Understanding Static Methods in Scala Objects
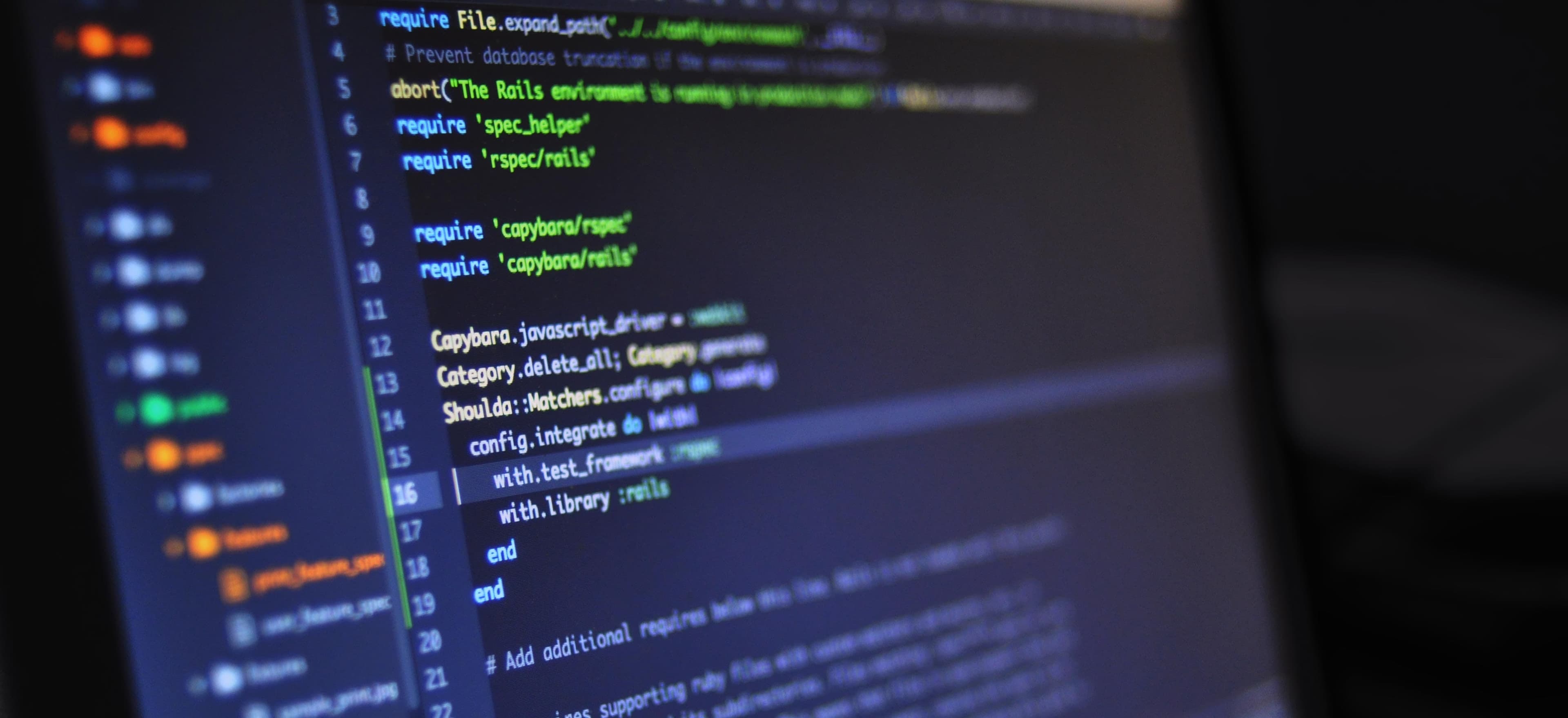
- Published on
An In-depth Look into Static Methods in Scala Objects
Static methods play a crucial role in object-oriented programming, enabling the invocation of functionalities without the need for object instantiation. In Scala, these static methods are commonly implemented within objects, a construct distinctive to the language. Despite their similarity to Java's static methods, Scala objects offer unique capabilities and characteristics that are worth exploring. This blog post delves into the intricacies of static methods within Scala objects, providing readers with a comprehensive understanding of their usage and benefits.
Overview of Scala Objects
In Scala, objects serve as single instances of their own defined types, akin to singletons in other programming languages. They are used to encapsulate methods, variables, and other functionalities, providing a convenient way to organize and access these elements without the need for instantiation. One key feature that distinguishes Scala objects from classes is that they are created implicitly upon their first access, making them readily available for use without the need for manual instantiation.
Declaring Static Methods in Scala Objects
To define a static method within a Scala object, the object
keyword is utilized, followed by the object name and the method's declaration. The static method can then be accessed directly using the object's name, without requiring an instance of the object. Let's consider an example to illustrate this concept:
object MathUtil {
def square(x: Int): Int = {
x * x
}
}
In this example, the MathUtil
object encapsulates a static method named square
, which accepts an integer x
as a parameter and returns its square value. To invoke this static method, the following syntax is employed:
val result = MathUtil.square(5) // Returns 25
Here, the square
method is invoked directly through the MathUtil
object, exemplifying the static nature of the method.
Advantages of Using Static Methods in Scala Objects
The usage of static methods within Scala objects presents several advantages, including:
Accessibility
Static methods can be accessed without the need for object instantiation, providing a convenient and direct approach to invoking functionalities encapsulated within objects.
Organization
By encapsulating related functionalities within a single object, static methods contribute to a well-organized codebase, offering clarity and cohesion in the organization of functionalities.
Memory Efficiency
As static methods do not require object instantiation, they contribute to memory efficiency by minimizing the creation of unnecessary object instances.
Scala Objects vs. Companion Objects
In Scala, the concept of a companion object arises, which is an object sharing the same name as a class and residing in the same source file. This companion object has access to the class's private members and is commonly utilized for creating factory methods, static methods, and other class-level functionalities. By declaring static methods within companion objects, developers can ensure a unified organization of class-related functionalities and leverage the cohesion between the class and its companion object.
Practical Use Cases of Static Methods in Scala Objects
Static methods within Scala objects find application in various scenarios, including:
Utility Functions
Scala objects encapsulate utility functions in the form of static methods, facilitating easy access to common functionalities such as date manipulation, string operations, and mathematical computations.
Factory Methods
Companion objects serve as a common location for defining factory methods, enabling the creation of class instances with specific configurations or initializations.
Final Considerations
In conclusion, static methods within Scala objects provide a convenient and organized approach to encapsulate and access functionalities without object instantiation. By leveraging the intrinsic characteristics of Scala objects and companion objects, developers can architect well-structured and cohesive codebases, fostering reusability and maintainability. The utilization of static methods in Scala objects offers a pragmatic solution to various programming challenges, contributing to the robustness and elegance of Scala applications.
By thoroughly comprehending the nuances and benefits of static methods in Scala objects, developers can harness the full potential of this language feature, strengthening their proficiency in Scala development.
To further explore the topic of Scala objects and static methods, refer to Scala Documentation and Scala School. These resources offer additional insights and practical examples, enriching your understanding and proficiency in Scala programming.