Concatenating Columns in SparkR Dataframe
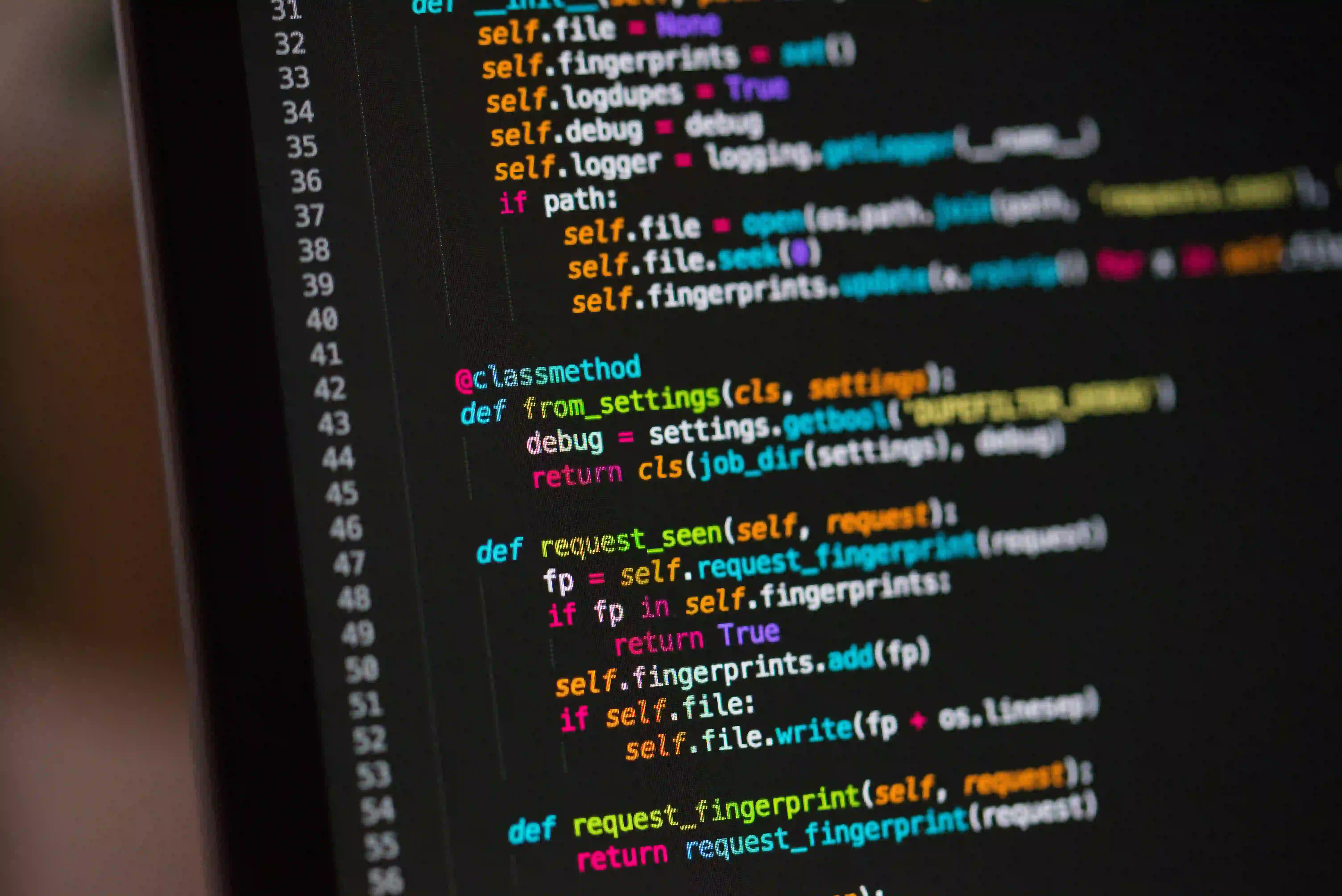
How to Concatenate Columns in SparkR Dataframe
In this post, we will explore the process of concatenating columns in a SparkR dataframe. Apache Spark is an open-source distributed computing system that provides an interface for programming entire clusters with implicit data parallelism and fault tolerance. In SparkR, we can perform various operations on dataframes, including concatenating columns.
Understanding the Problem
In many data analysis and data manipulation scenarios, there is a need to combine or concatenate the values of two or more columns into a single column. This is a common requirement when dealing with structured data, such as in data preprocessing or feature engineering tasks.
Approach
This post assumes a basic understanding of Apache Spark and SparkR. We'll focus on the specific operation of concatenating columns in a SparkR dataframe.
Let's begin by looking at how we can achieve this using SparkR.
Code Implementation
To demonstrate concatenating columns in a SparkR dataframe, we first need to set up a SparkR session and create a sample dataframe.
# Load the SparkR library
library(SparkR)
# Initialize Spark session
sparkR.session()
# Create a sample dataframe
data <- data.frame(id = c(1, 2, 3),
first_name = c("John", "Alice", "Bob"),
last_name = c("Doe", "Smith", "Johnson"))
df <- createDataFrame(data)
In the above code, we have initialized a Spark session and created a sample dataframe df
with columns id
, first_name
, and last_name
.
Now, let's proceed to concatenate the first_name
and last_name
columns into a new column full_name
.
# Concatenate columns
df <- withColumn(df, "full_name", paste(df$first_name, df$last_name, sep = " "))
Here, we use the withColumn
function to add a new column full_name
to the dataframe df
. We combine the values of first_name
and last_name
using the paste
function, and specify a space as the separator.
Explanation
The withColumn
function in SparkR is used to add a new column to a dataframe based on the manipulation of existing columns. In this case, we are utilizing it to concatenate the values of first_name
and last_name
into a new column full_name
.
The paste
function is a base R function that concatenates its arguments element-wise. We use it here to combine the values of first_name
and last_name
, with a space as the separator.
Key Takeaways
In this post, we have explored the process of concatenating columns in a SparkR dataframe. By leveraging the withColumn
function and the paste
function from base R, we were able to combine the values of two columns into a new column. This operation is essential in various data preprocessing and manipulation tasks and can be seamlessly performed in SparkR.
Now you have the knowledge to concatenate columns in a SparkR dataframe with ease. Try incorporating this into your SparkR data manipulation workflows for efficient and streamlined data processing.
For further reading, check out the official SparkR documentation to delve deeper into SparkR dataframe operations and transformations.