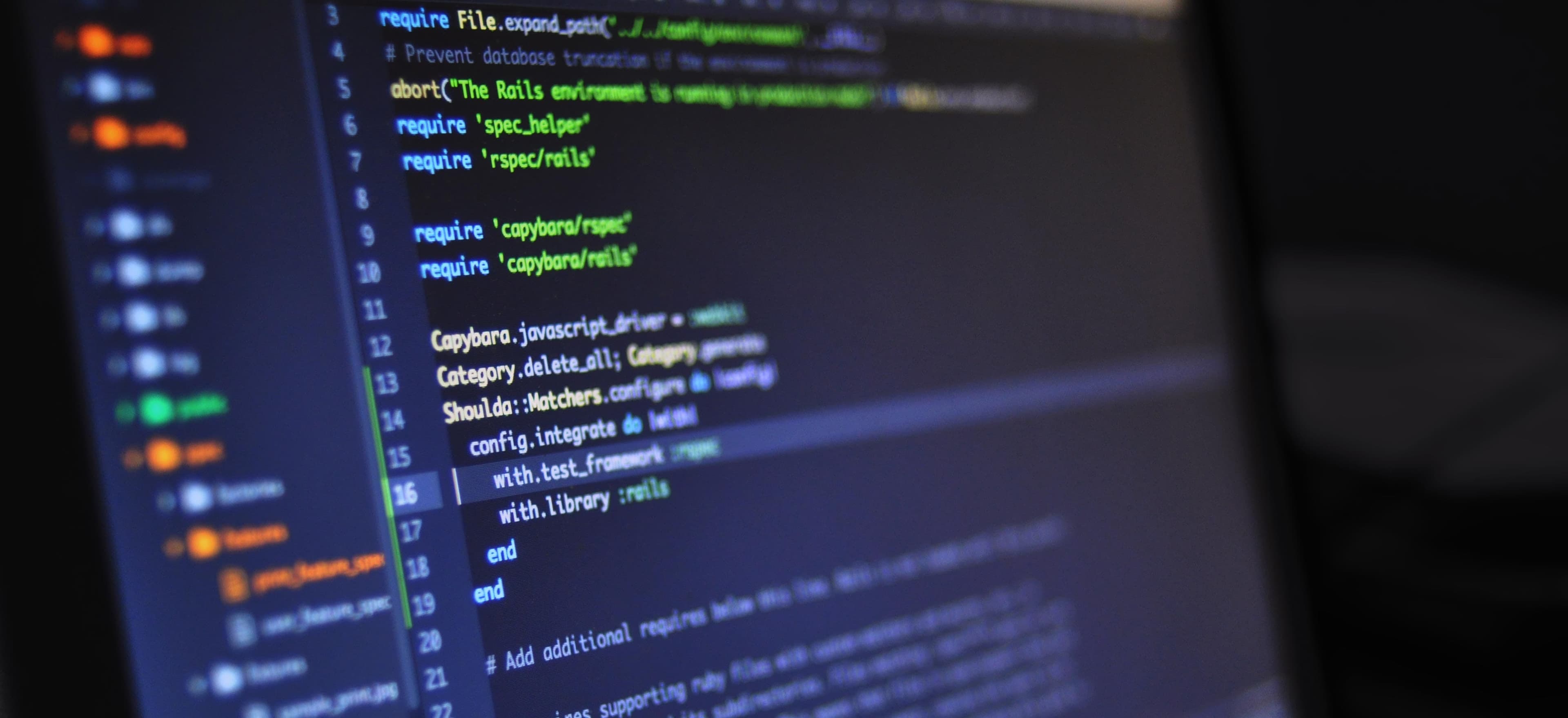
- Published on
Handling Command Line Options with Getopt4j
When developing a Java application, it is often necessary to handle command line options. This allows users to customize the behavior of the application, making it more versatile and user-friendly. In this blog post, we will explore how to handle command line options in Java using the Getopt4j library. Getopt4j is a command line parser for Java, inspired by the GNU getopt() function.
What is Getopt4j?
Getopt4j is a Java library that provides an easy way to parse command line options in Java applications. It supports both short and long option formats, making it flexible for users to input their preferences. With Getopt4j, you can define the options your application supports, parse the command line arguments, and retrieve the values entered by the user.
Adding Getopt4j to Your Project
Before we dive into using Getopt4j, let's first add the library to our project. You can include Getopt4j as a dependency using a build tool like Maven or Gradle.
For Maven, add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.kohsuke</groupId>
<artifactId>getopt4j</artifactId>
<version>1.0.3</version>
</dependency>
If you're using Gradle, add the following to your build.gradle
file:
dependencies {
implementation 'org.kohsuke:getopt4j:1.0.3'
}
With the dependency added, you can start using Getopt4j to handle command line options in your Java application.
Using Getopt4j
Let's consider a hypothetical scenario where we have a Java application that accepts command line options for customization. In this example, we want to allow the user to specify a file to process and provide a verbose mode for extra output. We'll go through the steps to use Getopt4j to achieve this functionality.
Defining Options
The first step is to define the options that our application supports. We'll use Getopt4j to define the file
and verbose
options.
import gnu.getopt.Getopt;
public class CommandLineApp {
public static void main(String[] args) {
Getopt opts = new Getopt("CommandLineApp", args, "f:v");
int c;
String file = null;
boolean verbose = false;
while ((c = opts.getopt()) != -1) {
switch (c) {
case 'f':
file = opts.getOptarg();
break;
case 'v':
verbose = true;
break;
default:
// Handle unknown options
break;
}
}
// Process the file and verbose flag
processFile(file, verbose);
}
private static void processFile(String file, boolean verbose) {
// Implementation to process the file with verbose mode
}
}
In the code above, we create a Getopt
instance and define the supported options as characters (f
for file and v
for verbose). We then iterate through the command line arguments and set the file
and verbose
variables based on the user input.
Running the Application
To run the application with the defined options, the user would input something like:
java CommandLineApp -f sample.txt -v
This command specifies the file sample.txt
using the -f
option and enables verbose mode using the -v
option.
Why Use Getopt4j?
Now, you might wonder: Why use Getopt4j instead of manually parsing command line arguments?
Getopt4j simplifies the process of parsing command line options, providing a clean and efficient way to handle user input. With Getopt4j, you don't have to reinvent the wheel by writing your own parsing logic, error handling, and option validation. The library takes care of these aspects, allowing you to focus on the core functionality of your application.
Additionally, with Getopt4j, you can easily define both short and long options, making it more user-friendly and aligned with standard command line conventions.
A Final Look
In this blog post, we explored how to handle command line options in Java using the Getopt4j library. We covered the basics of defining options, parsing user input, and utilizing the parsed values in the application. By incorporating Getopt4j, you can enhance the usability of your Java applications by allowing users to customize the behavior via command line options.
Getopt4j simplifies the process of handling command line options, making your application more user-friendly and intuitive. Whether it's defining simple options like file paths or enabling verbose modes, Getopt4j provides a convenient way to interact with your Java applications via the command line.
So why not give Getopt4j a try for your next Java command line application? You may find that it significantly streamlines the process and enhances the overall user experience.
Incorporating Getopt4j into your projects can save valuable development time and effort, ensuring seamless handling of command line options in your Java applications. Happy coding!
By incorporating Getopt4j into your projects, you can save valuable development time and effort, ensuring seamless handling of command line options in your Java applications. Happy coding!