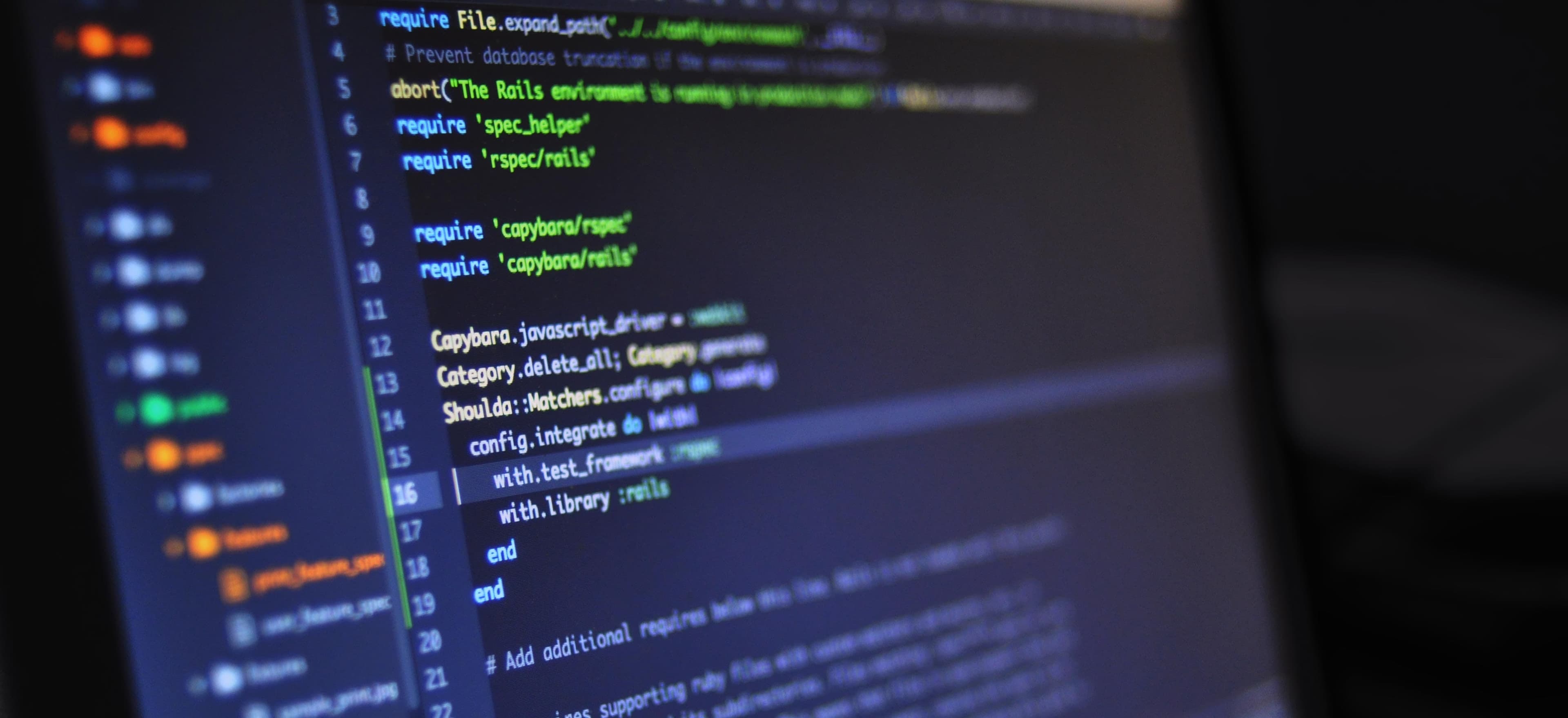
- Published on
Choosing the Right Database: SQL or NoSQL?
When developing a Java application, the choice of the right database is crucial. Each type of database, SQL and NoSQL, has its strengths and weaknesses, and understanding them is essential for making an informed decision. In this article, we will dive into the characteristics of SQL and NoSQL databases to help you make the right choice for your Java application.
Understanding SQL and NoSQL
SQL Databases
Structured Query Language (SQL) databases are relational databases that use a structured schema and are based on tables. They are known for their ACID (Atomicity, Consistency, Isolation, Durability) compliance and are suitable for applications with complex queries and transactions, such as financial systems and e-commerce platforms.
NoSQL Databases
NoSQL databases, on the other hand, are non-relational databases that provide flexible schemas and are designed to handle large volumes of unstructured data. They are known for their high scalability, fault tolerance, and eventual consistency, making them ideal for handling big data, real-time web applications, and IoT (Internet of Things) systems.
When to Choose SQL
If your Java application requires complex queries, data integrity, and ACID compliance, a SQL database such as MySQL, PostgreSQL, or Oracle would be a suitable choice. SQL databases are well-suited for applications that deal with structured data and need to maintain strict data consistency.
Consider using SQL databases when:
- You need to maintain complex relationships between entities.
- Your application requires high transactional integrity.
- Data security and ACID compliance are critical for your application.
When to Choose NoSQL
NoSQL databases like MongoDB, Cassandra, and Redis are ideal for Java applications that handle semi-structured or unstructured data, require horizontal scalability, and prioritize high availability and partition tolerance (as per the CAP theorem).
Consider using NoSQL databases when:
- Your application needs to handle large volumes of rapidly changing data.
- Scalability and high availability are top priorities.
- Your data structure is expected to evolve over time.
Integration with Java
When integrating a database with a Java application, both SQL and NoSQL databases offer excellent support through JDBC (Java Database Connectivity) for SQL databases and native Java APIs for NoSQL databases. Remember to consider the maturity of the database drivers and the availability of community support when making your decision.
Example: Integrating MySQL with a Java Application
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLConnector {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "root";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, username, password)) {
System.out.println("Connected to MySQL database!");
} catch (SQLException e) {
System.out.println("Connection failed! Error: " + e.getMessage());
}
}
}
In this example, we utilize JDBC to connect to a MySQL database from a Java application. The DriverManager.getConnection()
method establishes a connection using the database URL, username, and password. This demonstrates the simplicity and ease of integrating SQL databases with Java.
Example: Integrating MongoDB with a Java Application
import com.mongodb.client.MongoClients;
import com.mongodb.client.MongoClient;
import com.mongodb.client.MongoDatabase;
public class MongoDBConnector {
public static void main(String[] args) {
try (MongoClient mongoClient = MongoClients.create("mongodb://localhost:27017")) {
MongoDatabase database = mongoClient.getDatabase("mydatabase");
System.out.println("Connected to MongoDB database!");
} catch (Exception e) {
System.out.println("Connection failed! Error: " + e.getMessage());
}
}
}
In this example, we use the MongoDB Java driver to connect to a MongoDB database. With the MongoClients.create()
method, we establish a connection to the MongoDB server and retrieve the database for further operations. This showcases the straightforward integration of NoSQL databases with Java applications.
Scalability and Performance Considerations
When evaluating the scalability and performance aspects of SQL and NoSQL databases for your Java application, it's essential to consider factors such as data volume, query complexity, read and write patterns, and anticipated growth.
-
SQL Database Scalability: SQL databases can scale vertically by increasing the server's processing power and storage. While this provides robust transactional support, it may have limitations in handling massive concurrent write operations and distributed architectures.
-
NoSQL Database Scalability: NoSQL databases enable horizontal scalability by adding more nodes to distribute the data load. They excel in handling high volumes of read and write operations and are well-suited for distributed and cloud-based environments.
Security and Data Consistency
Data security and consistency are critical aspects to consider when choosing a database for your Java application.
-
SQL Database Security: SQL databases offer robust security features such as role-based access control, encrypted connections, and compliance with industry standards like GDPR and HIPAA.
-
NoSQL Database Security: NoSQL databases provide security features but may require additional effort in implementing access control and data encryption. However, they excel in handling large-scale distributed systems and are often used for real-time processing of big data.
Making the Decision
In conclusion, the choice between SQL and NoSQL databases for your Java application boils down to the specific requirements of your project.
If your application demands strict data integrity, complex queries, and a well-defined schema, an SQL database is the way to go. On the other hand, if you anticipate handling large volumes of rapidly changing data, require high scalability, and prioritize flexibility in data modeling, a NoSQL database would be a better fit.
By carefully evaluating the characteristics, integration ease, scalability, security, and data consistency of SQL and NoSQL databases, you can make an informed decision that aligns with your Java application's current and future needs.
Remember, the database you choose will play a significant role in shaping the performance, scalability, and maintenance of your Java application, so choose wisely.
Make your choice based on your application's specific requirements, and you'll set the foundation for a robust and efficient database-driven Java application.