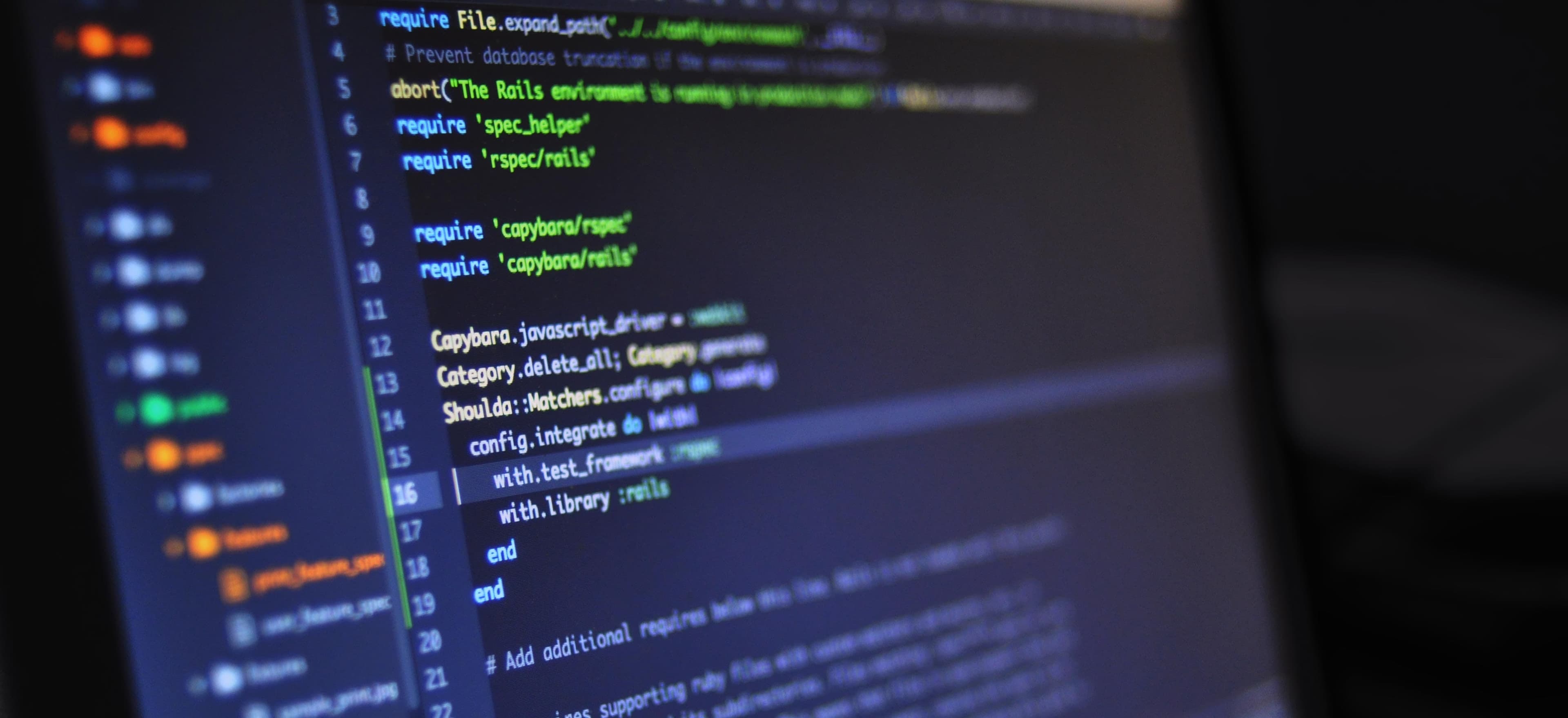
- Published on
Creating Reusable FXML Components in Java
In Java development, creating reusable components is essential for building maintainable and efficient applications. When working with JavaFX, developers often use FXML to define the user interface, and it's crucial to create reusable FXML components to promote modularity and reusability within the application.
In this article, we will explore the process of creating reusable FXML components in Java, focusing on the best practices and techniques to achieve this goal.
Why Reusable FXML Components?
Before diving into the technical details, it's important to understand the significance of creating reusable FXML components. By encapsulating specific UI elements and their associated behavior into reusable components, developers can achieve the following benefits:
-
Modularity: Reusable components promote a modular design, making it easier to manage and maintain the codebase.
-
Code Reusability: Developers can reuse the same component across different parts of the application, reducing code duplication and enhancing consistency.
-
Ease of Maintenance: When a change is required, having reusable components allows developers to make updates in a centralized manner, thereby reducing the maintenance overhead.
Setting Up the Project
Let's start by setting up a simple JavaFX project using Maven, which will serve as the basis for creating our reusable FXML components.
Maven Project Structure
reusable-fxml-components
├── src
│ ├── main
│ │ ├── java
│ │ └── resources
│ └── test
│ └── java
└── pom.xml
In the pom.xml
file, we include the necessary dependencies for JavaFX:
<dependencies>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-controls</artifactId>
<version>16</version>
</dependency>
<dependency>
<groupId>org.openjfx</groupId>
<artifactId>javafx-fxml</artifactId>
<version>16</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.openjfx</groupId>
<artifactId>javafx-maven-plugin</artifactId>
<version>0.0.7</version>
<configuration>
<mainClass>your.main.class.Here</mainClass>
</configuration>
</plugin>
</plugins>
</build>
Now that we have our project structure set up, we can proceed with creating our reusable FXML components.
Creating a Reusable FXML Component
Let's say we want to create a custom button component that we can reuse throughout our JavaFX application.
FXML Structure
First, we define the FXML structure for our custom button component. Create a new FXML file named CustomButton.fxml
in the resources
directory:
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Button?>
<?import javafx.scene.layout.VBox?>
<VBox xmlns="http://javafx.com/javafx"
xmlns:fx="http://javafx.com/fxml"
fx:controller="com.example.CustomButtonController">
<Button fx:id="customButton" text="Custom Button" onAction="#handleButtonClick"/>
</VBox>
In the FXML structure above, we have a VBox
layout containing a Button
with an fx:id
of customButton
and an onAction
event handler.
Controller Class
Next, we create the controller class CustomButtonController
for our custom button component:
package com.example;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Button;
public class CustomButtonController {
@FXML
private Button customButton;
@FXML
private void handleButtonClick(ActionEvent event) {
System.out.println("Custom button clicked");
}
}
In the controller class, we inject the Button
using the @FXML
annotation, and we define the handleButtonClick
method to handle the button click event.
Using the Reusable Component
To use our custom button component in another FXML file or Java code, we simply include it using the fx:include
tag in the parent FXML or instantiate it in the Java code.
Including in Another FXML File
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.layout.AnchorPane?>
<?import javafx.scene.layout.VBox?>
<AnchorPane xmlns="http://javafx.com/javafx"
xmlns:fx="http://javafx.com/fxml">
<fx:include source="CustomButton.fxml"/>
<!-- Other UI elements -->
</AnchorPane>
Instantiating in Java Code
package com.example;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Scene;
import javafx.stage.Stage;
import java.io.IOException;
public class MainApp extends Application {
@Override
public void start(Stage stage) throws IOException {
FXMLLoader loader = new FXMLLoader(getClass().getResource("CustomButton.fxml"));
VBox customButton = loader.load();
// Add customButton to the scene and perform other necessary operations
Scene scene = new Scene(customButton);
stage.setScene(scene);
stage.show();
}
}
The Bottom Line
Creating reusable FXML components in JavaFX facilitates the development of maintainable and modular applications. By encapsulating UI elements and their behavior into reusable components, developers can streamline development efforts and enhance code reusability.
In this article, we've walked through the process of creating a reusable FXML component, and demonstrated how to use it in other parts of the application. By following these best practices, developers can effectively leverage the power of reusable components in JavaFX development.
Now that you have a foundational understanding, you can delve deeper into complex FXML component creation and integration, leading to the development of robust and efficient JavaFX applications.
Happy coding!