Converting Byte Array to InputStream and OutputStream
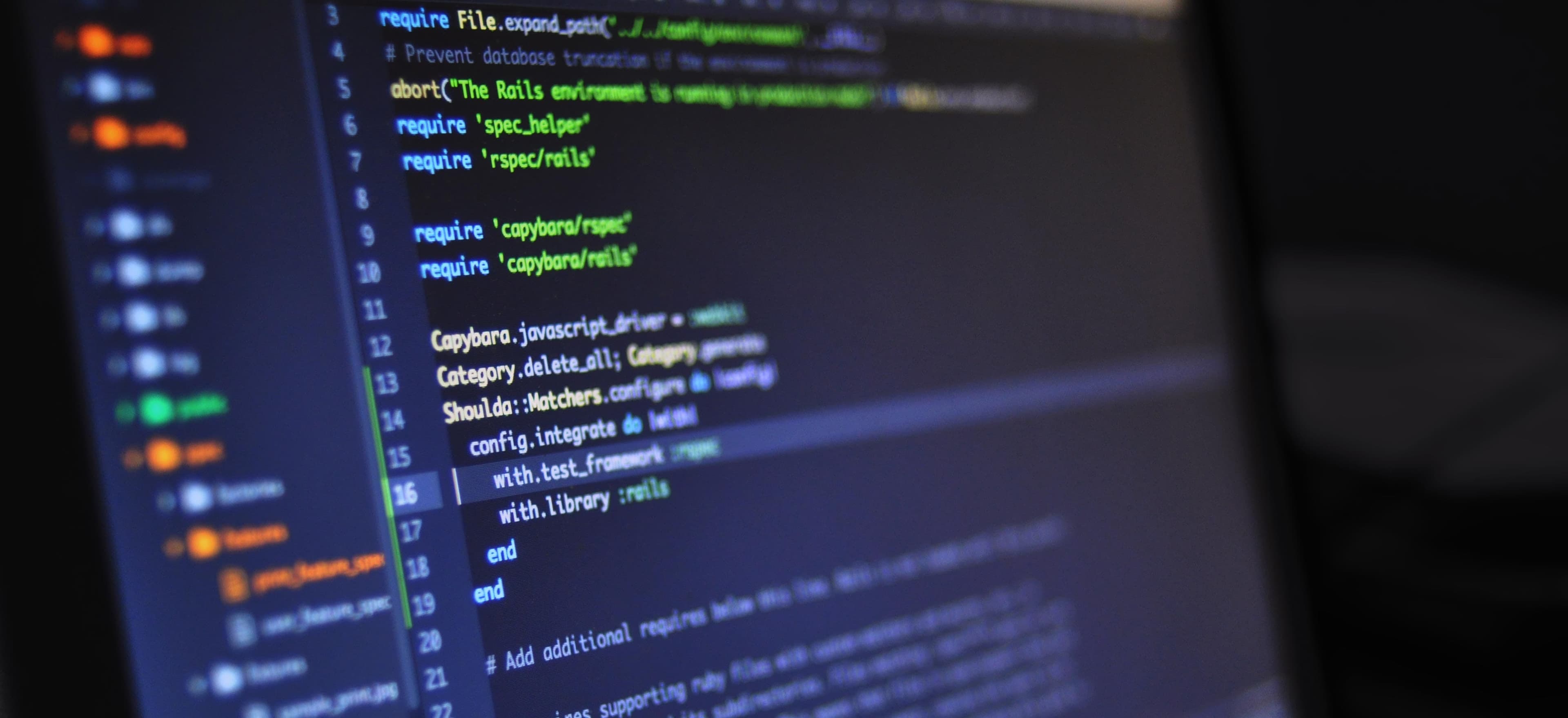
- Published on
In Java, it's common to convert data between different formats. One common task is converting a byte array to an InputStream or OutputStream, and vice versa. This can be particularly useful when working with data in memory or when dealing with I/O operations. In this blog post, we will explore how to perform these conversions in Java, and discuss the scenarios where this can be beneficial.
To start, let's consider the scenario of converting a byte array to an InputStream. This can be useful when you have data in the form of a byte array and need to process it using methods that accept an InputStream as input. One such example is when reading data from a byte array and parsing it using libraries or APIs that operate on InputStreams.
Here's an example of how you can convert a byte array to an InputStream in Java:
byte[] byteArray = // your byte array
InputStream inputStream = new ByteArrayInputStream(byteArray);
In this example, we use the ByteArrayInputStream
class, which extends InputStream
, to create an InputStream from the byte array. This allows us to seamlessly use the data from the byte array as an input stream.
Next, let's consider the opposite scenario - converting an OutputStream to a byte array. This can be beneficial when you want to capture the output of operations performed on an OutputStream into a byte array, for example, when you want to serialize data to a byte array.
Here's an example of how you can achieve this in Java:
OutputStream outputStream = new ByteArrayOutputStream();
// Perform operations on the outputStream
byte[] byteArray = ((ByteArrayOutputStream) outputStream).toByteArray();
In this example, we first create a ByteArrayOutputStream
, which extends OutputStream
, to capture the output of operations performed on the OutputStream. We then use the toByteArray
method to obtain the contents of the ByteArrayOutputStream as a byte array.
Now, let's discuss when these conversions can be particularly useful. When working with external libraries or APIs that expect data in a specific format, such as InputStream, being able to convert data to the expected format can simplify integration and usage. Additionally, in scenarios where you need to process data in memory before writing it to a file or a network stream, these conversions can be very handy.
It's important to note that while these conversions can be helpful, they also come with performance considerations. Converting data between different formats can incur overhead, especially when dealing with large data sets. It's essential to be mindful of such overhead, especially in performance-critical applications.
In conclusion, being able to convert byte arrays to InputStreams and OutputStreams, and vice versa, is a valuable capability in Java. It allows for seamless integration with libraries and APIs, as well as efficient in-memory data processing. However, it's crucial to weigh the benefits against the performance considerations, especially when working with large data sets.
For further reading on InputStream and OutputStream in Java, you can refer to the official Java documentation:
Stay tuned for more Java-related insights and tutorials!