Managing Named Queries in JPA
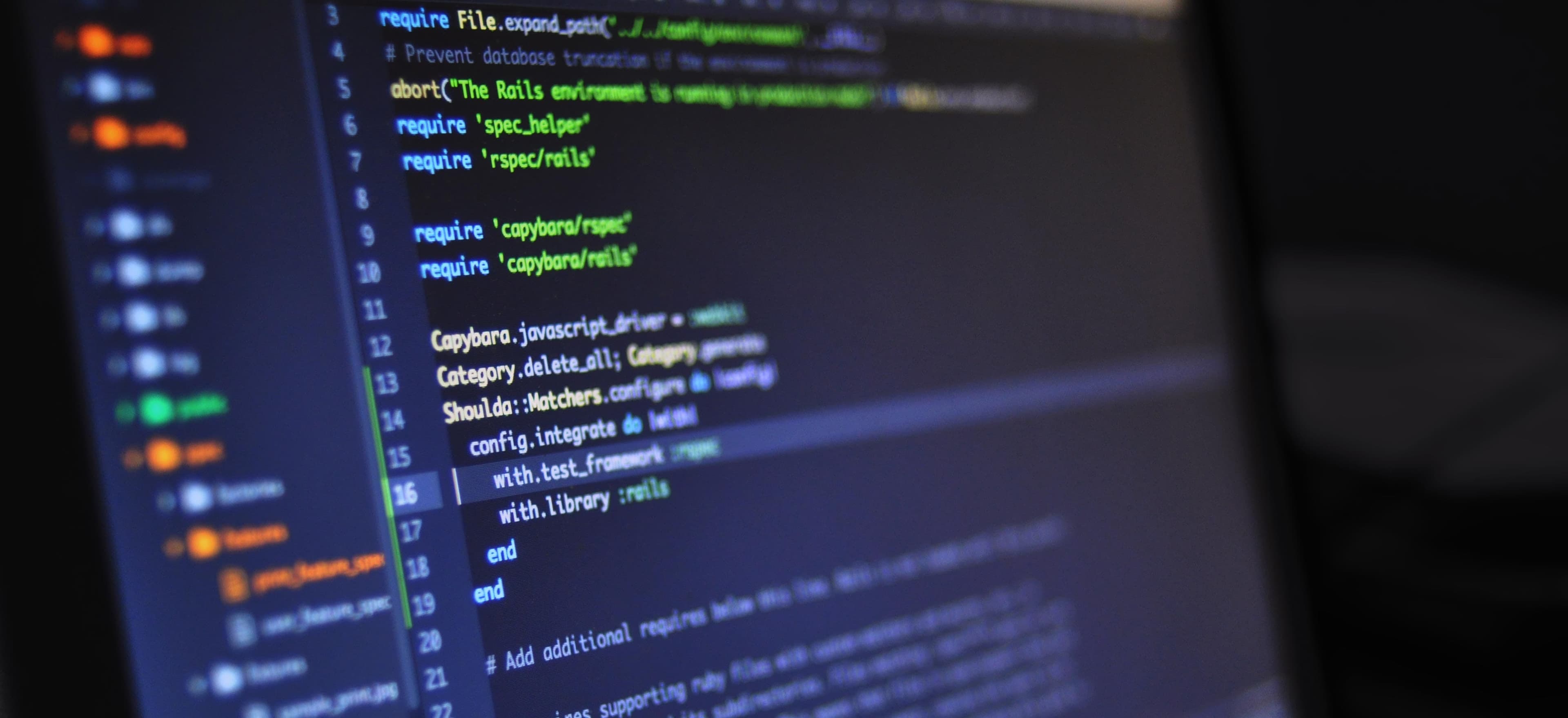
- Published on
Understanding Named Queries in JPA
In Java Persistence API (JPA), named queries play a crucial role in managing and executing queries. Named queries allow developers to define a query with a name and then reference that name when performing database operations. This not only enhances code maintainability but also promotes reusability.
Declaring a Named Query
To declare a named query in JPA, you can use the @NamedQuery
annotation. This annotation is usually placed on the entity class and includes the name of the query and the query string.
Here's an example of declaring a named query in an entity class:
@Entity
@NamedQuery(
name = "findAllEmployees",
query = "SELECT e FROM Employee e"
)
public class Employee {
// entity attributes and methods
}
In the above example, the named query "findAllEmployees" has been defined to retrieve all Employee entities from the database.
Executing a Named Query
Once a named query is declared, it can be executed using the createNamedQuery
method of the EntityManager
interface. This method takes the name of the named query and returns a TypedQuery
instance, which can then be used to set parameters and retrieve results.
Here's how to execute the "findAllEmployees" named query:
TypedQuery<Employee> query = entityManager.createNamedQuery("findAllEmployees", Employee.class);
List<Employee> employees = query.getResultList();
In this code snippet, entityManager
is an instance of the EntityManager
interface, and we use the createNamedQuery
method to obtain a TypedQuery<Employee>
for the "findAllEmployees" named query. We then execute the query and retrieve the results as a list of Employee
entities.
Benefits of Named Queries
Improved Code Readability and Maintainability
Using named queries makes the code more readable by abstracting the query logic into a named reference. This improves code maintainability as any changes to the query can be made in a central location.
Performance Optimization
Named queries are parsed and validated at deployment time, which can help optimize performance by minimizing the time spent parsing and validating queries at runtime.
Dynamic Named Queries
In some cases, you may want to define named queries with dynamic parameters. This can be achieved using named query parameters.
Here's an example of a dynamic named query with a parameter:
@Entity
@NamedQuery(
name = "findEmployeeById",
query = "SELECT e FROM Employee e WHERE e.id = :id"
)
public class Employee {
// entity attributes and methods
}
In the above example, the ":id" in the query string represents a named parameter. When executing the named query, the parameter value can be set using the setParameter
method of the TypedQuery
interface.
TypedQuery<Employee> query = entityManager.createNamedQuery("findEmployeeById", Employee.class);
query.setParameter("id", 123);
Employee employee = query.getSingleResult();
In this code snippet, we set the value of the "id" parameter to 123 before executing the named query "findEmployeeById" to retrieve a specific Employee
entity.
In Conclusion, Here is What Matters
Named queries in JPA are powerful tools for managing and executing queries in a Java application. By using named queries, developers can improve code readability, maintainability, and performance. Additionally, dynamic named queries provide flexibility in handling parameterized queries.
Incorporating named queries into your JPA application can lead to more organized and efficient data access code.
For more in-depth information about JPA named queries, you can refer to the official Oracle documentation.
Start leveraging the benefits of named queries in your JPA applications for enhanced query management and execution.