Troubleshooting Common Issues with Hibernate 4 in Spring
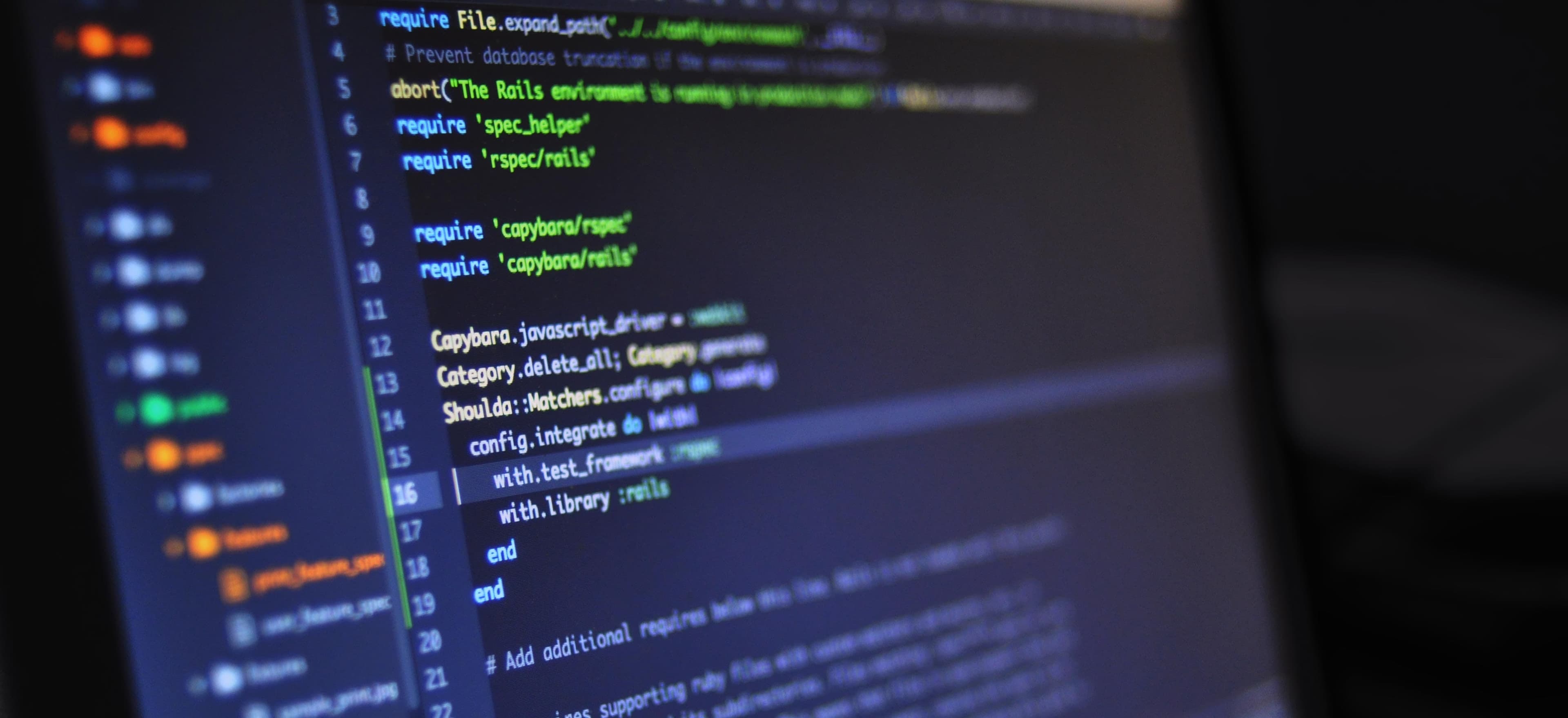
- Published on
Troubleshooting Common Issues with Hibernate 4 in Spring
When working with Hibernate 4 in a Spring application, you may encounter various issues related to configuration, mapping, transactions, and performance. In this blog post, we'll discuss some common problems that developers face when using Hibernate 4 with Spring and how to troubleshoot and resolve them.
Issue 1: SessionFactory Bean Configuration
One of the most common issues when using Hibernate 4 in a Spring application is misconfiguring the SessionFactory bean. It's crucial to ensure that the SessionFactory is set up correctly to establish a connection with the database and provide the necessary configuration for Hibernate.
Troubleshooting Steps:
Check the Hibernate configuration file (hibernate.cfg.xml or hibernate.properties) for any errors in database connection settings, dialect, or mapping files.
Ensure that the dataSource bean is properly configured in the Spring configuration file, and it is being injected into the LocalSessionFactoryBean.
@Configuration
@EnableTransactionManagement
public class HibernateConfig {
@Autowired
private DataSource dataSource;
@Bean
public LocalSessionFactoryBean sessionFactory() {
LocalSessionFactoryBean sessionFactory = new LocalSessionFactoryBean();
sessionFactory.setDataSource(dataSource);
// Other Hibernate properties
return sessionFactory;
}
}
Issue 2: Mapping Entities
Another common issue arises when mapping entities in Hibernate 4. This includes incorrect annotations, missing mappings, or improper use of associations such as @OneToOne, @OneToMany, @ManyToOne, or @ManyToMany.
Troubleshooting Steps:
Double-check the entity classes and their annotations for correctness. Ensure that the primary key is properly annotated using @Id and that relationships are defined accurately.
Verify that the hbm2ddl.auto property is set appropriately to create/update tables based on the entity mappings.
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
// Other fields and mappings
}
Issue 3: Transaction Management
Managing transactions in a Spring application with Hibernate 4 is crucial for ensuring data integrity and consistency. Issues with transaction management can lead to data anomalies and runtime errors.
Troubleshooting Steps:
Ensure that the @Transactional annotation is used correctly on service methods that interact with the database to demarcate transactions.
Check that the PlatformTransactionManager is configured properly in the Spring configuration file and injected into the transactional beans.
@Service
@Transactional
public class ProductService {
@Autowired
private ProductRepository productRepository;
// Transactional method
public void saveProduct(Product product) {
productRepository.save(product);
}
}
Issue 4: Performance Optimization
Hibernate 4 is powerful, but inefficient queries and lazy loading issues can impact performance. It's essential to optimize data retrieval and processing to avoid performance bottlenecks.
Troubleshooting Steps:
Use proper indexing on database columns frequently involved in queries to improve query performance.
Avoid N+1 query issues by using fetch joins or entity graphs to fetch related entities eagerly when needed.
public List<Order> getOrdersWithProducts() {
return sessionFactory.getCurrentSession()
.createQuery("select o from Order o join fetch o.products", Order.class)
.getResultList();
}
Issue 5: Logging and Debugging
Logging is indispensable when troubleshooting issues in Hibernate 4. It helps in understanding the generated SQL queries, configuration, and runtime behavior.
Troubleshooting Steps:
Configure the logging level for Hibernate and Spring Data Source to debug or trace to capture detailed information.
Use tools like p6spy or enable show_sql property to log SQL statements and parameters.
<logger name="org.hibernate">
<level value="debug"/>
</logger>
<logger name="org.springframework.jdbc.core">
<level value="debug"/>
</logger>
My Closing Thoughts on the Matter
By addressing these common issues with Hibernate 4 in Spring applications and following the troubleshooting steps outlined, developers can ensure smooth integration and optimal performance. Additionally, staying updated with the latest releases and best practices for Hibernate and Spring framework will contribute to a robust and efficient application.
For further reading, refer to the official Hibernate documentation and Spring Framework reference.