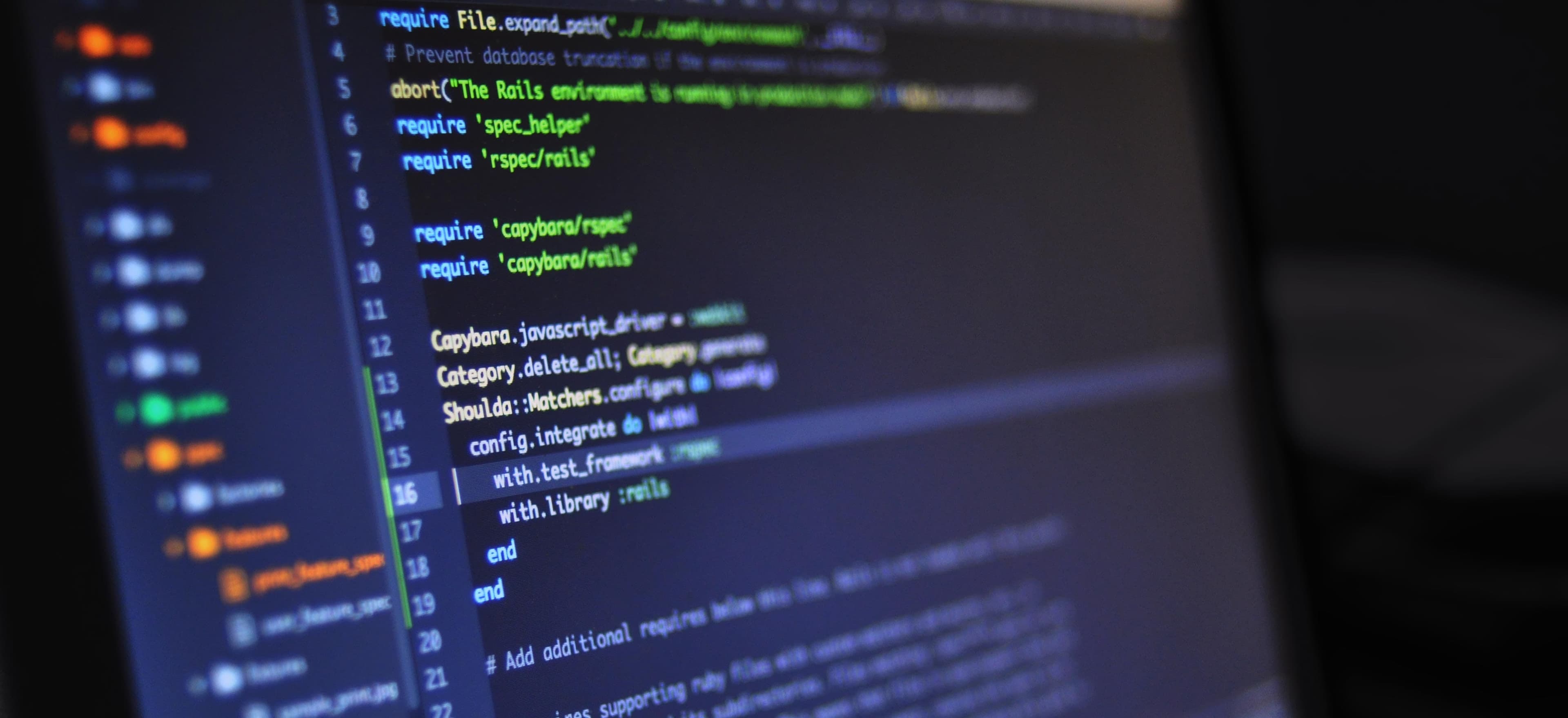
- Published on
Best Practices for Managing Sensitive Data in Microservices
When working with microservices, it's crucial to handle sensitive data appropriately to ensure the security and integrity of the system. In this article, we'll explore the best practices for managing sensitive data in microservices, focusing on Java-based microservices. We'll discuss various strategies and tools that can help in securely handling passwords, API keys, database credentials, and other sensitive information.
1. Use Environment Variables
When dealing with sensitive data in microservices, it's essential to avoid hardcoding any credentials or secrets directly into the code. One common approach is to utilize environment variables to inject sensitive information into the microservices at runtime. In Java, you can access these environment variables using System.getenv("ENV_VARIABLE_NAME")
.
String dbUsername = System.getenv("DB_USERNAME");
String dbPassword = System.getenv("DB_PASSWORD");
By using environment variables, you can separate the configuration from the codebase, making it easier to manage different configurations for various environments such as development, testing, and production.
2. Leverage Vault or Key Management Services
Another effective approach is to use a dedicated tool like HashiCorp Vault or a cloud-based Key Management Service (KMS) to store and manage sensitive data securely. These tools provide encryption, access control, and audit trails for managing secrets. In Java, you can use libraries or SDKs provided by these tools to integrate with your microservices.
// Sample code for integrating with Vault
VaultConfig config = new VaultConfig().address("https://vault.example.com").token("your-token-here").build();
Vault vault = new Vault(config);
String apiToken = vault.logical().read("secret/api").getData().get("token");
By utilizing these tools, you can centralize the management of secrets, rotate credentials regularly, and enforce access policies, improving the overall security posture of your microservices.
3. Encrypt Sensitive Data
In scenarios where you need to store sensitive data in configuration files or databases, it's essential to encrypt the information to prevent unauthorized access. Java provides strong cryptographic support through the Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE). You can leverage these APIs to encrypt and decrypt sensitive data within your microservices.
// Example of encrypting sensitive data using JCA/JCE
String originalData = "sensitive information";
byte[] encryptedData = encryptionService.encrypt(originalData);
Always remember to securely manage the encryption keys and consider the trade-offs between security and performance when implementing encryption within your microservices.
4. Secure Communication with TLS/SSL
When microservices need to communicate with each other or external systems, it's vital to ensure the security of data in transit. Transport Layer Security (TLS) or its predecessor, Secure Sockets Layer (SSL), can provide a secure communication channel by encrypting the data exchanged between services. In Java, you can configure TLS/SSL for your microservices using tools like Java Keytool for certificate management and libraries like Apache HttpClient for secure HTTP communication.
// Configuring TLS/SSL for secure communication
CloseableHttpClient httpClient = HttpClients.custom().setSSLContext(SSLContext.getDefault()).build();
HttpGet request = new HttpGet("https://api.example.com/data");
CloseableHttpResponse response = httpClient.execute(request);
By implementing TLS/SSL, you can ensure that sensitive data remains encrypted during transit, preventing eavesdropping and unauthorized access.
Closing the Chapter
In conclusion, managing sensitive data in microservices requires a comprehensive approach that encompasses environment variables, dedicated secret management tools, encryption, and secure communication protocols. By following these best practices, you can enhance the security of your Java-based microservices and mitigate the risks associated with handling sensitive information.
Remember, as you handle sensitive data, always stay updated with the best practices and security standards, such as OWASP Top Ten and NIST guidelines. Keep learning and adapting to the evolving landscape of security in microservice architectures.
Implementing these best practices from the beginning will save you from potential security breaches and provide a solid foundation for the secure operation of your microservices.
Now, go ahead, implement these best practices, and ensure the security of your microservices!