Mastering Thread Pools for Efficient Stream Processing
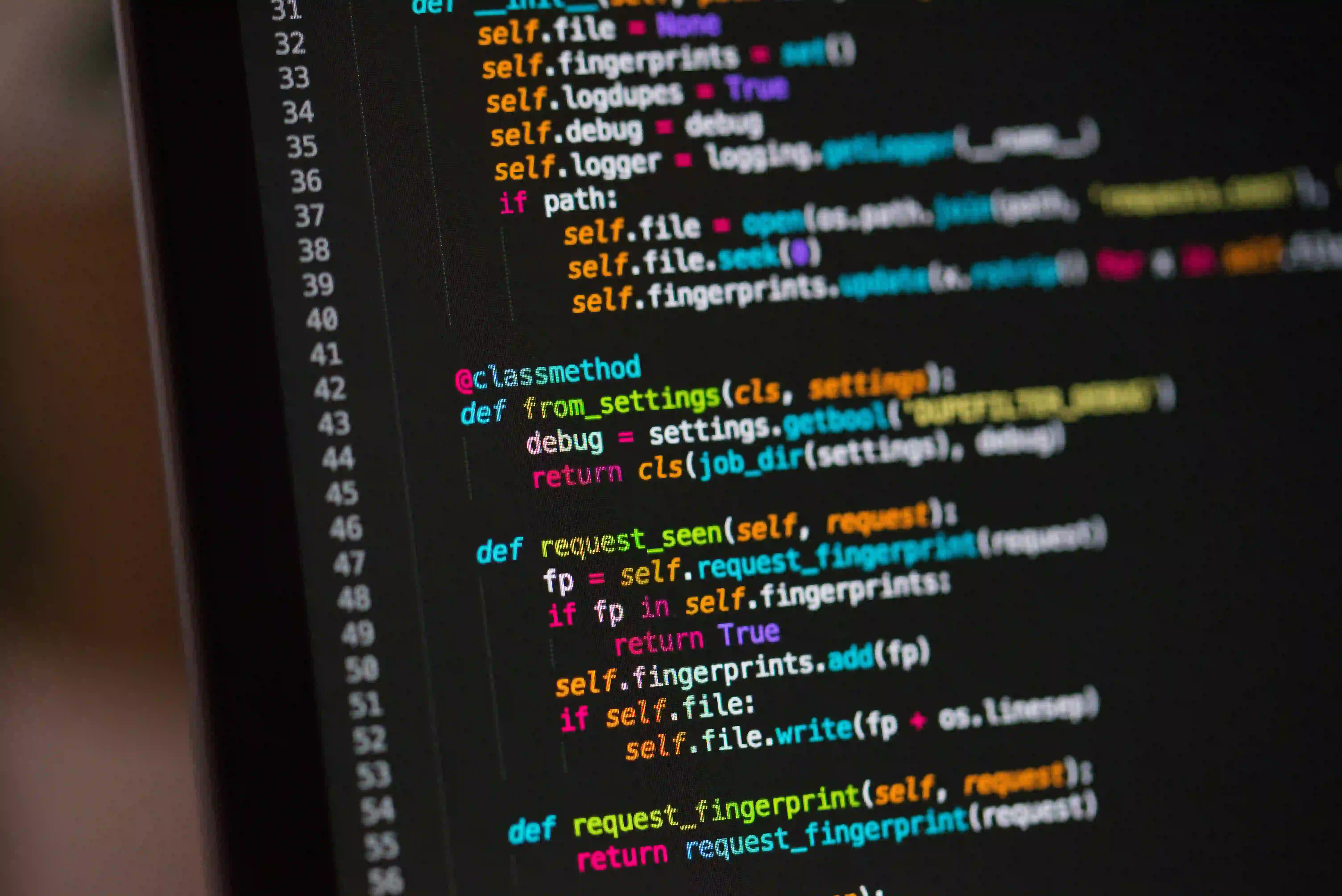
Mastering Thread Pools for Efficient Stream Processing
In modern software development, efficient handling of concurrent tasks is vital, especially when working with data streams. With the rise of multi-core processors, leveraging threading has become essential to maximize performance. In this blog post, we will explore the concept of thread pools in Java, focusing on how they enable efficient stream processing.
Understanding Thread Pools
A thread pool is a collection of pre-initialized threads that can be reused for executing multiple tasks over time, instead of continually creating new threads. This model improves performance by minimizing the overhead associated with thread creation, destruction, and context switching.
Why Use Thread Pools?
- Performance: Creating threads can be expensive. Thread pools mitigate this by reusing existing threads.
- Resource Management: They allow for better control over the number of concurrent threads, preventing potential system resource exhaustion.
- Faster Task Execution: Available threads can quickly pick up new tasks, leading to reduced latency.
- Simplified Thread Management: Using thread pools provides a higher-level abstraction for executing tasks asynchronously.
Implementing Thread Pools in Java
Java provides a robust java.util.concurrent
package that includes the ExecutorService
interface, allowing the easy creation and management of thread pools. Here, we will walk through the practical aspects of implementing thread pools for stream processing.
Creating a Simple Thread Pool
You can create a thread pool using the Executors
utility class. Let’s create a simple thread pool and submit tasks for execution.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class SimpleThreadPoolExample {
public static void main(String[] args) {
// Create a thread pool with a fixed number of threads
ExecutorService executor = Executors.newFixedThreadPool(4);
// Submit tasks to the executor
for (int i = 0; i < 10; i++) {
final int taskId = i;
executor.submit(() -> {
System.out.println("Executing task " + taskId + " by thread " + Thread.currentThread().getName());
// Simulate some work
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
// Shutdown the executor
executor.shutdown();
}
}
Commentary on the Code
In this snippet:
- We create a fixed thread pool with four threads.
- We submit ten tasks to the executor, each simulating a wait time to represent some processing.
- The
executor.shutdown()
method is called to stop accepting new tasks once the submitted tasks finish executing.
The above implementation ensures that all ten tasks are executed using only four threads concurrently, demonstrating the efficiency of thread pools.
Using Thread Pools with Stream API
The Java Stream API provides powerful abstractions for working with collections in a functional style. By integrating thread pools with the Stream API, you can achieve efficient parallel processing.
Parallel Stream Example
Here's how you can process a large data set using a thread pool combined with a parallel stream:
import java.util.List;
import java.util.Arrays;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ParallelStreamThreadPool {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(4);
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
// Process numbers using parallel streams within a thread pool context
numbers.parallelStream().forEach(number -> {
executor.submit(() -> {
System.out.println("Processing number " + number + " by thread " + Thread.currentThread().getName());
// Simulate work
try {
Thread.sleep(500);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
});
executor.shutdown();
}
}
Commentary on Parallel Stream Example
In this example:
- We create a fixed thread pool and initialize a list of integers.
- We utilize a parallel stream to process the numbers.
- Each task is submitted to the executor pool, decoupling the parallel stream processing from the underlying thread management.
Using a parallel stream leverages the thread pool for executing tasks in parallel, which is ideal for CPU-bound operations.
Custom Thread Pool with Callable
Sometimes, you may require a thread pool that returns results. For such tasks, Callable
can be used instead of Runnable
.
Example of Callable with Future
import java.util.List;
import java.util.Arrays;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class CallableExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(4);
List<Future<Integer>> futures;
// Create callable tasks that return results
futures = executor.invokeAll(Arrays.asList(
() -> {
Thread.sleep(1000);
return 1;
},
() -> {
Thread.sleep(1500);
return 2;
},
() -> {
Thread.sleep(2000);
return 3;
}
));
// Collect results
for (Future<Integer> future : futures) {
try {
System.out.println("Result: " + future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
executor.shutdown();
}
}
Commentary on Callable Example
In this code:
- We utilize
Callable
to create tasks that return integer results. - The
execute.invokeAll()
method submits multiple tasks and waits for their completion. - After all tasks complete, we collect the results via
Future.get()
, which blocks until the result is available.
This pattern is particularly useful for batch processing scenarios where results need to be gathered after executing multiple parallel tasks.
In Conclusion, Here is What Matters
Thread pools are a powerful mechanism for efficiently processing streams of data in Java. By leveraging the ExecutorService
interface, developers can create robust applications that maximize resource usage while simplifying concurrency management.
Whether for CPU-bound tasks via parallel streams or for returning results through Callable
, mastering thread pools will significantly enhance your Java programming toolkit.
For further reading, consider exploring the official Java concurrency documentation to gain deeper insights into concurrency handling and advanced thread pool configurations.
Additional Resources
- Java Concurrency Tutorial
- Stream API Documentation
With these principles and techniques at your disposal, you're well on your way to mastering thread pools for efficient stream processing in Java!